
I messed up the merge, so pushing it over to another repo so I don't lose it. Will tidy up and remove later
Dependencies: BufferedSerial FatFileSystemCpp mbed
FIZCanon.h
00001 #ifndef __FIZCanon_H__ 00002 #define __FIZCanon_H__ 00003 #include "FIZReader.h" 00004 00005 //FIZ protocol used for Canon Lens. 00006 00007 class FIZCanon : public FIZReader 00008 { 00009 00010 public: 00011 FIZCanon(const PinName Tx, const PinName Rx); 00012 virtual void requestCurrent(); 00013 00014 private: 00015 static const int InBufferSize = 32; 00016 00017 void OnRx(void); 00018 void parsePacket(); 00019 00020 int missedPackets; 00021 uint8_t inputBuffer[InBufferSize]; 00022 int inputPtr; 00023 }; 00024 00025 #define CANON_ZOOM 0xC1 00026 #define CANON_FOCUS 0xC3 00027 #define CANON_IRIS 0xC5 00028 #define CANON_START 0x94 00029 00030 00031 #endif
Generated on Thu Dec 15 2022 06:07:04 by
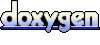