A WIP Roguelike game by Adekto, VonBednat, Trelemar and Wuuff made for CGAJam in spring 2017
Dependencies: PokittoLib
Fork of Arcade by
main.cpp
00001 #include "Pokitto.h" 00002 #include <vector> 00003 #include <string> 00004 #include "sprites.h" 00005 00006 #include "mapgen.c" 00007 #include "classes.cpp" 00008 00009 #define SEED 344 //Change this value for unique map every time. Hoping for RTC on Hardware! 00010 00011 bool solids[255]; 00012 00013 char dungeon[MAPSIZE][MAPSIZE]; 00014 int dungeonSize = 16;//Starting dungeon size, which increases each level 00015 int dungeonDepth = 1;//Which floor the player is on 00016 00017 bool solid(char map[][MAPSIZE],int x, int y){ 00018 return solids[map[y][x]]; 00019 } 00020 00021 void init_solids(){ 00022 for( int i = 1; i <= ID_COFFIN_OPEN_BOTTOM; i++ ) 00023 solids[i]=true; 00024 } 00025 00026 Pokitto::Core game; 00027 00028 int playerX = 1; 00029 int playerY = 2; 00030 00031 00032 void Ent::draw(){ //Classes are not implemented yet. This wont work either 00033 game.display.drawBitmap(playerX-x*14,playerY-y*14,sprites[id]); 00034 } 00035 00036 #define MAP_TILES 0 00037 #define ITEM_TILES (MAP_TILES + 34) 00038 #define ENTITY_TILES (ITEM_TILES + 36) 00039 00040 #define ID_PLAYER ENTITY_TILES + 2 00041 00042 #define ID_STAIRS_DOWN MAP_TILES + 19 00043 #define ID_GOBLIN_WARRIOR ENTITY_TILES + 5 00044 #define ID_GOBLIN_MAGE ENTITY_TILES + 6 00045 #define ID_SKELETON_MAGE ENTITY_TILES + 7 00046 #define ID_SKELETON_ARCHER ENTITY_TILES + 8 00047 #define ID_SKELETON_WARIOR ENTITY_TILES + 9 00048 #define ID_BLOOD_SKELETON ENTITY_TILES + 10 00049 #define ID_BLOOD ENTITY_TILES + 11 00050 #define ID_RAT ENTITY_TILES + 4 00051 #define ID_SCROLL ITEM_TILES + 3 00052 #define ID_CHEST ENTITY_TILES + 12 00053 #define ID_CHEST_OPEN ENTITY_TILES + 13 00054 #define ID_MIMIC ENTITY_TILES + 14 00055 #define ID_COIN ITEM_TILES 00056 #define ID_COINS ITEM_TILES + 1 00057 #define ID_BAG ITEM_TILES + 2 00058 00059 #define StateGame 0 00060 #define StateMenu 1 00061 #define StateIntro 2 00062 #define StateDead 3 00063 00064 void addDescent(char map[][MAPSIZE]){ 00065 int x=random(1,dungeonSize-1); 00066 int y=random(1,dungeonSize-1); 00067 if (!solid(map,x,y) && !solid(map,x-1,y) && !solid(map,x+1,y) && !solid(map,x,y-1) && !solid(map,x,y+1)) { 00068 map[y][x] = ID_STAIRS_DOWN; 00069 } 00070 else { 00071 addDescent(map); 00072 } 00073 } 00074 00075 //globals 00076 //std::vector<entity> entities(entities_size); 00077 struct entity{ 00078 uint8_t x; 00079 uint8_t y; 00080 int8_t hp; 00081 uint8_t id; 00082 }; 00083 #define ESIZE 2 00084 std::vector<entity> entities(ESIZE); 00085 std::vector<std::string> inventory; 00086 00087 void removeEntity(int i){ 00088 00089 using std::swap; 00090 std::swap(entities[i], entities.back()); 00091 entities.pop_back(); 00092 00093 } 00094 00095 void spawner(int amount){ 00096 entities.clear(); 00097 for(int i = 0; i < amount; i++ ){ 00098 entity spawn; 00099 bool l = true; 00100 int sx, sy; 00101 while(l){ 00102 sx = rand()%dungeonSize; 00103 sy = rand()%dungeonSize; 00104 if(dungeon[sy][sx] == 0){ 00105 spawn.id = ENTITY_TILES+4+(rand()%16);//rand()%8+ENTITY_TILES+4;//Skip first few entities for now 00106 spawn.x = sx; 00107 spawn.y = sy; 00108 spawn.hp = rand()%20; 00109 entities.push_back(spawn); 00110 l = false; 00111 } 00112 } 00113 } 00114 } 00115 00116 00117 char printer[40] = ""; 00118 int playerGold = 0; 00119 int playerHP = 100; 00120 uint8_t GameState = StateIntro; 00121 uint8_t MenuSelector = 0; 00122 #include "gui.h" 00123 #include "crapai.h" 00124 using namespace std; 00125 00126 00127 int main () { 00128 init_solids(); 00129 srand(SEED); 00130 mapinit(dungeon,dungeonSize,dungeonSize); 00131 mapgen(dungeon,dungeonSize,dungeonSize,0,0,dungeonSize-1,dungeonSize-1); 00132 mappretty(dungeon,dungeonSize,dungeonSize); 00133 addDescent(dungeon); 00134 std::vector<Ent> ents; 00135 inventory.push_back("adekto"); 00136 inventory.push_back("trelemar"); 00137 inventory.push_back("VonBednar"); 00138 inventory.push_back("wuuff"); 00139 Ent Etemp(3,3); 00140 ents.push_back(Etemp); 00141 pokInitSD(); // before game.begin if streaming 00142 game.sound.playMusicStream("coffins/coffins.snd"); // before game.begin if streaming 00143 game.begin(); 00144 game.sound.playMusicStream(); //after game.begin so that sound buffer is ready 00145 game.display.setFont(font5x7); 00146 00147 //mapgen(0,0,0,20,20); 00148 00149 game.display.loadRGBPalette(paletteCGA); 00150 //game.display.setFont(fontAdventurer); 00151 //game.display.persistence = true; 00152 game.display.setInvisibleColor(0); 00153 00154 spawner(ESIZE); 00155 /*entities[0].id = 12; 00156 entities[0].x = 5; 00157 entities[0].y = 5; 00158 entities[0].hp = rand()%20;*/ 00159 00160 uint8_t introspinner=0xFF; 00161 00162 while (game.isRunning()) { 00163 00164 if (game.update()) { 00165 00166 if( GameState == StateIntro){ 00167 if (introspinner<85) { 00168 game.display.setFont(fontAdventurer); 00169 game.display.setCursor(game.display.getWidth()/4-16,36); 00170 game.display.print("Columns & Coffins \n \n A Pokitto Roguelike \n \n PRESS A(z)"); 00171 game.display.setFont(font5x7); 00172 } else if (introspinner>160) { 00173 game.display.load565Palette(cnctitle_pal); 00174 game.display.drawBitmap(0,0,cnctitle); 00175 } else { 00176 game.display.loadRGBPalette(paletteCGA); 00177 game.display.drawBitmap(33,70,pokitteam); 00178 } 00179 if( game.buttons.held(BTN_A,0) ){ 00180 game.display.loadRGBPalette(paletteCGA); 00181 GameState = StateGame; 00182 } 00183 introspinner--; 00184 continue; 00185 } 00186 00187 if( GameState == StateDead){ 00188 game.display.setFont(fontAdventurer); 00189 game.display.setCursor(game.display.getWidth()/4,32); 00190 char over[60]; 00191 00192 sprintf(over,"Game Over \n \n You died on floor %i \n \n with %i gold",dungeonDepth,playerGold); 00193 game.display.print(over); 00194 //game.display.print(dungeonDepth); 00195 //game.display.print("\n \n You made it to year: "); 00196 //game.display.print("ERR");//Not implemented 00197 game.display.print("\n \n PRESS A"); 00198 if( game.buttons.held(BTN_A,0) ){ 00199 GameState = StateIntro; 00200 dungeonSize = 16; 00201 dungeonDepth = 1; 00202 playerHP = 100; 00203 playerX = 1; 00204 playerY = 2; 00205 mapinit(dungeon,dungeonSize,dungeonSize); 00206 mapgen(dungeon,dungeonSize,dungeonSize,0,0,dungeonSize-1,dungeonSize-1); 00207 mappretty(dungeon,dungeonSize,dungeonSize); 00208 addDescent(dungeon); 00209 spawner(ESIZE); 00210 } 00211 game.display.setFont(font5x7); 00212 continue; 00213 } 00214 00215 //If the player is standing on stairs down, generate a new bigger map 00216 if( dungeon[playerY][playerX] == ID_STAIRS_DOWN ){ 00217 if( dungeonSize + 2 < MAPSIZE ){ //As long as we aren't at maximum size 00218 dungeonSize += 2;//Increase map x and y by 2 00219 } 00220 dungeonDepth++; 00221 playerX = 1; 00222 playerY = 2; 00223 mapinit(dungeon,dungeonSize,dungeonSize); 00224 mapgen(dungeon,dungeonSize,dungeonSize,0,0,dungeonSize-1,dungeonSize-1); 00225 mappretty(dungeon,dungeonSize,dungeonSize); 00226 addDescent(dungeon); 00227 spawner(ESIZE); 00228 } 00229 00230 if (game.buttons.held(BTN_C,0)){ 00231 //doing it this way since more context may happen 00232 if(GameState == StateGame){ 00233 //game.display.rotatePalette(1); 00234 GameState = StateMenu; 00235 } 00236 else if(GameState == StateMenu){ 00237 //game.display.rotatePalette(-1); 00238 GameState = StateGame; 00239 MenuSelector = 0; 00240 isInventory = false; 00241 } 00242 } 00243 if(GameState == StateGame){ 00244 if( playerHP <= 0){ 00245 GameState = StateDead; 00246 continue; 00247 } 00248 00249 if (game.buttons.repeat(BTN_UP,4)){ 00250 if (!solids[dungeon[playerY-1][playerX]]){ 00251 if(entitiesLogic( playerX, playerY-1)) playerY --; 00252 } 00253 } 00254 if (game.buttons.repeat(BTN_DOWN,4)){ 00255 if (!solids[dungeon[playerY+1][playerX]]){ 00256 if(entitiesLogic( playerX, playerY+1)) playerY ++; 00257 } 00258 } 00259 if (game.buttons.repeat(BTN_LEFT,4)){ 00260 if (!solids[dungeon[playerY][playerX-1]]){ 00261 if(entitiesLogic( playerX-1, playerY))playerX --; 00262 } 00263 } 00264 if (game.buttons.repeat(BTN_RIGHT,4)){ 00265 if (!solids[dungeon[playerY][playerX+1]]){ 00266 if(entitiesLogic( playerX+1, playerY))playerX ++; 00267 } 00268 } 00269 } 00270 00271 00272 for(int x =playerX-7; x<playerX+8; x++){ //7 00273 for(int y =playerY-6; y<playerY+6; y++){ 00274 if(x >= 0 && y >= 0 && x < dungeonSize && y < dungeonSize){ 00275 game.display.drawBitmap(14*(x-playerX+7),14*(y-playerY+6),sprites[dungeon[y][x]]); 00276 } 00277 } 00278 } 00279 00280 for(int i=0; i<entities.size(); ++i){ 00281 game.display.color = 0; //remove before release 00282 game.display.fillRect(14*(entities[i].x-playerX+7),14*(entities[i].y-playerY+6),14,14);//remove and fix before release 00283 game.display.drawBitmap(14*(entities[i].x-playerX+7),14*(entities[i].y-playerY+6),sprites[entities[i].id]); 00284 } 00285 00286 game.display.setCursor(0,168); 00287 game.display.color = 1; 00288 game.display.print(printer); 00289 00290 00291 00292 drawHP( playerHP); 00293 //ents[0].draw(); 00294 00295 game.display.drawBitmap(14*(7),14*(6),sprites[ID_PLAYER]); 00296 00297 if(GameState == StateMenu){ 00298 drawMenu( 1,1, MenuSelector,1); 00299 } 00300 } 00301 00302 } 00303 00304 return 1; 00305 } 00306 00307
Generated on Sun Jul 17 2022 15:24:13 by
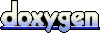