TSL1401 is the line sensor camera.This library is used to read the raw data. modified Polytec paris sud
Fork of TSL1401 by
TSL1401.cpp
00001 #include "TSL1401.h" 00002 #include "FastIO.h" 00003 #include "FastAnalogIn.h" 00004 /* Macro */ 00005 #define TAOS_SI_HIGH TAOS_SI = 1 00006 #define TAOS_SI_LOW TAOS_SI = 0 00007 #define TAOS_CLK_HIGH TAOS_CLK = 1 00008 #define TAOS_CLK_LOW TAOS_CLK = 0 00009 00010 FastAnalogIn AA1(p20,0); 00011 /* Constructor */ 00012 TSL1401::TSL1401(PinName s, PinName c, PinName a ) 00013 { 00014 00015 SI = s; 00016 CLK = c; 00017 A0 = new AnalogIn( a ); 00018 } 00019 /* Destructor */ 00020 TSL1401::~TSL1401() 00021 { 00022 if( A0 != NULL) 00023 { 00024 delete A0; 00025 } 00026 } 00027 /* Image Caputure */ 00028 int *TSL1401::Capture( int LineStart, int LineStop) 00029 { 00030 int i; 00031 FastOut<p9> TAOS_SI; 00032 FastOut<p10> TAOS_CLK; 00033 00034 /* DigitalOut TAOS_SI(SI); 00035 DigitalOut TAOS_CLK(CLK); 00036 */ 00037 Max = 0,Min = 70000; 00038 TAOS_SI_HIGH; 00039 TAOS_CLK_HIGH; 00040 TAOS_SI_LOW; 00041 ImageData[0] = 0; 00042 volatile int *pp=ImageData; 00043 TAOS_CLK_LOW; 00044 /* for(i = 1; i < LineStart; i++) { 00045 TAOS_CLK_HIGH; 00046 TAOS_CLK_HIGH; 00047 TAOS_CLK_LOW; 00048 } 00049 */ 00050 for(i = LineStart; i < LineStop; i++) { 00051 TAOS_CLK_HIGH; 00052 // TAOS_CLK_HIGH; 00053 TAOS_CLK_HIGH; 00054 // ImageData[i] = A0->read_u16(); // inputs data from camera (one pixel each time through loop) 00055 // ImageData[i] 00056 *pp = AA1.read_u16(); 00057 TAOS_CLK_LOW; 00058 Max = ( Max < *pp ? *pp : Max); 00059 // Max = ( Max < ImageData[i] ? ImageData[i] : Max); 00060 /*if(Max < ImageData[i]){ 00061 Max = ImageData[i]; 00062 } 00063 */ 00064 Min = (Min > *pp ? *pp : Min); 00065 pp++; 00066 //Min = (Min > ImageData[i] ? ImageData[i] : Min); 00067 00068 } 00069 return ImageData; 00070 } 00071 00072 00073
Generated on Mon Jul 25 2022 21:23:33 by
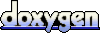