
display i2c
Dependencies: mbed SSD1308_128x64_I2C USBDevice
main.cpp
00001 /* mbed Seeed 128x64 OLED Test 00002 * http://www.seeedstudio.com/depot/grove-oled-display-12864-p-781.html?cPath=163_167 00003 * 00004 * Copyright (c) 2012 Wim Huiskamp 00005 * Released under the MIT License: http://mbed.org/license/mit 00006 * 00007 * version 0.2 Initial Release 00008 * version 0.3 2017 Fixed non-copyable issue (Thx kenjiArai) 00009 */ 00010 #include "mbed.h" 00011 #include "mbed_logo.h" 00012 #include "SSD1308.h" 00013 #include "USBSerial.h" 00014 00015 // Host PC Communication channels 00016 USBSerial pc; 00017 00018 #define D_SDA PB_9 00019 #define D_SCL PB_8 00020 00021 00022 I2C i2c(D_SDA, D_SCL); 00023 00024 //Host PC Baudrate (Virtual Com Port on USB) 00025 //#define D_BAUDRATE 9600 00026 //#define D_BAUDRATE 57600 00027 00028 // mbed Interface Hardware definitions 00029 DigitalOut myled1(LED1); 00030 DigitalOut myled2(LED2); 00031 DigitalOut myled3(LED3); 00032 DigitalOut heartbeatLED(LED4); 00033 00034 00035 // Instantiate OLED 00036 SSD1308 oled = SSD1308(&i2c, SSD1308_SA0); 00037 00038 void show_menu() { 00039 pc.printf("0: Exit\r"); 00040 pc.printf("1: Show Menu\r"); 00041 pc.printf("2: Send Message\r"); 00042 pc.printf("3: Fill Display\r"); 00043 pc.printf("4: Display cleared\r"); 00044 pc.printf("5: Display off\r"); 00045 pc.printf("6: Display on\r"); 00046 pc.printf("7: Display Invert\r"); 00047 pc.printf("8: Display Normal\r"); 00048 pc.printf("9: Display Bitmap\r"); 00049 pc.printf("A: Brightness Ramp\r"); 00050 pc.printf("B: Send Inverted Message\r"); 00051 pc.printf("C: Flip and Mirror\r"); 00052 pc.printf("D: Blink\r"); 00053 pc.printf("E: Big Chars\r"); 00054 pc.printf("F: Progress Scale\r"); 00055 pc.printf("G: Scroll\r"); 00056 pc.printf("H: Hor Scroll\r"); 00057 pc.printf("V: Ver and Hor Scroll\r"); 00058 // pc.printf("\n\r"); 00059 00060 } 00061 00062 00063 void oled_Test() { 00064 pc.printf("OLED test start\r"); 00065 00066 00067 pc.printf("Hello World from ST32F401RE\n\r"); 00068 00069 00070 // oled.writeString(0, 0, 13, "Hello World !"); 00071 oled.writeString(0, 0, "Hello World !"); 00072 pc.printf("Printed something\r"); 00073 wait(3); 00074 00075 // oled.writeString(1, 0, 8, "baz quux"); 00076 oled.writeString(1, 0, "baz quux"); 00077 pc.printf("Printed something\r"); 00078 wait(3); 00079 00080 // oled.writeString(4, 0, 272, "a long, rather lengthy, extended string passage thing, eh, that just goes on, and on, and on, and on, and on, and on, and on, yes, further, continuing, extending, expanding beyond all reason or sanity!!!!! and yet, there's more! so much more! for ever and ever, oh yeah"); 00081 // pc.printf("Printed something\r"); 00082 // wait(3); 00083 00084 oled.fillDisplay(0xAA); 00085 pc.printf("Display filled\r"); 00086 wait(3); 00087 00088 oled.setDisplayOff(); 00089 pc.printf("Display off\r"); 00090 wait(0.5); 00091 00092 oled.setDisplayOn(); 00093 pc.printf("Display on\r"); 00094 wait(0.5); 00095 00096 oled.clearDisplay(); 00097 pc.printf("Display cleared\r"); 00098 wait(0.5); 00099 00100 // oled.writeString(0, 0, 11, "Bye World !"); 00101 oled.writeString(0, 0, "Bye World !"); 00102 pc.printf("Printed something\r"); 00103 wait(3); 00104 00105 pc.printf("OLED test done\r"); 00106 } 00107 00108 // Variables for Heartbeat and Status monitoring 00109 Ticker heartbeat; 00110 bool heartbeatflag=false; 00111 00112 // Local functions 00113 void clear_screen() { 00114 //ANSI Terminal Commands 00115 pc.printf("\x1B[2J"); 00116 pc.printf("\x1B[H"); 00117 } 00118 00119 00120 void init_interfaces() { 00121 i2c.frequency(400000); // according to the spec the max bitrate for the SSD1308 is 400 kbit/s 00122 } 00123 00124 00125 // Heartbeat monitor 00126 void pulse() { 00127 heartbeatLED = !heartbeatLED; 00128 } 00129 00130 void heartbeat_start() { 00131 heartbeat.attach(&pulse, 0.5); 00132 } 00133 00134 void heartbeat_stop() { 00135 heartbeat.detach(); 00136 } 00137 00138 00139 int main() { 00140 bool running=true; 00141 bool left = true; 00142 bool down = true; 00143 00144 char command; 00145 00146 init_interfaces(); 00147 00148 heartbeat_start(); 00149 00150 clear_screen(); 00151 00152 pc.printf("Hello World!\r"); 00153 00154 #if(0) 00155 // Quick test 00156 oled_Test(); 00157 00158 while(1) { 00159 myled1 = 1; 00160 wait(0.2); 00161 00162 myled1 = 0; 00163 wait(0.2); 00164 pc.printf("*"); 00165 } 00166 #else 00167 // Interactive Test 00168 show_menu(); 00169 00170 while(running) { 00171 00172 if(pc.readable()) { 00173 command = pc.getc(); 00174 //pc.printf("command= %c \n\r", command); 00175 pc.printf("\r"); 00176 00177 switch (command) { 00178 case '0' : 00179 pc.printf("Done\r"); 00180 running = false; 00181 break; 00182 00183 case '1' : 00184 show_menu(); 00185 break; 00186 00187 case '2' : 00188 pc.printf("Hello World!\r"); 00189 oled.writeString(0, 0, "Hello World !"); 00190 break; 00191 00192 case '3' : 00193 pc.printf("Fill part of Display 0xA5\r"); 00194 // oled.fillDisplay(0xA5); 00195 00196 oled.fillDisplay(0xA5, 2, 5, 0, 63); 00197 break; 00198 00199 case '4' : 00200 pc.printf("Display cleared\r"); 00201 oled.clearDisplay(); 00202 break; 00203 00204 case '5' : 00205 pc.printf("Display off\r"); 00206 oled.setDisplayOff(); 00207 break; 00208 case '6' : 00209 pc.printf("Display on\r"); 00210 oled.setDisplayOn(); 00211 break; 00212 00213 case '7' : 00214 pc.printf("Display Invert\r"); 00215 oled.setDisplayInverse(); 00216 break; 00217 00218 case '8' : 00219 pc.printf("Display Normal\r"); 00220 oled.setDisplayNormal(); 00221 break; 00222 00223 case '9' : 00224 pc.printf("Display bitmap\r"); 00225 oled.writeBitmap((uint8_t*) mbed_logo); 00226 00227 break; 00228 00229 case 'A' : 00230 pc.printf("Brightness Ramp Down\r"); 00231 for (int contrast=0x7F; contrast >= 0x10; contrast--) { 00232 oled.setContrastControl(contrast); 00233 wait(0.05); 00234 } 00235 00236 wait(1); 00237 00238 pc.printf("Brightness Ramp Up\r"); 00239 for (int contrast=0x10; contrast <= 0x7F; contrast++) { 00240 oled.setContrastControl(contrast); 00241 wait(0.05); 00242 } 00243 00244 pc.printf("Brightness Ramp Done\r"); 00245 00246 break; 00247 00248 case 'B' : 00249 pc.printf("Send Inverted Message\r"); 00250 oled.setInverted(true); 00251 // oled.writeString(0, 0, 13, "Hello World !"); 00252 oled.writeString(0, 0, "Hello World !"); 00253 oled.setInverted(false); 00254 00255 oled.printf(" Result is %d", 12345); 00256 00257 break; 00258 00259 case 'C' : 00260 pc.printf("Flip and Mirror (Rewrite display to show horizontal effect)\r"); 00261 left = !left; 00262 down = !down; 00263 oled.setDisplayFlip(left, down); 00264 break; 00265 00266 case 'D' : 00267 pc.printf("D: Blink and Fade (not supported)\r"); 00268 00269 // oled.setDisplayBlink(true); 00270 // wait(4); 00271 // oled.setDisplayBlink(false); 00272 00273 oled.setDisplayFade(true); 00274 wait(4); 00275 oled.setDisplayFade(false); 00276 00277 pc.printf("D: Blink done\r"); 00278 break; 00279 00280 case 'E' : 00281 pc.printf("E: Big Chars\r"); 00282 00283 oled.writeBigChar(0, 0, '+'); 00284 oled.writeBigChar(0, 16, '7'); 00285 oled.writeBigChar(0, 32, '8'); 00286 oled.writeBigChar(0, 48, '9'); 00287 00288 pc.printf("E: Big Chars done\r"); 00289 break; 00290 00291 case 'F' : 00292 pc.printf("F: Progress Scale\r"); 00293 00294 for (int percentage=0; percentage <= 100; percentage++) { 00295 oled.writeProgressBar(2, 0, percentage); 00296 oled.printf(" %3d%%", percentage); 00297 00298 oled.writeProgressBar(4, 0, 100 - percentage); 00299 oled.printf(" %3d%%", 100 - percentage); 00300 00301 oled.writeLevelBar(6, 0, percentage); 00302 oled.printf(" %3dmV", percentage); 00303 00304 wait(0.05); 00305 } 00306 00307 pc.printf("F: Progress Scale done\r"); 00308 break; 00309 00310 case 'G' : 00311 pc.printf("G: Scroll\r"); 00312 00313 for (int line=0; line < ROWS; line++) { 00314 oled.setDisplayStartLine(line); 00315 wait(0.05); 00316 } 00317 00318 oled.setDisplayStartLine(0); 00319 00320 pc.printf("G: Scroll done\r"); 00321 break; 00322 00323 00324 case 'H' : 00325 // pc.printf("H: Hor Scroll\r"); 00326 pc.printf("H: Hor Scroll (Page 0-3)\r"); 00327 // oled.setContinuousHorizontalScroll(true, PAGE0, PAGE7, SCROLL_INTERVAL_25_FRAMES); 00328 oled.setContinuousHorizontalScroll(true, PAGE0, PAGE3, SCROLL_INTERVAL_25_FRAMES); 00329 oled.setDisplayScroll(true); 00330 00331 wait(5); 00332 00333 oled.setDisplayScroll(false); 00334 00335 pc.printf("H: Hor Scroll done\r"); 00336 break; 00337 00338 00339 case 'V' : 00340 pc.printf("V: Ver and Hor Scroll (Page 0-7)\r"); 00341 00342 00343 oled.setContinuousVerticalAndHorizontalScroll(true, PAGE0, PAGE7, 0x01, SCROLL_INTERVAL_25_FRAMES); 00344 oled.setVerticalScrollArea(20, 20); 00345 00346 oled.setDisplayScroll(true); 00347 00348 00349 wait(5); 00350 00351 oled.setDisplayScroll(false); 00352 00353 pc.printf("V: Ver and Hor Scroll done\r"); 00354 break; 00355 00356 00357 } //switch 00358 }//if 00359 }//while 00360 #endif 00361 00362 pc.printf("Bye World!\n\r"); 00363 }//main 00364
Generated on Thu Jul 21 2022 03:41:05 by
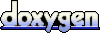