
principal
Dependencies: mbed fastlib vga640x400
main.c
00001 #include "mbed.h" 00002 #include "vga640x400/vga640x400.h" 00003 #include <string.h> 00004 #include <fichier.h> 00005 00006 00007 00008 // P8 et P25 pour horloge, P5 data 00009 //Serial pc(USBTX, USBRX); // tx, rx 00010 // This must be an SPI MOSI pin. 00011 #define DATA_PIN p11 00012 00013 00014 extern unsigned char font_lin[256*16]; 00015 Ticker timer1; 00016 00017 InterruptIn CAPT(p15 ); 00018 00019 DigitalIn BPA(p17); 00020 DigitalIn BPB(p18); 00021 DigitalIn BPC(p19); 00022 DigitalIn BPD(p20); 00023 00024 DigitalOut led1(LED1); 00025 DigitalOut led2(LED2); 00026 DigitalOut led3(LED3); 00027 DigitalOut led4(LED4); 00028 00029 void int_timer(void); 00030 void int_capt(void); 00031 void int_capt2(void); 00032 void vide(void); 00033 void tourne_roue(void); 00034 00035 int passage; 00036 00037 00038 void lancer_action(void){ 00039 00040 FILE *fp = fopen("/local/act.csv", "r"); 00041 if(!fp) { 00042 error("Could not open file!\n\r"); 00043 } 00044 00045 // 00046 00047 char ligne[500]; 00048 char stock[500]; 00049 char* morceau; 00050 bool passe = false; 00051 00052 int a = 1; 00053 while( a == 1) 00054 a = (rand() % nombre_ligne_fichier(fp)) +1; 00055 00056 printf("rand = %d\n\r",a); 00057 int b; 00058 00059 00060 // 00061 clear_buffer(); 00062 /*-----------------------------------*/ 00063 /* RETOURNE LE CONTENU DE LA LIGNE X */ 00064 /*-----------------------------------*/ 00065 retourne_ligne(a,ligne,fp); 00066 00067 printf("LIGNE CHOISI: %s\n\r", ligne); 00068 00069 /*--------------------------------*/ 00070 /* AFFICHE L'ACTION A LECRAN */ 00071 /*--------------------------------*/ 00072 morceau = retourne_element_ligne(1, ligne); 00073 strcpy(stock, morceau); 00074 printf("l'action est: %s \n\r", text_buffer); 00075 for (int i=0 ; i<strlen(stock)+1; i ++) 00076 text_buffer[(320+i)^1] = stock[i]; 00077 00078 00079 a =0; 00080 while (text_buffer[a] != '\0'){ 00081 a++; 00082 } 00083 printf("a comptage : %d\n\r", a); 00084 00085 /*-----------------------------------*/ 00086 /* AFFICHE LE TEMPS A LECRAN */ 00087 /*-----------------------------------*/ 00088 strcpy(stock, "RESTER APPUYER SUR UN BOUTON POUR PASSER ->"); 00089 for (int j=0 ; j<strlen(stock)+1; j++) 00090 text_buffer[(880+j)^1] = stock[j]; 00091 00092 morceau = retourne_element_ligne(2, ligne); 00093 b = atoi(morceau); 00094 00095 while(b >= 0 ){ 00096 wait(0.9); 00097 sprintf(stock, "%3d", b); 00098 printf("COMPTEUR: %s\n\r", stock); 00099 for (int i=0 ; i<strlen(stock)+1; i ++) 00100 text_buffer[((a/80+1)*80+i)^1] = stock[i]; 00101 b--; 00102 if(! (BPA == 1 && BPB == 1 && BPC == 1 && BPD == 1) ){ 00103 passe = true; 00104 clear_buffer(); 00105 while(!(BPA == 1 && BPB == 1 && BPC == 1 && BPD == 1) ){ 00106 } 00107 b=-1; 00108 } 00109 00110 } 00111 00112 /* 00113 if (!passe){ 00114 printf("dans le pas passe\n\r"); 00115 clear_buffer(); 00116 strcpy(stock, "APPUYER SUR UN BOUTON POUR CONTINUER -> "); 00117 for (int j=0 ; j<strlen(stock)+1; j++) 00118 text_buffer[(880+j)^1] = stock[j]; 00119 while(BPA == 1 && BPB == 1 && BPC == 1 && BPD == 1){ 00120 } 00121 while(! (BPA == 1 && BPB == 1 && BPC == 1 && BPD == 1) ){ 00122 } 00123 clear_buffer(); 00124 passe = false; 00125 } 00126 */ 00127 /*--------------------------------*/ 00128 /* AFFICHE LA RAISON A LECRAN */ 00129 /*--------------------------------*/ 00130 00131 00132 00133 morceau = retourne_element_ligne(3, ligne); 00134 strcpy(stock, morceau); 00135 for (int j=0 ; j<strlen(stock); j++) 00136 text_buffer[(400+j)^1] = stock[j]; 00137 00138 wait(0.5); 00139 strcpy(stock, "LANCER LA ROUE POUR REJOUER"); 00140 for (int j=0 ; j<strlen(stock)+1; j++) 00141 text_buffer[(1120+j)^1] = stock[j]; 00142 00143 tourne_roue(); 00144 /* 00145 while(BPA == 1){ 00146 } 00147 00148 while(BPA == 0){ 00149 } 00150 */ 00151 fclose(fp); 00152 printf("FIN\n\r"); 00153 00154 } 00155 00156 void lancer_question(void){ 00157 00158 FILE *fp = fopen("/local/form.csv", "r"); 00159 if(!fp) { 00160 error("Could not open file!\n\r"); 00161 } 00162 00163 // 00164 00165 char ligne[500]; 00166 char stock[500]; 00167 char* morceau; 00168 int a = (rand() % nombre_ligne_fichier(fp)) +1; 00169 printf("rand = %d\n\r",a); 00170 int b; 00171 00172 00173 // 00174 clear_buffer(); 00175 /*-----------------------------------*/ 00176 /* RETOURNE LE CONTENU DE LA LIGNE X */ 00177 /*-----------------------------------*/ 00178 retourne_ligne(a,ligne,fp); 00179 00180 /* 00181 for (int i=0 ; i<strlen(ligne)+1; i ++) 00182 text_buffer[(400+i)^1] = ligne[i]; //80 caractères par lignes 00183 00184 printf("ligne est %s\n\r",ligne); 00185 wait(5); 00186 clear_buffer(); 00187 */ 00188 00189 /*-----------------------------------*/ 00190 /* AFFICHE LA QUESTION A LECRAN */ 00191 /*-----------------------------------*/ 00192 morceau = retourne_element_ligne(1, ligne); 00193 strcpy(stock, morceau); 00194 for (int i=0 ; i<strlen(stock)+1; i ++) 00195 text_buffer[(400+i)^1] = stock[i]; 00196 printf("la question est: %s \n\r", text_buffer); 00197 00198 00199 a =0; 00200 while (text_buffer[a] != '\0'){ 00201 a++; 00202 } 00203 printf("a comptage : %d\n\r", a); 00204 /*-----------------------------------*/ 00205 /* AFFICHE LES REPONSES A LECRAN */ 00206 /*-----------------------------------*/ 00207 00208 for(int i = 1; i < 5; i++){ 00209 morceau = retourne_element_ligne(i+1, ligne); 00210 printf("Reponse %d: %s \n\r",i, morceau); 00211 strcpy(stock, morceau); 00212 for (int j=0 ; j<strlen(stock)+1; j++) 00213 text_buffer[((a/80+1)*80+j+i*160)^1] = stock[j]; 00214 } 00215 00216 00217 while(BPA == 1 && BPB == 1 && BPC == 1 && BPD == 1){ 00218 } 00219 00220 00221 unsigned int ba, bb, bc, bd; 00222 00223 ba = BPA; 00224 bb = BPB; 00225 bc = BPC; 00226 bd = BPD; 00227 00228 while(! (BPA == 1 && BPB == 1 && BPC == 1 && BPD == 1) ){ 00229 } 00230 00231 00232 printf("a: %d \n\r", ba); 00233 printf("a: %d \n\r", bb); 00234 printf("a: %d \n\r", bc); 00235 printf("a: %d \n\r", bd); 00236 if(!ba) 00237 b = 1; 00238 else if(!bb) 00239 b = 2; 00240 else if(!bc) 00241 b = 3; 00242 else if(!bd) 00243 b = 4; 00244 00245 clear_buffer(); 00246 00247 00248 /*----------------------------------------*/ 00249 /* AFFICHE LA BONNE REPONSES A LECRAN */ 00250 /*----------------------------------------*/ 00251 00252 a = num_bonne_reponse(ligne); 00253 00254 00255 if(a == b){ 00256 strcpy(stock, "BONNE REPONSE =)"); 00257 for (int j=0 ; j<strlen(stock)+1; j++) 00258 text_buffer[(1+j)^1] = stock[j]; 00259 } 00260 else{ 00261 strcpy(stock, "MAUVAISE REPONSE =("); 00262 for (int j=0 ; j<strlen(stock)+1; j++) 00263 text_buffer[(1+j)^1] = stock[j]; 00264 } 00265 morceau = retourne_element_ligne(a+1, ligne); 00266 strcpy(stock, morceau); 00267 for (int j=0 ; j<strlen(stock)+1; j++) 00268 text_buffer[(400+j)^1] = stock[j]; 00269 00270 00271 strcpy(stock, " PARCE QUE ->"); 00272 for (int j=0 ; j<strlen(stock)+1; j++) 00273 text_buffer[(560+j)^1] = stock[j]; 00274 00275 00276 morceau = retourne_element_ligne(7, ligne); 00277 strcpy(stock, morceau); 00278 for (int j=0 ; j<strlen(stock); j++) 00279 text_buffer[(640+j)^1] = stock[j]; 00280 00281 wait(0.5); 00282 strcpy(stock, "LANCER LA ROUE POUR REJOUER"); 00283 for (int j=0 ; j<strlen(stock)+1; j++) 00284 text_buffer[(1120+j)^1] = stock[j]; 00285 00286 00287 tourne_roue(); 00288 /*while(BPA == 1){ 00289 } 00290 00291 while(BPA == 0){ 00292 } 00293 00294 00295 while(BPA == 1 && BPB == 1 && BPC == 1 && BPD == 1){ 00296 } 00297 00298 while(! (BPA == 1 && BPB == 1 && BPC == 1 && BPD == 1) ){ 00299 } 00300 */ 00301 fclose(fp); 00302 printf("FIN\n\r"); 00303 00304 } 00305 00306 void acceuil(void){ 00307 00308 clear_buffer(); 00309 char stock[500]; 00310 00311 strcpy(stock, "BIENVENUE A ROU[E]COLO"); 00312 for (int j=0 ; j<strlen(stock)+1; j++) 00313 text_buffer[(400+j)^1] = stock[j]; 00314 00315 strcpy(stock, "LANCER LA ROUE POUR JOUER"); 00316 for (int j=0 ; j<strlen(stock)+1; j++) 00317 text_buffer[(1120+j)^1] = stock[j]; 00318 00319 tourne_roue(); 00320 /* 00321 while(BPA == 1){ 00322 } 00323 00324 while(BPA == 0){ 00325 } 00326 */ 00327 00328 return; 00329 } 00330 00331 void vide(void){ 00332 } 00333 00334 void int_timer(void){ 00335 passage = 2; 00336 } 00337 00338 void int_capt(){ 00339 passage = 1; 00340 } 00341 00342 void int_capt2(){ 00343 timer1.attach(&int_timer, 3); 00344 } 00345 00346 void tourne_roue(void){ 00347 CAPT.rise(&int_capt); 00348 CAPT.fall(&int_capt); 00349 00350 passage=0; 00351 while(passage == 0){ 00352 printf("passage 0 \n\r"); 00353 } 00354 00355 timer1.attach(&int_timer, 3); 00356 CAPT.rise(&int_capt2); 00357 CAPT.fall(&int_capt2); 00358 00359 while(passage == 1){ 00360 printf("passage 1 \n\r"); 00361 } 00362 printf("passage 2\n\r"); 00363 timer1.detach(); 00364 CAPT.rise(&vide); 00365 CAPT.fall(&vide); 00366 } 00367 00368 int main() { 00369 LocalFileSystem local("local"); 00370 00371 00372 font = font_lin; 00373 init_vga(); 00374 00375 00376 acceuil(); 00377 00378 00379 // 00380 while(1){ 00381 00382 if(CAPT.read() == 1) 00383 lancer_action(); 00384 else 00385 lancer_question(); 00386 00387 } 00388 }
Generated on Fri Jul 15 2022 05:10:10 by
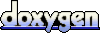