Driver for the NXP PCT2075 digital temperature sensor and thermal watchdog
Embed:
(wiki syntax)
Show/hide line numbers
PCT2075.h
00001 #ifndef _pct2075_h_ 00002 #define _pct2075_h_ 00003 00004 #include "mbed.h" 00005 00006 class PCT2075 { 00007 private: 00008 I2C &i2c; 00009 uint8_t address_write; 00010 uint8_t address_read; 00011 00012 public: 00013 static const int16_t TEMP_MAX = 12500; // centi-°C 00014 static const int16_t TEMP_MIN = -5500; // centi-°C 00015 static const uint16_t TIDLE_MAX = 3100; // ms 00016 static const uint16_t TIDLE_MIN = 100; // ms 00017 00018 // Fault queue is defined as the number of faults that must occur 00019 // consecutively to activate the OS output. It is provided to avoid false 00020 // tripping due to noise. Because faults are determined at the end of data 00021 // conversions, fault queue is also defined as the number of consecutive 00022 // conversions returning a temperature trip. 00023 enum OSFaultQue { 00024 OS_FAULT_QUE_1 = 0, 00025 OS_FAULT_QUE_2 = 1, 00026 OS_FAULT_QUE_4 = 2, 00027 OS_FAULT_QUE_6 = 3 00028 }; 00029 00030 enum OSPolarity { 00031 OS_ACTIVE_LOW = 0, 00032 OS_ACTIVE_HIGH = 1 00033 }; 00034 00035 enum OSMode { 00036 OS_MODE_COMP = 0, 00037 OS_MODE_INTERRUPT = 1 00038 }; 00039 00040 // In shutdown mode, the PCT2075 draws a small current of <1.0 µA and the 00041 // power dissipation is minimized; the temperature conversion stops, but the 00042 // I2C-bus interface remains active and register write/read operation can be 00043 // performed. When the shutdown is set, the OS output will be unchanged in 00044 // comparator mode and reset in interrupt mode. 00045 enum DeviceMode { 00046 DEVICE_MODE_NORMAL = 0, 00047 DEVICE_MODE_SHUTDOWN = 1 00048 }; 00049 00050 struct Configuration { 00051 OSFaultQue os_fault_que; 00052 OSPolarity os_polarity; 00053 OSMode os_mode; 00054 DeviceMode device_mode; 00055 }; 00056 00057 PCT2075 (I2C &i2c_obj, uint8_t address = 0x48); 00058 00059 ~PCT2075(); 00060 int16_t PCT2075_os_temp; 00061 int16_t PCT2075_hyst_temp; 00062 uint8_t PCT2075_temp_idle_cycle; 00063 00064 Configuration get_configuration(); 00065 void set_configuration(Configuration& config); 00066 00067 // Shorthand functions for changing one parameter of the configuration 00068 // without changing the other parameters. 00069 // Note: When changing multiple parameters it is more efficient to batch 00070 // them using set_configuration. 00071 void set_os_fault_queue(OSFaultQue fault_que); 00072 void set_os_polarity(OSPolarity polarity); 00073 void set_os_mode(OSMode mode); 00074 void set_device_mode(DeviceMode mode); 00075 void shutdown_mode(); 00076 void normal_mode(); 00077 00078 // Returns the temperature in centi-degrees C, 00079 // resolution of .125 degrees, rounded down to .1 degrees 00080 int16_t read_temperature(); 00081 00082 // Get the OS temperature in centi-degrees C, 00083 // rounded to .5 degrees C 00084 int16_t get_os_temperature(); 00085 // Set the OS temperature in centi-degrees C, 00086 // rounded to .5 degrees C 00087 void set_os_temperature(int16_t temperature); 00088 00089 // Get the hysteresis temperature in centi-degrees C, 00090 // rounded to .5 degrees C 00091 int16_t get_hyst_temperature(); 00092 // Set the hysteresis temperature in centi-degrees C, 00093 // rounded to .5 degrees C 00094 void set_hyst_temperature(int16_t temperature); 00095 00096 // Get idle time in ms, resolution of 100ms (deci-seconds) 00097 uint16_t get_idle_time(); 00098 // Set idle time in ms, rounded down to 100ms (deci-seconds) 00099 // In normal operation mode, the temp-to-digital conversion is executed 00100 // every 100 ms or other programmed value and the Temp register is updated 00101 // at the end of each conversion. During each ‘conversion period’ (Tconv) of 00102 // about 100 ms, the device takes only about 28 ms, called ‘temperature 00103 // conversion time’ (tconv(T)), to complete a temperature-to-data conversion 00104 // and then becomes idle for the time remaining in the period. This feature 00105 // is implemented to significantly reduce the device power dissipation. 00106 void set_idle_time(uint16_t time); 00107 }; 00108 00109 #endif // _PCT2075_H_
Generated on Thu Jul 21 2022 09:31:43 by
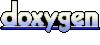