
I messed up the merge, so pushing it over to another repo so I don't lose it. Will tidy up and remove later
Dependencies: BufferedSerial FatFileSystemCpp mbed
LTCApp.h
00001 #ifndef __LTCApp_h__ 00002 #define __LTCApp_h__ 00003 00004 #include "mbed.h" 00005 #include "LTCDecode.h" 00006 #include "VIPSSerialProtocol.h" 00007 #include "frameRates.h" 00008 #include "BufferedSerial.h" 00009 #include "FIZ_DISNEY.h" 00010 #include "FIZ_digiPower.h" 00011 #include "FIZDigiPowerActive.h" 00012 #include "FIZCanon.h" 00013 #include "FIZ_ArriCmotion.h" 00014 #include "frameclock.h" 00015 #include "FreeD.h" 00016 #include <stdint.h> 00017 #include <vector> 00018 00019 extern volatile uint32_t VBOXTicks; 00020 extern volatile bool ppsActive; 00021 00022 extern BufferedSerial pc; 00023 00024 extern frameRates detectedRate; 00025 00026 extern Timer TimeSinceLastFrame; 00027 extern uint32_t TimeSinceLastFrameWrap; 00028 extern DigitalOut led1; 00029 00030 extern void vipsBypassRx(char byte); 00031 00032 typedef struct UserSettings_s { 00033 int FIZmode; 00034 int SerialOutMode; 00035 int FreeDPort; 00036 int DataOutPort; 00037 int VipsUDPPort; 00038 int SettingsPort; 00039 char IPAddress[32]; 00040 char Gateway[32]; 00041 char Subnet[32]; 00042 int FilterOrder; 00043 float FilterFreq; 00044 float FilterRate; 00045 bool FilterXY; 00046 bool FilterZ; 00047 bool FilterRoll; 00048 bool FilterPitch; 00049 bool FilterYaw; 00050 bool AutoHyperSmooth; 00051 bool FlexibleVIPSOut; 00052 float SerialTxDelayMS; 00053 float UDPTxDelayMS; 00054 float SerialTxDelayFrame; 00055 float UDPTxDelayFrame; 00056 float InterpolationOffset_uS; 00057 float InterpolationOffsetFrame; 00058 bool InvertRoll; 00059 bool InvertPitch; 00060 bool InvertYaw; 00061 int OffsetRoll; 00062 int OffsetPitch; 00063 int OffsetYaw; 00064 int bypassBaud; 00065 bool ForcePPF; 00066 bool HalfRate; 00067 float focus_scale; 00068 float focus_offset; 00069 float iris_scale; 00070 float iris_offset; 00071 float zoom_scale; 00072 float zoom_offset; 00073 bool low_zoom_precision; 00074 bool absolute_focus; 00075 bool absolute_iris; 00076 bool absolute_zoom; 00077 vector<unsigned int> focus_encoder_map; 00078 vector<unsigned int> focus_absolute_map; 00079 vector<unsigned int> iris_encoder_map; 00080 vector<unsigned int> iris_absolute_map; 00081 vector<unsigned int> zoom_encoder_map; 00082 vector<unsigned int> zoom_absolute_map; 00083 } UserSettings_t; 00084 00085 extern UserSettings_t UserSettings; 00086 00087 // extern int pos_upper; 00088 // extern int pos_lower; 00089 // extern int pos_value; 00090 00091 #endif
Generated on Thu Dec 15 2022 06:07:04 by
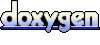