
I messed up the merge, so pushing it over to another repo so I don't lose it. Will tidy up and remove later
Dependencies: BufferedSerial FatFileSystemCpp mbed
LTCDecode.cpp
00001 #include "LTCApp.h" 00002 00003 00004 const uint32_t minBitPeriodUS = 00005 (uint32_t)((1000000.0f / 2400) / 1.1f); // allow for 10% time error 00006 const uint32_t maxMidSymbolTimeUS = (uint32_t)( 00007 ((1000000.0f / (1920 * 2))) * 1.1f); // allow for 10% time error 00008 00009 00010 LTCDecode::LTCDecode(const PinName pin) : _LTCIn(pin) 00011 { 00012 LTCsynced = false; 00013 inputTimer.reset(); 00014 inputTimer.start(); 00015 FrameCallback = NULL; 00016 } 00017 00018 void LTCDecode::enable(bool enable) 00019 { 00020 LTCsynced = false; 00021 if (enable) { 00022 inputTimer.start(); 00023 inputTimer.reset(); 00024 _LTCIn.fall(callback(this, <CDecode::LTCOnEdge)); 00025 _LTCIn.rise(callback(this, <CDecode::LTCOnEdge)); 00026 } else { 00027 inputTimer.stop(); 00028 _LTCIn.fall(NULL); 00029 _LTCIn.rise(NULL); 00030 } 00031 } 00032 00033 void LTCDecode::LTCOnEdge() 00034 { 00035 uint32_t transitionTime = inputTimer.read_us(); 00036 uint32_t period = (transitionTime - lastTransition); 00037 lastTransition = transitionTime; 00038 bool gotSync = false; 00039 00040 if (period > maxMidSymbolTimeUS) { // time since last transition is > maximum time for 00041 // a 1, must be a zero and start of the next bit. 00042 lastBitStart = transitionTime; 00043 addBitToBuffer(false); 00044 return;// can't be an end of frame, they must end on a 1 00045 } 00046 00047 // if it's not a 0 it must be a 1 but are we at the end of the bit yet? 00048 if ((transitionTime - lastBitStart)> minBitPeriodUS) { // end of a bit 00049 lastBitStart = transitionTime; 00050 gotSync = addBitToBuffer(true); 00051 if (gotSync) { 00052 LTCsynced = true; 00053 decodeTime(); 00054 if (FrameCallback) 00055 FrameCallback.call(); 00056 } 00057 } 00058 } 00059 00060 // add a bit. Return true if we got the end of frame pattern 00061 bool LTCDecode::addBitToBuffer(bool bit) 00062 { 00063 LTCBuffer[0] = LTCBuffer[0]>>1; 00064 if (LTCBuffer[1] & 0x01) 00065 LTCBuffer[0] |= 0x80000000; 00066 00067 LTCBuffer[1] = LTCBuffer[1]>>1; 00068 if (LTCBuffer[2] & 0x01) 00069 LTCBuffer[1] |= 0x80000000; 00070 00071 LTCBuffer[2] = LTCBuffer[2]>>1; 00072 if (bit) 00073 LTCBuffer[2] |= 0x80000000; 00074 00075 // frame sync patterin is 1011 1111 1111 1100 00076 // if it's a valid sync we will have it just received (buffer2 top bits) and the one from the last frame still in the buffer as the low 16 bits of buffer 0. 00077 return ((((LTCBuffer[2]>>16) & 0x0000FFFF) == 0xBFFC) && ((LTCBuffer[0] & 0x0000FFFF) == 0xBFFC)); 00078 } 00079 00080 void LTCDecode::decodeTime() 00081 { 00082 _frame = ((LTCBuffer[0]>>16) & 0x0f) + ((LTCBuffer[0] >> 24) & 0x03) * 10; 00083 _seconds = ((LTCBuffer[1]>>0) & 0x0f) + ((LTCBuffer[1] >> 8) & 0x07) * 10; 00084 _minutes = ((LTCBuffer[1]>>16) & 0x0f) + ((LTCBuffer[1] >> 24) & 0x07) * 10; 00085 _hours = ((LTCBuffer[2]>>0) & 0x0f) + ((LTCBuffer[2] >> 8) & 0x03) * 10; 00086 _frameDrop = (LTCBuffer[0] & (1<<26)); 00087 }
Generated on Thu Dec 15 2022 06:07:04 by
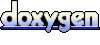