
I messed up the merge, so pushing it over to another repo so I don't lose it. Will tidy up and remove later
Dependencies: BufferedSerial FatFileSystemCpp mbed
FIZ_DISNEY.cpp
00001 #include "FIZ_DISNEY.h" 00002 #include "LTCApp.h" 00003 FIZDisney::FIZDisney(const PinName Tx, const PinName Rx) : FIZReader(Tx,Rx) 00004 { 00005 inputPtr = 0; 00006 newData = false; 00007 _port.baud(115200); 00008 _port.attach(callback(this, &FIZDisney::OnRx)); 00009 } 00010 00011 void FIZDisney::requestCurrent(void) 00012 { 00013 _port.putc(0x02); 00014 _port.putc('0'); 00015 _port.putc('C'); 00016 _port.putc('0'); 00017 _port.putc('0'); 00018 _port.putc('D'); 00019 _port.putc('5'); 00020 _port.putc(0x03); 00021 } 00022 00023 void FIZDisney::OnRx(void) 00024 { 00025 inputBuffer[inputPtr] = _port.getc(); 00026 if (inputPtr==0) { 00027 if (inputBuffer[inputPtr] == 0x02) 00028 inputPtr++; 00029 } else if (inputBuffer[inputPtr] == 0x03) { 00030 parsePacket(); 00031 inputPtr = 0; 00032 } else { 00033 inputPtr++; 00034 if (inputPtr == InBufferSize) 00035 inputPtr = 0; 00036 } 00037 } 00038 00039 void FIZDisney::parsePacket() 00040 { 00041 // expect ASCII string of "0C07ffffffiiiizzzzcs" where ffffff is 6 char hex value for focus 00042 // iiii is 4 char hex value for tstop, zzzz is 4 char hex value for zoom and cs is 2 char checksum 00043 if (inputPtr != 21) 00044 return; 00045 if (inputBuffer[1] != '0') 00046 return; 00047 if (inputBuffer[2] != 'C') 00048 return; 00049 _focus = 0; 00050 _iris = 0; 00051 _zoom = 0; 00052 for (int count = 0; count<6; count++) { 00053 _focus*=16; 00054 _focus += hexValue(inputBuffer[5+count]); 00055 } 00056 for (int count = 0; count<4; count++) { 00057 _iris*=16; 00058 _iris += hexValue(inputBuffer[11+count]); 00059 _zoom*=16; 00060 _zoom += hexValue(inputBuffer[15+count]); 00061 } 00062 // raw iris data is in 10ths of a stop. We want 100ths 00063 _iris *= 10; 00064 newData = true; 00065 }
Generated on Thu Dec 15 2022 06:07:04 by
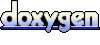