
This library enables users to communicate with the ADXL345 accelerometer through the I2C bus on the mbed. The API names are similar and work nearly the same way as those made in the SPI libraries for the ADXL345.
Dependencies: mbed
ADXL345_I2C.cpp
00001 /** 00002 * @author Peter Swanson 00003 * A personal note from me: Jesus Christ has changed my life so much it blows my mind. I say this because 00004 * today, religion is thought of as something that you do or believe and has about as 00005 * little impact on a person as their political stance. But for me, God gives me daily 00006 * strength and has filled my life with the satisfaction that I could never find in any 00007 * of the other things that I once looked for it in. 00008 * If your interested, heres verse that changed my life: 00009 * Rom 8:1-3: "Therefore, there is now no condemnation for those who are in Christ Jesus, 00010 * because through Christ Jesus, the law of the Spirit who gives life has set 00011 * me free from the law of sin (which brings...) and death. For what the law 00012 * was powerless to do in that it was weakened by the flesh, God did by sending 00013 * His own Son in the likeness of sinful flesh to be a sin offering. And so He 00014 * condemned sin in the flesh in order that the righteous requirements of the 00015 * (God's) law might be fully met in us, who live not according to the flesh 00016 * but according to the Spirit." 00017 * 00018 * A special thanks to Ewout van Bekkum for all his patient help in developing this library! 00019 * 00020 * @section LICENSE 00021 * 00022 * Permission is hereby granted, free of charge, to any person obtaining a copy 00023 * of this software and associated documentation files (the "Software"), to deal 00024 * in the Software without restriction, including without limitation the rights 00025 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00026 * copies of the Software, and to permit persons to whom the Software is 00027 * furnished to do so, subject to the following conditions: 00028 * 00029 * The above copyright notice and this permission notice shall be included in 00030 * all copies or substantial portions of the Software. 00031 * 00032 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00033 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00034 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00035 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00036 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00037 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00038 * THE SOFTWARE. 00039 * 00040 * @section DESCRIPTION 00041 * 00042 * ADXL345, triple axis, I2C interface, accelerometer. 00043 * 00044 * Datasheet: 00045 * 00046 * http://www.analog.com/static/imported-files/data_sheets/ADXL345.pdf 00047 */ 00048 00049 /** 00050 * Includes 00051 */ 00052 #include "ADXL345_I2C.h" 00053 00054 //#include "mbed.h" 00055 00056 ADXL345_I2C::ADXL345_I2C(PinName sda, PinName scl) : i2c_(sda, scl) { 00057 00058 //400kHz, allowing us to use the fastest data rates. 00059 i2c_.frequency(100000); 00060 // initialize the BW data rate 00061 char tx[2]; 00062 tx[0] = ADXL345_BW_RATE_REG; 00063 tx[1] = ADXL345_100HZ; //value greater than or equal to 0x0A is written into the rate bits (Bit D3 through Bit D0) in the BW_RATE register 00064 i2c_.write( ADXL345_I2C_WRITE , tx, 2); 00065 //Data format (for +-16g) - This is done by setting Bit D3 of the DATA_FORMAT register (Address 0x31) and writing a value of 0x03 to the range bits (Bit D1 and Bit D0) of the DATA_FORMAT register (Address 0x31). 00066 00067 char rx[2]; 00068 rx[0] = ADXL345_DATA_FORMAT_REG; 00069 rx[1] = 0x0B; 00070 // full res and +_16g 00071 i2c_.write( ADXL345_I2C_WRITE , rx, 2); 00072 00073 // Set Offset - programmed into the OFSX, OFSY, and OFXZ registers, respectively, as 0xFD, 0x03 and 0xFE. 00074 char x[2]; 00075 x[0] = ADXL345_OFSX_REG ; 00076 x[1] = 0xFD; 00077 i2c_.write( ADXL345_I2C_WRITE , x, 2); 00078 char y[2]; 00079 y[0] = ADXL345_OFSY_REG ; 00080 y[1] = 0x03; 00081 i2c_.write( ADXL345_I2C_WRITE , y, 2); 00082 char z[2]; 00083 z[0] = ADXL345_OFSZ_REG ; 00084 z[1] = 0xFE; 00085 i2c_.write( ADXL345_I2C_WRITE , z, 2); 00086 00087 // LCD init 00088 char init[7] = {LCD_CMD, LCD_CLEAR, LCD_CMD, LCD_CURSOR, 0, LCD_CMD, LCD_ON}; 00089 i2c_.write(LCD, init, 7); 00090 } 00091 00092 00093 char ADXL345_I2C::SingleByteRead(char address){ 00094 char tx = address; 00095 char output; 00096 i2c_.write( ADXL345_I2C_WRITE , &tx, 1); //tell it what you want to read 00097 i2c_.read( ADXL345_I2C_READ , &output, 1); //tell it where to store the data 00098 return output; 00099 00100 } 00101 00102 00103 /* 00104 ***info on the i2c_.write*** 00105 address 8-bit I2C slave address [ addr | 0 ] 00106 data Pointer to the byte-array data to send 00107 length Number of bytes to send 00108 repeated Repeated start, true - do not send stop at end 00109 returns 0 on success (ack), or non-0 on failure (nack) 00110 */ 00111 00112 int ADXL345_I2C::SingleByteWrite(char address, char data){ 00113 int ack = 0; 00114 char tx[2]; 00115 tx[0] = address; 00116 tx[1] = data; 00117 return ack | i2c_.write( ADXL345_I2C_WRITE , tx, 2); 00118 } 00119 00120 00121 00122 void ADXL345_I2C::multiByteRead(char address, char* output, int size) { 00123 i2c_.write( ADXL345_I2C_WRITE, &address, 1); //tell it where to read from 00124 i2c_.read( ADXL345_I2C_READ , output, size); //tell it where to store the data read 00125 } 00126 00127 00128 int ADXL345_I2C::multiByteWrite(char address, char* ptr_data, int size) { 00129 int ack; 00130 00131 ack = i2c_.write( ADXL345_I2C_WRITE, &address, 1); //tell it where to write to 00132 return ack | i2c_.write( ADXL345_I2C_READ, ptr_data, size); //tell it what data to write 00133 00134 } 00135 00136 00137 void ADXL345_I2C::getOutput(int* readings){ 00138 char buffer[6]; 00139 multiByteRead(ADXL345_DATAX0_REG, buffer, 6); 00140 00141 readings[0] = (int)buffer[1] << 8 | (int)buffer[0]; 00142 readings[1] = (int)buffer[3] << 8 | (int)buffer[2]; 00143 readings[2] = (int)buffer[5] << 8 | (int)buffer[4]; 00144 00145 } 00146 00147 00148 void ADXL345_I2C::writeEEPROM(char *data, uint8_t lenght){ 00149 i2c_.write(EEPROM_WRITE, data, lenght, 0); 00150 } 00151 00152 00153 void ADXL345_I2C::LCDprint(float Xaxes = 0, float Yaxes = 0, float Zaxes = 0){ 00154 00155 // X 00156 stringstream ss; 00157 ss << Xaxes; 00158 string x = "X : " + ss.str(); 00159 uint8_t length = x.size(); 00160 char data[length + 2]; 00161 data[0] = LCD_CURSOR; 00162 data[1] = 0; 00163 for(uint8_t pop = 0; pop < length; pop++){ 00164 data[pop + 2] = x[pop]; 00165 } 00166 i2c_.write(LCD, data, length); 00167 00168 // Y 00169 ss << Yaxes; 00170 string y = "Y : " + ss.str(); 00171 length = y.size(); 00172 data[length + 2]; 00173 data[0] = LCD_CURSOR; 00174 data[1] = 0x40; 00175 for(uint8_t pop = 0; pop < length; pop++){ 00176 data[pop + 2] = y[pop]; 00177 } 00178 i2c_.write(LCD, data, length); 00179 00180 // Z 00181 ss << Zaxes; 00182 string z = "z : " + ss.str(); 00183 length = z.size(); 00184 data[length + 2]; 00185 data[0] = LCD_CURSOR; 00186 data[1] = 0x14; 00187 for(uint8_t pop = 0; pop < length; pop++){ 00188 data[pop + 2] = z[pop]; 00189 } 00190 i2c_.write(LCD, data, length); 00191 } 00192 00193 00194 char ADXL345_I2C::getDeviceID() { 00195 return SingleByteRead(ADXL345_DEVID_REG); 00196 } 00197 // 00198 int ADXL345_I2C::setPowerMode(char mode) { 00199 00200 //Get the current register contents, so we don't clobber the rate value. 00201 char registerContents = (mode << 4) | SingleByteRead(ADXL345_BW_RATE_REG); 00202 00203 return SingleByteWrite(ADXL345_BW_RATE_REG, registerContents); 00204 00205 } 00206 00207 char ADXL345_I2C::getPowerControl() { 00208 return SingleByteRead(ADXL345_POWER_CTL_REG); 00209 } 00210 00211 int ADXL345_I2C::setPowerControl(char settings) { 00212 return SingleByteWrite(ADXL345_POWER_CTL_REG, settings); 00213 00214 } 00215 00216 00217 00218 char ADXL345_I2C::getDataFormatControl(void){ 00219 00220 return SingleByteRead(ADXL345_DATA_FORMAT_REG); 00221 } 00222 00223 int ADXL345_I2C::setDataFormatControl(char settings){ 00224 00225 return SingleByteWrite(ADXL345_DATA_FORMAT_REG, settings); 00226 00227 } 00228 00229 int ADXL345_I2C::setDataRate(char rate) { 00230 00231 //Get the current register contents, so we don't clobber the power bit. 00232 char registerContents = SingleByteRead(ADXL345_BW_RATE_REG); 00233 00234 registerContents &= 0x10; 00235 registerContents |= rate; 00236 00237 return SingleByteWrite(ADXL345_BW_RATE_REG, registerContents); 00238 00239 } 00240 00241 00242 char ADXL345_I2C::getOffset(char axis) { 00243 00244 char address = 0; 00245 00246 if (axis == ADXL345_X) { 00247 address = ADXL345_OFSX_REG; 00248 } else if (axis == ADXL345_Y) { 00249 address = ADXL345_OFSY_REG; 00250 } else if (axis == ADXL345_Z) { 00251 address = ADXL345_OFSZ_REG; 00252 } 00253 00254 return SingleByteRead(address); 00255 } 00256 00257 int ADXL345_I2C::setOffset(char axis, char offset) { 00258 00259 char address = 0; 00260 00261 if (axis == ADXL345_X) { 00262 address = ADXL345_OFSX_REG; 00263 } else if (axis == ADXL345_Y) { 00264 address = ADXL345_OFSY_REG; 00265 } else if (axis == ADXL345_Z) { 00266 address = ADXL345_OFSZ_REG; 00267 } 00268 00269 return SingleByteWrite(address, offset); 00270 00271 } 00272 00273 00274 char ADXL345_I2C::getFifoControl(void){ 00275 00276 return SingleByteRead(ADXL345_FIFO_CTL); 00277 00278 } 00279 00280 int ADXL345_I2C::setFifoControl(char settings){ 00281 return SingleByteWrite(ADXL345_FIFO_STATUS, settings); 00282 00283 } 00284 00285 char ADXL345_I2C::getFifoStatus(void){ 00286 00287 return SingleByteRead(ADXL345_FIFO_STATUS); 00288 00289 } 00290 00291 00292 00293 char ADXL345_I2C::getTapThreshold(void) { 00294 00295 return SingleByteRead(ADXL345_THRESH_TAP_REG); 00296 } 00297 00298 int ADXL345_I2C::setTapThreshold(char threshold) { 00299 00300 return SingleByteWrite(ADXL345_THRESH_TAP_REG, threshold); 00301 00302 } 00303 00304 00305 float ADXL345_I2C::getTapDuration(void) { 00306 00307 return (float)SingleByteRead(ADXL345_DUR_REG)*625; 00308 } 00309 00310 int ADXL345_I2C::setTapDuration(short int duration_us) { 00311 00312 short int tapDuration = duration_us / 625; 00313 char tapChar[2]; 00314 tapChar[0] = (tapDuration & 0x00FF); 00315 tapChar[1] = (tapDuration >> 8) & 0x00FF; 00316 return multiByteWrite(ADXL345_DUR_REG, tapChar, 2); 00317 00318 } 00319 00320 float ADXL345_I2C::getTapLatency(void) { 00321 00322 return (float)SingleByteRead(ADXL345_LATENT_REG)*1.25; 00323 } 00324 00325 int ADXL345_I2C::setTapLatency(short int latency_ms) { 00326 00327 latency_ms = latency_ms / 1.25; 00328 char latChar[2]; 00329 latChar[0] = (latency_ms & 0x00FF); 00330 latChar[1] = (latency_ms << 8) & 0xFF00; 00331 return multiByteWrite(ADXL345_LATENT_REG, latChar, 2); 00332 00333 } 00334 00335 float ADXL345_I2C::getWindowTime(void) { 00336 00337 return (float)SingleByteRead(ADXL345_WINDOW_REG)*1.25; 00338 } 00339 00340 int ADXL345_I2C::setWindowTime(short int window_ms) { 00341 00342 window_ms = window_ms / 1.25; 00343 char windowChar[2]; 00344 windowChar[0] = (window_ms & 0x00FF); 00345 windowChar[1] = ((window_ms << 8) & 0xFF00); 00346 return multiByteWrite(ADXL345_WINDOW_REG, windowChar, 2); 00347 00348 } 00349 00350 char ADXL345_I2C::getActivityThreshold(void) { 00351 00352 return SingleByteRead(ADXL345_THRESH_ACT_REG); 00353 } 00354 00355 int ADXL345_I2C::setActivityThreshold(char threshold) { 00356 return SingleByteWrite(ADXL345_THRESH_ACT_REG, threshold); 00357 00358 } 00359 00360 char ADXL345_I2C::getInactivityThreshold(void) { 00361 return SingleByteRead(ADXL345_THRESH_INACT_REG); 00362 00363 } 00364 00365 //int FUNCTION(short int * ptr_Output) 00366 //short int FUNCTION () 00367 00368 int ADXL345_I2C::setInactivityThreshold(char threshold) { 00369 return SingleByteWrite(ADXL345_THRESH_INACT_REG, threshold); 00370 00371 } 00372 00373 char ADXL345_I2C::getTimeInactivity(void) { 00374 00375 return SingleByteRead(ADXL345_TIME_INACT_REG); 00376 00377 } 00378 00379 int ADXL345_I2C::setTimeInactivity(char timeInactivity) { 00380 return SingleByteWrite(ADXL345_TIME_INACT_REG, timeInactivity); 00381 00382 } 00383 00384 char ADXL345_I2C::getActivityInactivityControl(void) { 00385 00386 return SingleByteRead(ADXL345_ACT_INACT_CTL_REG); 00387 00388 } 00389 00390 int ADXL345_I2C::setActivityInactivityControl(char settings) { 00391 return SingleByteWrite(ADXL345_ACT_INACT_CTL_REG, settings); 00392 00393 } 00394 00395 char ADXL345_I2C::getFreefallThreshold(void) { 00396 00397 return SingleByteRead(ADXL345_THRESH_FF_REG); 00398 00399 } 00400 00401 int ADXL345_I2C::setFreefallThreshold(char threshold) { 00402 return SingleByteWrite(ADXL345_THRESH_FF_REG, threshold); 00403 00404 } 00405 00406 char ADXL345_I2C::getFreefallTime(void) { 00407 00408 return SingleByteRead(ADXL345_TIME_FF_REG)*5; 00409 00410 } 00411 00412 int ADXL345_I2C::setFreefallTime(short int freefallTime_ms) { 00413 freefallTime_ms = freefallTime_ms / 5; 00414 char fallChar[2]; 00415 fallChar[0] = (freefallTime_ms & 0x00FF); 00416 fallChar[1] = (freefallTime_ms << 8) & 0xFF00; 00417 00418 return multiByteWrite(ADXL345_TIME_FF_REG, fallChar, 2); 00419 00420 } 00421 00422 char ADXL345_I2C::getTapAxisControl(void) { 00423 00424 return SingleByteRead(ADXL345_TAP_AXES_REG); 00425 00426 } 00427 00428 int ADXL345_I2C::setTapAxisControl(char settings) { 00429 return SingleByteWrite(ADXL345_TAP_AXES_REG, settings); 00430 00431 } 00432 00433 char ADXL345_I2C::getTapSource(void) { 00434 00435 return SingleByteRead(ADXL345_ACT_TAP_STATUS_REG); 00436 00437 } 00438 00439 00440 00441 char ADXL345_I2C::getInterruptEnableControl(void) { 00442 00443 return SingleByteRead(ADXL345_INT_ENABLE_REG); 00444 00445 } 00446 00447 int ADXL345_I2C::setInterruptEnableControl(char settings) { 00448 return SingleByteWrite(ADXL345_INT_ENABLE_REG, settings); 00449 00450 } 00451 00452 char ADXL345_I2C::getInterruptMappingControl(void) { 00453 00454 return SingleByteRead(ADXL345_INT_MAP_REG); 00455 00456 } 00457 00458 int ADXL345_I2C::setInterruptMappingControl(char settings) { 00459 return SingleByteWrite(ADXL345_INT_MAP_REG, settings); 00460 00461 } 00462 00463 char ADXL345_I2C::getInterruptSource(void){ 00464 00465 return SingleByteRead(ADXL345_INT_SOURCE_REG); 00466 00467 } 00468 00469 00470 00471
Generated on Wed Jul 13 2022 12:49:20 by
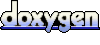