Armageddon - a missile commad clone by Wuuff, originally for Gamebuino. Conversion by Jonne
Dependencies: PokittoLib
Fork of Asterocks by
ageddon.cpp
00001 /* This file was automatically parsed from an Arduino sourcefile */ 00002 /* by PokittoParser v0.1 Copyright 2016 Jonne Valola */ 00003 /* USE AT YOUR OWN RISK - NO GUARANTEES GIVEN OF 100% CORRECTNESS */ 00004 00005 #include "PokittoCore.h" 00006 #include "PokittoCookie.h" 00007 00008 #define NUM_HIGHSCORES 5 00009 #define NAME_SIZE 10 00010 #define ENTRY_SIZE 15 00011 00012 /* Writing directly to EEPROM is deprecated */ 00013 /* 00014 uint32_t highscores[NUM_HIGHSCORES] = {10000,7000,5000,4000,2000}; 00015 char names[NUM_HIGHSCORES][NAME_SIZE+1] = {"Crack Shot", "Defender", "Gunner", "We Tried", "No Hope"}; 00016 */ 00017 00018 /* This is how you do it with the PokittoCookie class !!!*/ 00019 class gdata : public Pokitto::Cookie { 00020 public: 00021 uint32_t highscores[NUM_HIGHSCORES]; 00022 char names[NUM_HIGHSCORES][NAME_SIZE+1]; 00023 int check_number; 00024 gdata() { 00025 } 00026 }; 00027 00028 gdata gamedata; 00029 00030 /* Auto-generated function declarations */ 00031 void setup(); 00032 void nextStage(); 00033 void nextLull(); 00034 void drawScore(); 00035 void drawTargets(); 00036 void drawCities(); 00037 void drawAmmo(); 00038 void drawMissiles(); 00039 void drawDetonations(); 00040 void launchMissile(uint8_t); 00041 void tryLaunchEnemy(); 00042 void stepMissiles(); 00043 void stepDetonations(); 00044 void stepCollision(); 00045 void checkMenu(); 00046 void checkWin(); 00047 void checkLose(); 00048 void stepGame(); 00049 void drawLull(); 00050 void stepLull(); 00051 void stepDead(); 00052 void stepPregame(); 00053 void loop(); 00054 void loadHighscores(); 00055 uint8_t isHighscore(uint32_t); 00056 void saveHighscore(uint32_t,char*); 00057 void drawHighscores(); 00058 void initSound(); 00059 void playSound(uint8_t); 00060 00061 00062 Pokitto::Core gb; // game object 00063 00064 00065 int main() { 00066 setup(); 00067 gb.display.setDefaultPalette(); 00068 while (gb.isRunning()) { 00069 loop(); 00070 } 00071 } 00072 00073 #define WIDTH 110 00074 #define HEIGHT 88 00075 #define TEXT_WIDTH 6 00076 #define TEXT_HEIGHT 8 00077 00078 const byte armageddon[] PROGMEM = {112,88, 00079 B11111111,B11111111,B11111000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B01111111,B11111111,B11111100, 00080 B11111111,B11111111,B11111000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B01111111,B11111111,B11111100, 00081 B11111111,B11000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00001111,B11111100, 00082 B11111111,B11000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00001111,B11111100, 00083 B11111111,B11000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00001111,B11111100, 00084 B11111000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B01111100, 00085 B11111000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B01111100, 00086 B11000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00001100, 00087 B11000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00001100, 00088 B11000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00001100, 00089 B00000001,B10000001,B11100000,B00110000,B11000000,B11000000,B00111000,B00111111,B10011110,B00000111,B10000000,B00011000,B00011000,B01100000, 00090 B00000001,B10000001,B11100000,B00110000,B11000000,B11000000,B00111000,B00111111,B10011110,B00000111,B10000000,B00011000,B00011000,B01100000, 00091 B00000001,B10000001,B10011000,B00111001,B11000000,B11000000,B11000110,B00110000,B00011001,B10000110,B01100000,B01100110,B00011100,B01100000, 00092 B00000001,B10000001,B10011000,B00111001,B11000000,B11000000,B11000110,B00110000,B00011001,B10000110,B01100000,B01100110,B00011100,B01100000, 00093 B00000001,B10000001,B10011000,B00111001,B11000000,B11000000,B11000110,B00110000,B00011001,B10000110,B01100000,B01100110,B00011100,B01100000, 00094 B00000110,B01100001,B11111000,B00111001,B11000011,B00110000,B11000000,B00111111,B00011000,B01100110,B00011001,B10000001,B10011011,B01100000, 00095 B00000110,B01100001,B11111000,B00111001,B11000011,B00110000,B11000000,B00111111,B00011000,B01100110,B00011001,B10000001,B10011011,B01100000, 00096 B00000111,B11100001,B10011000,B00110110,B11000011,B11110000,B11001110,B00110000,B00011000,B01100110,B00011001,B10000001,B10011000,B11100000, 00097 B00000111,B11100001,B10011000,B00110110,B11000011,B11110000,B11001110,B00110000,B00011000,B01100110,B00011001,B10000001,B10011000,B11100000, 00098 B00000111,B11100001,B10011000,B00110110,B11000011,B11110000,B11001110,B00110000,B00011000,B01100110,B00011001,B10000001,B10011000,B11100000, 00099 B00000110,B01100001,B10011100,B00110000,B11000011,B00110000,B11000110,B00110000,B00011001,B10000110,B01100000,B01100110,B00011000,B01100000, 00100 B00000110,B01100001,B10001110,B00110000,B11000011,B00110000,B11000110,B00110000,B00011001,B10000110,B01100000,B01100110,B00011000,B01100000, 00101 B00011110,B01111001,B10000110,B00110000,B11001111,B00111100,B00111000,B00111111,B10011110,B00000111,B10000000,B00011000,B00011000,B01100000, 00102 B00011110,B01111001,B10000110,B00110000,B11001111,B00111100,B00111000,B00111111,B10011110,B00000111,B10000000,B00011000,B00011000,B01100000, 00103 B11000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00001100, 00104 B11000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00001100, 00105 B11000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00001100, 00106 B11100000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00011100, 00107 B11100000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00000000,B00011100, 00108 B11111000,B00000000,B00000000,B00000000,B01111111,B10000000,B00000000,B00000000,B00000111,B11111000,B00000000,B00000000,B00000000,B01111100, 00109 B11111000,B00000000,B00000000,B00000000,B01111111,B10000000,B00000000,B00000000,B00000111,B11111000,B00000000,B00000000,B00000000,B01111100, 00110 B11111000,B00000000,B00000000,B00000000,B01111111,B10000000,B00000000,B00000000,B00000111,B11111000,B00000000,B00000000,B00000000,B01111100, 00111 B11111111,B10000000,B00000000,B00111111,B11111111,B11111100,B00000000,B00000000,B11111111,B11111111,B11110000,B00000000,B00000111,B11111100, 00112 B11111111,B10000000,B00000000,B00111111,B11111111,B11111100,B00000000,B00000000,B11111111,B11111111,B11110000,B00000000,B00000111,B11111100, 00113 B11111111,B11110000,B00000111,B11111111,B11111111,B11111111,B00000000,B00000011,B11111111,B11111111,B11111111,B10000000,B00111111,B11111100, 00114 B11111111,B11110000,B00000111,B11111111,B11111111,B11111111,B00000000,B00000011,B11111111,B11111111,B11111111,B10000000,B00111111,B11111100, 00115 B11111111,B11110000,B00000111,B11111111,B11111111,B11111111,B00000000,B00000011,B11111111,B11111111,B11111111,B10000000,B00111111,B11111100, 00116 B11111111,B11111111,B11111111,B11111111,B11111111,B11111111,B00000000,B00000011,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00117 B11111111,B11111111,B11111111,B11111111,B11111111,B11111111,B00000000,B00000011,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00118 B11111111,B11111111,B11111111,B11111111,B11111111,B11111111,B11000000,B00001111,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00119 B11111111,B11111111,B11111111,B11111111,B11111111,B11111111,B11000000,B00001111,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00120 B11111111,B11111111,B11111111,B11111111,B11111111,B11111111,B11000000,B00001111,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00121 B11111111,B11111111,B11111111,B11111111,B11111111,B11111111,B11000000,B00001111,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00122 B11111111,B11111111,B11111111,B11111111,B11111111,B11111111,B11000000,B00001111,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00123 B11111111,B11111111,B11111111,B11111111,B11111111,B00000000,B00000000,B00000000,B00000011,B11111111,B11111111,B11111111,B11111111,B11111100, 00124 B11111111,B11111111,B11111111,B11111111,B11111111,B00000000,B00000000,B00000000,B00000011,B11111111,B11111111,B11111111,B11111111,B11111100, 00125 B11111111,B11111111,B11111111,B11111111,B10000000,B11111111,B11000000,B00001111,B11111100,B00000111,B11111111,B11111111,B11111111,B11111100, 00126 B11111111,B11111111,B11111111,B11111111,B10000000,B11111111,B11000000,B00001111,B11111100,B00000111,B11111111,B11111111,B11111111,B11111100, 00127 B11111111,B11111111,B11111111,B11111111,B10000000,B11111111,B11000000,B00001111,B11111100,B00000111,B11111111,B11111111,B11111111,B11111100, 00128 B11111111,B11111111,B11111111,B11111110,B01111111,B11111111,B00000000,B00000011,B11111111,B11111001,B11111111,B11111111,B11111111,B11111100, 00129 B11111111,B11111111,B11111111,B11111110,B01111111,B11111111,B00000000,B00000011,B11111111,B11111001,B11111111,B11111111,B11111111,B11111100, 00130 B11111111,B11111111,B11111111,B11111110,B01111111,B11111111,B00000000,B00000011,B11111111,B11111001,B11111111,B11111111,B11111111,B11111100, 00131 B11111111,B11111111,B11111111,B11111110,B01111111,B11111111,B00000000,B00000011,B11111111,B11111001,B11111111,B11111111,B11111111,B11111100, 00132 B11111111,B11111111,B11111111,B11111110,B01111111,B11111111,B00000000,B00000011,B11111111,B11111001,B11111111,B11111111,B11111111,B11111100, 00133 B11111111,B11111111,B11111111,B11111110,B01111111,B11111111,B00000000,B00000011,B11111111,B11111001,B11111111,B11111111,B11111111,B11111100, 00134 B11111111,B11111111,B11111111,B11111110,B01111111,B11111111,B00000000,B00000011,B11111111,B11111001,B11111111,B11111111,B11111111,B11111100, 00135 B11111111,B11111111,B11111111,B11111111,B10000000,B11111111,B00000000,B00000011,B11111100,B00000111,B11111111,B11111111,B11111111,B11111100, 00136 B11111111,B11111111,B11111111,B11111111,B10000000,B11111111,B00000000,B00000011,B11111100,B00000111,B11111111,B11111111,B11111111,B11111100, 00137 B11111111,B11111111,B11111111,B11111111,B10000000,B11111111,B00000000,B00000011,B11111100,B00000111,B11111111,B11111111,B11111111,B11111100, 00138 B11111111,B11111111,B11111111,B11111111,B11111111,B00000011,B00000000,B00000011,B00000011,B11111111,B11111111,B11111111,B11111111,B11111100, 00139 B11111111,B11111111,B11111111,B11111111,B11111111,B00000011,B00000000,B00000011,B00000011,B11111111,B11111111,B11111111,B11111111,B11111100, 00140 B11111111,B11111111,B11111111,B11111111,B11111111,B11111100,B00000000,B00000000,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00141 B11111111,B11111111,B11111111,B11111111,B11111111,B11111100,B00000000,B00000000,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00142 B11111111,B11111111,B11111111,B11111111,B11111111,B11111100,B00000000,B00000000,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00143 B11111111,B11111111,B11111111,B11111111,B11111111,B11111111,B00000000,B00000011,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00144 B11111111,B11111111,B11111111,B11111111,B11111111,B11111111,B00000000,B00000011,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00145 B11111111,B11111111,B11111111,B11111111,B11111111,B11111100,B00000000,B00000000,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00146 B11111111,B11111111,B11111111,B11111111,B11111111,B11111100,B00000000,B00000000,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00147 B11111111,B11111111,B11111111,B11111111,B11111111,B11111100,B00000000,B00000000,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00148 B11111111,B11111111,B11111111,B11111111,B11111111,B11111100,B00000000,B00000000,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00149 B11111111,B11111111,B11111111,B11111111,B11111111,B11111100,B00000000,B00000000,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00150 B11111111,B11111111,B11111111,B11111111,B11111111,B11111100,B00000000,B00000000,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00151 B11111111,B11111111,B11111111,B11111111,B11111111,B11111100,B00000000,B00000000,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00152 B11111111,B11111111,B11111111,B11111111,B11111111,B11111100,B00000000,B00000000,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00153 B11111111,B11111111,B11111111,B11111111,B11111111,B11111100,B00000000,B00000000,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00154 B11111111,B11111111,B11111111,B11111111,B11111111,B11111100,B00000000,B00000000,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00155 B11111111,B11111111,B11111111,B11111111,B11111111,B11111100,B00000000,B00000000,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00156 B11111111,B11111111,B11111111,B11111111,B11111111,B11111100,B00000000,B00000000,B11111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00157 B11111111,B11111111,B11111111,B11111111,B11111111,B11111000,B00000000,B00000000,B01111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00158 B11111111,B11111111,B11111111,B11111111,B11111111,B11111000,B00000000,B00000000,B01111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00159 B11111111,B11111111,B11111111,B11111111,B11111111,B11111000,B00000000,B00000000,B01111111,B11111111,B11111111,B11111111,B11111111,B11111100, 00160 B11111111,B11111111,B11111111,B11111111,B11111111,B11100000,B00000000,B00000000,B00011111,B11111111,B11111111,B11111111,B11111111,B11111100, 00161 B11111111,B11111111,B11111111,B11111111,B11111111,B11100000,B00000000,B00000000,B00011111,B11111111,B11111111,B11111111,B11111111,B11111100, 00162 B11111111,B11111111,B11111111,B11111111,B11111111,B00000000,B00000000,B00000000,B00000011,B11111111,B11111111,B11111111,B11111111,B11111100, 00163 B11111111,B11111111,B11111111,B11111111,B11111111,B00000000,B00000000,B00000000,B00000011,B11010101,B01010100,B01000111,B00010001,B01000100, 00164 B11111111,B11111111,B11111111,B11111111,B11111111,B00000000,B00000000,B00000000,B00000011,B11010101,B01010101,B11011111,B11010101,B01110100, 00165 B11111111,B11111111,B11111111,B11111111,B11111100,B00000000,B00000000,B00000000,B00000000,B11000101,B01010100,B11001111,B00110101,B01101100, 00166 B11111111,B11111111,B11111111,B11111111,B11111100,B00000000,B00000000,B00000000,B00000000,B11010100,B01000101,B11011111,B00010001,B01011100, 00167 }; 00168 00169 /*const byte city[] PROGMEM = {8,8, 00170 B00000000, 00171 B00000000, 00172 B00000000, 00173 B00000000, 00174 B00100010, 00175 B01110110, 00176 B01111110, 00177 B11111111, 00178 };*/ 00179 00180 const byte city[] PROGMEM = {8,8, 00181 B00000000, 00182 B00000000, 00183 B00000000, 00184 B00000000, 00185 B01100100, 00186 B01101110, 00187 B01111111, 00188 B11111111, 00189 }; 00190 00191 const byte deadcity[] PROGMEM = {8,8, 00192 B00000000, 00193 B00000000, 00194 B00000000, 00195 B00000000, 00196 B00000000, 00197 B00000000, 00198 B00100000, 00199 B01100010, 00200 }; 00201 00202 const byte launcher[] PROGMEM = {8,8, 00203 B00011000, 00204 B00011000, 00205 B00011000, 00206 B00100100, 00207 B00100100, 00208 B01000010, 00209 B01000010, 00210 B10000001, 00211 }; 00212 00213 const byte deadlauncher[] PROGMEM = {8,8, 00214 B00000000, 00215 B00000000, 00216 B00000000, 00217 B00000000, 00218 B00000000, 00219 B01100110, 00220 B01011010, 00221 B10000001, 00222 }; 00223 00224 #define SOUND_PLAUNCH 0 00225 #define SOUND_ELAUNCH 1 00226 #define SOUND_DETONATE 2 00227 #define SOUND_SCORE 3 00228 #define SOUND_DEAD 4 00229 #define SOUND_LOSE 5 00230 00231 #define MODE_PREGAME 0 00232 #define MODE_GAME 1 00233 #define MODE_LULL 2 00234 #define MODE_DEAD 3 00235 #define MODE_POSTDEAD 4 00236 00237 #define TARGET_SPEED 3 00238 00239 #define LAUNCHER_ONE 1 00240 #define LAUNCHER_TWO 2 00241 #define MAX_PMISSILES 10 00242 #define PSPEED 4 00243 #define PRADIUS 7 00244 #define EXPAND 0 00245 #define SHRINK 1 00246 00247 #define MAX_EMISSILES 10 00248 #define MAX_CHANCE 50 00249 00250 // State variables 00251 uint8_t mode = 0; 00252 uint8_t counter = 0; 00253 uint8_t flash = 0; 00254 uint8_t stage = 0; //Maximum of 255 stages 00255 uint32_t score = 0; //Score needs more space than other vars 00256 00257 uint32_t lullMissiles = 0; 00258 uint8_t lullCities[8] = {0,0,0,0,0,0,0,0}; 00259 00260 uint8_t cities[8] = {1,1,1,1,1,1,1,1}; //Whether the cities or launchers are alive 00261 00262 uint8_t targetX = WIDTH/2; 00263 uint8_t targetY = HEIGHT/2; 00264 uint8_t pammo[2] = {10,10}; 00265 uint8_t pDests[MAX_PMISSILES][2] = {{100,100},{100,100},{100,100},{100,100},{100,100},{100,100},{100,100},{100,100},{100,100},{100,100}}; 00266 float pMissiles[MAX_PMISSILES][3] = {{100,100,0},{100,100,0},{100,100,0},{100,100,0},{100,100,0},{100,100,0},{100,100,0},{100,100,0},{100,100,0},{100,100,0}}; 00267 uint8_t pDetonations[MAX_PMISSILES][4] = {{100,100,0,0},{100,100,0,0},{100,100,0,0},{100,100,0,0},{100,100,0,0},{100,100,0,0},{100,100,0,0},{100,100,0,0},{100,100,0,0},{100,100,0,0}}; 00268 00269 uint8_t etotal = 5; 00270 uint8_t echance = 1; 00271 float espeed = 0.2; 00272 uint8_t eDests[MAX_EMISSILES] = {100,100,100,100,100,100,100,100,100,100}; 00273 float eMissiles[MAX_EMISSILES][4] = {{100,100,100,100},{100,100,100,100},{100,100,100,100},{100,100,100,100},{100,100,100,100},{100,100,100,100},{100,100,100,100},{100,100,100,100},{100,100,100,100},{100,100,100,100}}; 00274 00275 void doTitle(uint8_t is_pause){ 00276 while (gb.isRunning()) { 00277 if( gb.update() ){ 00278 gb.display.setColor(7); 00279 gb.display.drawBitmap(0,0,armageddon); 00280 if( counter%8 == 0 ){ 00281 flash ^= 255; 00282 } 00283 00284 if( flash ){ 00285 gb.display.setColor(4); 00286 gb.display.cursorY = HEIGHT - TEXT_HEIGHT*2; 00287 if( is_pause ){ 00288 gb.display.cursorX = WIDTH/2 - TEXT_WIDTH*3; 00289 gb.display.print(("PAUSED")); 00290 }else{ 00291 gb.display.cursorX = WIDTH/2 - TEXT_WIDTH*3; 00292 gb.display.print(("PRESS \25")); 00293 } 00294 } 00295 counter++; 00296 if( gb.buttons.pressed(BTN_A) ){ 00297 gb.display.setColor(WHITE); 00298 break; 00299 } 00300 } 00301 } 00302 } 00303 00304 void setup() { 00305 loadHighscores(); // do this before gb.begin !! 00306 gb.begin(); 00307 00308 00309 00310 //We need to do extra Gamebuino-formatting-specific initialization if we don't use the title screen 00311 gb.display.setFont(font5x7); 00312 gb.display.fontSize = 1; 00313 gb.display.textWrap = false; 00314 gb.display.persistence = false; 00315 gb.battery.show = false; 00316 gb.display.setColor(BLACK); 00317 gb.display.setColorDepth(1); 00318 00319 doTitle(0); 00320 gb.pickRandomSeed(); 00321 } 00322 00323 void nextStage(){ 00324 stage++; 00325 //Reset cities (since we cleared them for the visual effect 00326 for( uint8_t i = 0; i < 8; i++ ){ 00327 cities[i] = lullCities[i]; 00328 } 00329 //Reset launchers 00330 cities[2] = 1; 00331 cities[5] = 1; 00332 //Reset missiles 00333 pammo[0] = 10; 00334 pammo[1] = 10; 00335 //Reset in-flight missiles 00336 for( uint8_t i = 0; i < 10; i++ ){ 00337 pDests[i][0] = 127; 00338 pDetonations[i][0] = 127; 00339 eDests[i] = 127; 00340 } 00341 00342 etotal = stage > 10 ? 20 : 10+stage; //Max of 20 missiles per stage, start at 10 00343 //echance = stage > 5 ? 5 : stage; //Max of 5/100 chance per frame 00344 espeed = stage > 18 ? 2 : 0.2+(stage*0.1); //Max speed of 2, up 0.1 per stage, starts at 0.2 00345 } 00346 00347 void nextLull(){ 00348 //Reset lullMissiles 00349 lullMissiles = 0; 00350 //Reset lullCities 00351 for( uint8_t i = 0; i < 8; i++ ){ 00352 lullCities[i] = 0; 00353 } 00354 } 00355 00356 void drawScore(){ 00357 gb.display.cursorX = WIDTH/2 - TEXT_WIDTH*3; 00358 gb.display.cursorY = 0; 00359 00360 if( score < 100000 ){ 00361 gb.display.print(0); 00362 } 00363 if( score < 10000 ){ 00364 gb.display.print(0); 00365 } 00366 if( score < 1000 ){ 00367 gb.display.print(0); 00368 } 00369 if( score < 100 ){ 00370 gb.display.print(0); 00371 } 00372 if( score < 10 ){ 00373 gb.display.print(0); 00374 } 00375 gb.display.print(score); 00376 } 00377 00378 void drawTargets(){ 00379 gb.display.drawFastHLine(targetX-1,targetY,3); 00380 gb.display.drawFastVLine(targetX,targetY-1,2); 00381 00382 for(uint8_t i = 0; i < MAX_PMISSILES; i++){ 00383 //Check for a valid destination without a current detonation 00384 if( pDests[i][0] <= WIDTH && pDetonations[i][0] > WIDTH ){ 00385 gb.display.drawPixel(pDests[i][0], pDests[i][1]); 00386 } 00387 } 00388 } 00389 00390 void drawCities(){ 00391 gb.display.setColor(4); 00392 //uint8_t alldead = 1; 00393 for(uint8_t i = 0; i < 8; i++){ 00394 if( i == 2 || i == 5 ){ 00395 if( cities[i] ){ 00396 gb.display.drawBitmap(i*14+2,HEIGHT-8,launcher); 00397 }else{ 00398 gb.display.drawBitmap(i*14+2,HEIGHT-8,deadlauncher); 00399 } 00400 }else{ 00401 if( cities[i] ){ 00402 //alldead = 0; 00403 gb.display.drawBitmap(i*14+2,HEIGHT-8,city); 00404 }else{ 00405 gb.display.drawBitmap(i*14+2,HEIGHT-8,deadcity); 00406 } 00407 } 00408 } 00409 00410 gb.display.setColor(1); 00411 /* 00412 if( alldead ){ 00413 gb.display.cursorX = 84/2 - 5*3; 00414 gb.display.cursorY = 48/2 - 5; 00415 gb.display.print("THE END"); 00416 } 00417 */ 00418 } 00419 00420 void drawAmmo(){ 00421 gb.display.setColor(3); 00422 for( uint8_t i = 0; i < 2; i++ ){ 00423 uint8_t xcoord = i == 0 ? 2*14 + 5 : 5*14 + 5; 00424 uint8_t ycoord = HEIGHT-1; 00425 if( cities[i*3+2] ){ //Is launcher alive? 00426 for( uint8_t j = 0; j < pammo[i]; j++ ){ 00427 gb.display.drawPixel(xcoord,ycoord); 00428 if( xcoord % 2 == 0 ){ 00429 xcoord--; 00430 ycoord--; 00431 }else{ 00432 xcoord++; 00433 } 00434 } 00435 } 00436 } 00437 gb.display.setColor(1); 00438 } 00439 00440 void drawMissiles(){ 00441 //Player Missiles 00442 gb.display.setColor(3); 00443 for(uint8_t i = 0; i < MAX_PMISSILES; i++){ 00444 //Check for a valid destination without a current detonation 00445 if( pDests[i][0] <= WIDTH && pDetonations[i][0] > WIDTH ){ 00446 if(pMissiles[i][2] == LAUNCHER_ONE){ 00447 gb.display.drawLine(2*14 + 5,HEIGHT-8,pMissiles[i][0], pMissiles[i][1]); 00448 }else{ 00449 gb.display.drawLine(5*14 + 6,HEIGHT-8,pMissiles[i][0], pMissiles[i][1]); 00450 } 00451 } 00452 } 00453 00454 //Enemy Missiles 00455 gb.display.setColor(7); 00456 for(uint8_t i = 0; i < MAX_EMISSILES; i++){ 00457 //Check for a valid destination 00458 if( eDests[i] <= WIDTH ){ 00459 gb.display.drawLine(eMissiles[i][0],eMissiles[i][1],eMissiles[i][2], eMissiles[i][3]); 00460 } 00461 } 00462 gb.display.setColor(1); 00463 } 00464 00465 uint8_t explosionColor = 0; 00466 void drawDetonations(){ 00467 gb.display.setColor(explosionColor+1); 00468 if(counter%2 == 0){ 00469 explosionColor++; 00470 explosionColor%=7; 00471 } 00472 for(uint8_t i = 0; i < MAX_PMISSILES; i++){ 00473 if( pDetonations[i][0] <= WIDTH ){ 00474 gb.display.fillCircle(pDetonations[i][0],pDetonations[i][1],pDetonations[i][2]); 00475 } 00476 } 00477 gb.display.setColor(1); 00478 } 00479 00480 void launchMissile(uint8_t launcher){ 00481 //If there is no free slot in pDests, do not launch 00482 for(uint8_t i = 0; i < MAX_PMISSILES; i++){ 00483 if( pDests[i][0] > WIDTH ){ 00484 // Which launcher? Alive? Has ammo? 00485 if(launcher == LAUNCHER_ONE && cities[2] && pammo[0]){ 00486 pDests[i][0] = targetX; 00487 pDests[i][1] = targetY; 00488 pMissiles[i][0] = 2*14 + 5; //X-coord of left launcher 00489 pMissiles[i][2] = LAUNCHER_ONE; //Launched from launcher one 00490 pammo[0]--; 00491 playSound(SOUND_PLAUNCH); 00492 }else if(launcher == LAUNCHER_TWO && cities[5] && pammo[1]){ 00493 pDests[i][0] = targetX; 00494 pDests[i][1] = targetY; 00495 pMissiles[i][0] = 5*14 + 6; //X-coord of right launcher 00496 pMissiles[i][2] = LAUNCHER_TWO; //Launched from launcher two 00497 pammo[1]--; 00498 playSound(SOUND_PLAUNCH); 00499 } 00500 pMissiles[i][1] = HEIGHT-8; //Y-coord of both launchers 00501 break; 00502 } 00503 } 00504 } 00505 00506 void tryLaunchEnemy(){ 00507 uint8_t someActive = 0; 00508 00509 if( etotal > 0 ){ 00510 00511 // Check whether there are any active missiles 00512 // If none are active, always spawn one to avoid 00513 // long pauses without any enemy missiles 00514 for(uint8_t i = 0; i < MAX_EMISSILES; i++){ 00515 if( eDests[i] <= WIDTH ){ 00516 someActive = 1; 00517 break; 00518 } 00519 } 00520 00521 if( (!someActive || echance >= random(100)) ){ //echance of 100 00522 for(uint8_t i = 0; i < MAX_EMISSILES; i++){ 00523 if( eDests[i] > WIDTH ){ 00524 etotal--; 00525 eDests[i] = random(8); //Target one of the 6 cities or 2 launch sites TODO: Come back later if random's behavior changes 00526 eMissiles[i][0] = random(WIDTH); //Screen width //TODO: if random is supposed to be inclusive, add a -1 00527 eMissiles[i][1] = 0; //Top of screen 00528 eMissiles[i][2] = eMissiles[i][0]; //Start and end are same 00529 eMissiles[i][3] = 0; //Top of screen 00530 playSound(SOUND_ELAUNCH); 00531 break; //Only spawn one 00532 } 00533 } 00534 } 00535 00536 } 00537 } 00538 00539 void stepMissiles(){ 00540 //Player Missiles 00541 for(uint8_t i = 0; i < MAX_PMISSILES; i++){ 00542 //Check for a valid destination without a current detonation 00543 if( pDests[i][0] <= WIDTH && pDetonations[i][0] > WIDTH ){ 00544 //If the missile is close enough to the destination, detonate 00545 if( abs( int(pDests[i][0] - pMissiles[i][0]) ) < PSPEED && abs( int(pDests[i][1] - pMissiles[i][1]) ) < PSPEED ){ 00546 pDetonations[i][0] = pDests[i][0]; 00547 pDetonations[i][1] = pDests[i][1]; 00548 pDetonations[i][2] = 0; //Start detonation at radius of 0 00549 pDetonations[i][3] = EXPAND; //Detonation is increasing in size 00550 //Otherwise, keep moving towards destination 00551 }else{ 00552 float dir = atan2(pDests[i][1]-pMissiles[i][1], pDests[i][0]-pMissiles[i][0]); 00553 pMissiles[i][0] += PSPEED * cos(dir); 00554 pMissiles[i][1] += PSPEED * sin(dir); 00555 } 00556 } 00557 } 00558 00559 //Enemy Missiles 00560 for(uint8_t i = 0; i < MAX_EMISSILES; i++){ 00561 //Check for a valid destination 00562 if( eDests[i] <= WIDTH ){ 00563 //If enemy missile is close enough to the destination, detonate 00564 if( abs( int((eDests[i]*14+6) - eMissiles[i][2]) ) < PSPEED && abs( int(HEIGHT-4 - eMissiles[i][3]) ) < PSPEED ){ 00565 cities[eDests[i]] = 0; //Destroy city/launcher 00566 00567 //If launcher, remove its ammo 00568 if( eDests[i] == 2 ){ 00569 pammo[0] = 0; 00570 } 00571 if( eDests[i] == 5 ){ 00572 pammo[1] = 0; 00573 } 00574 00575 eDests[i] = 127; //Reset enemy missile 00576 playSound(SOUND_DEAD); 00577 //Otherwise, keep moving towards destination 00578 }else{ 00579 float dir = atan2( HEIGHT-4 - eMissiles[i][3], (eDests[i]*14+6)-eMissiles[i][2] ); 00580 eMissiles[i][2] += espeed * cos(dir); 00581 eMissiles[i][3] += espeed * sin(dir); 00582 } 00583 } 00584 } 00585 } 00586 00587 void stepDetonations(){ 00588 if( counter%2 == 0 ){ 00589 for(uint8_t i = 0; i < MAX_PMISSILES; i++){ 00590 if( pDetonations[i][0] <= WIDTH ){ 00591 if( pDetonations[i][3] == EXPAND ){ //If detonation is expanding 00592 if( pDetonations[i][2] < PRADIUS ){ 00593 pDetonations[i][2]++; 00594 }else{ 00595 pDetonations[i][3] = SHRINK; //Start shrinking 00596 } 00597 } 00598 //Check this now instead of using else because it may have just 00599 //set to SHRINK; immediately checking will remove a delay at full 00600 //size. If a delay is desired, this if can be switched to an else. 00601 if( pDetonations[i][3] == SHRINK ){ //If detonation is shrinking 00602 if( pDetonations[i][2] > 0 ){ 00603 pDetonations[i][2]--; 00604 }else{ 00605 pDetonations[i][0] = 127; //Detonation is complete, remove it 00606 pDests[i][0] = 127; //Remove this destination 00607 } 00608 } 00609 } 00610 } 00611 } 00612 } 00613 00614 void stepCollision(){ 00615 //Player Detonations with Enemy Missiles 00616 for(uint8_t i = 0; i < MAX_PMISSILES; i++){ 00617 if( pDetonations[i][0] <= WIDTH ){ 00618 for(uint8_t j = 0; j < MAX_EMISSILES; j++){ 00619 //Add extra +1 to explosion radius so that collisions are more generous 00620 if( eDests[j] <= WIDTH && pDetonations[i][2] + 1 >= sqrt( (eMissiles[j][2]-pDetonations[i][0])*(eMissiles[j][2]-pDetonations[i][0]) + (eMissiles[j][3]-pDetonations[i][1])*(eMissiles[j][3]-pDetonations[i][1]) ) ){ 00621 eDests[j] = 127; //Remove enemy missile 00622 score+=25; 00623 playSound(SOUND_DETONATE); 00624 } 00625 } 00626 } 00627 } 00628 } 00629 00630 00631 void checkMenu(){ 00632 if( gb.buttons.pressed(BTN_C) ){ 00633 doTitle(1); 00634 } 00635 } 00636 00637 void checkWin(){ 00638 if( etotal == 0 ){ 00639 for(uint8_t i = 0; i < MAX_EMISSILES; i++){ 00640 //Check for a valid destination 00641 if( eDests[i] <= WIDTH ){ 00642 return; 00643 } 00644 } 00645 //If we get here, all enemy missiles are destroyed and they have no more 00646 nextLull(); 00647 mode = MODE_LULL; 00648 } 00649 } 00650 00651 void checkLose(){ 00652 for(uint8_t i = 0; i < 8; i++){ 00653 if( i != 2 && i != 5 ){ 00654 if( cities[i] ){ 00655 return; // A city remains alive 00656 } 00657 } 00658 } 00659 //If we get here, all cities are dead. It is the end of days. 00660 counter = 0; 00661 mode = MODE_DEAD; 00662 playSound(SOUND_LOSE); 00663 } 00664 00665 void stepGame(){ 00666 //Player input 00667 if( gb.buttons.pressed(BTN_A) ){ 00668 launchMissile(LAUNCHER_TWO); 00669 } 00670 if( gb.buttons.pressed(BTN_B) ){ 00671 launchMissile(LAUNCHER_ONE); 00672 } 00673 00674 if( gb.buttons.repeat(BTN_LEFT,1) ){ 00675 targetX = targetX-TARGET_SPEED > 0 ? targetX-TARGET_SPEED : 0; 00676 } 00677 if( gb.buttons.repeat(BTN_RIGHT,1) ){ 00678 targetX = targetX+TARGET_SPEED < WIDTH ? targetX+TARGET_SPEED : WIDTH; 00679 } 00680 if( gb.buttons.repeat(BTN_UP,1) ){ 00681 targetY = targetY-TARGET_SPEED > 0 ? targetY-TARGET_SPEED : 0; 00682 } 00683 if( gb.buttons.repeat(BTN_DOWN,1) ){ 00684 targetY = targetY+TARGET_SPEED < HEIGHT ? targetY+TARGET_SPEED : HEIGHT; 00685 } 00686 00687 //Game logic 00688 tryLaunchEnemy(); 00689 stepMissiles(); 00690 stepDetonations(); 00691 stepCollision(); 00692 00693 //Drawing 00694 drawScore(); 00695 drawTargets(); 00696 drawCities(); 00697 drawAmmo(); 00698 drawMissiles(); 00699 drawDetonations(); 00700 00701 checkWin(); 00702 checkLose(); 00703 } 00704 00705 void drawLull(){ 00706 uint32_t cityCount = 0; 00707 gb.display.cursorX = WIDTH/2 - TEXT_WIDTH*6; 00708 gb.display.cursorY = HEIGHT/2 - TEXT_HEIGHT*3; 00709 gb.display.print(("BONUS POINTS")); 00710 00711 gb.display.cursorX = WIDTH/2 - TEXT_WIDTH*8; 00712 gb.display.cursorY += TEXT_HEIGHT*2; 00713 gb.display.print(lullMissiles); 00714 00715 gb.display.setColor(3); 00716 for(uint8_t i = 0; i < lullMissiles; i++){ 00717 gb.display.drawPixel(WIDTH/2 - TEXT_WIDTH*6 + i*2,HEIGHT/2 - 3); 00718 } 00719 00720 gb.display.setColor(1); 00721 gb.display.cursorX = WIDTH/2 - TEXT_WIDTH*8; 00722 gb.display.cursorY += TEXT_HEIGHT*2; 00723 for( uint8_t i = 0; i < 8; i++ ){ 00724 if( lullCities[i] ) cityCount++; 00725 } 00726 gb.display.print(cityCount); 00727 00728 gb.display.setColor(4); 00729 for(uint8_t i = 0; i < cityCount; i++){ 00730 gb.display.drawBitmap(WIDTH/2 - TEXT_WIDTH*6 + i*9,HEIGHT/2+2, city); 00731 } 00732 00733 gb.display.setColor(1); 00734 drawScore(); 00735 drawCities(); 00736 drawAmmo(); 00737 } 00738 00739 void stepLull(){ 00740 00741 if( counter % 4 == 0 && (pammo[0] > 0 || pammo[1] > 0) ){ 00742 lullMissiles++; 00743 score+=10; 00744 if( pammo[0] > 0 ) pammo[0]--; 00745 else pammo[1]--; 00746 playSound(SOUND_SCORE); 00747 } 00748 00749 //If we have already iterated through the missiles 00750 if( counter % 8 == 0 && pammo[0] == 0 && pammo[1] == 0 ){ 00751 for( uint8_t i = 0; i < 9; i++ ){ 00752 if( i == 8 ){ //We have iterated through all live cities 00753 nextStage(); 00754 mode = MODE_GAME; 00755 return; 00756 } 00757 //If not a launcher and the city is alive 00758 if( i != 2 && i != 5 && cities[i] != 0 ){ 00759 lullCities[i] = 1; 00760 score+=100; 00761 cities[i] = 0; 00762 playSound(SOUND_SCORE); 00763 break; 00764 } 00765 } 00766 } 00767 00768 drawLull(); 00769 } 00770 00771 void stepDead(){ 00772 gb.display.setColor(7); 00773 gb.display.cursorX = WIDTH/2 - TEXT_WIDTH*3 - TEXT_WIDTH/2;; 00774 gb.display.cursorY = HEIGHT/2 - TEXT_HEIGHT; 00775 gb.display.print(("THE END")); 00776 gb.display.setColor(1); 00777 00778 if( mode == MODE_DEAD && counter%20 == 0 ){ 00779 mode = MODE_POSTDEAD; 00780 }else if( mode == MODE_POSTDEAD ){ 00781 if( counter%8 == 0 ){ 00782 flash ^= 255; 00783 } 00784 if( flash ){ 00785 gb.display.setColor(4); 00786 gb.display.cursorX = WIDTH/2 - TEXT_WIDTH*3 - TEXT_WIDTH/2; 00787 gb.display.cursorY = HEIGHT - TEXT_HEIGHT*2; 00788 gb.display.print(("PRESS \25")); 00789 00790 if( isHighscore(score) ){ 00791 gb.display.cursorX = WIDTH/2 - TEXT_WIDTH*6 - TEXT_WIDTH/2; 00792 gb.display.cursorY = HEIGHT - TEXT_HEIGHT*3; 00793 gb.display.print(("NEW HIGHSCORE")); 00794 } 00795 gb.display.setColor(1); 00796 } 00797 00798 if( gb.buttons.pressed(BTN_A) ){ 00799 if( isHighscore(score) ){ 00800 char tmp_name[11] = "User"; 00801 //gb.getDefaultName(tmp_name); 00802 gb.keyboard(tmp_name, 11); 00803 saveHighscore(score,tmp_name); 00804 } 00805 score = 0; 00806 mode = MODE_PREGAME; 00807 } 00808 } 00809 } 00810 00811 void stepPregame(){ 00812 drawHighscores(); 00813 00814 if( counter%8 == 0 ){ 00815 flash ^= 255; 00816 } 00817 if( flash ){ 00818 gb.display.setColor(4); 00819 gb.display.cursorX = WIDTH/2 - TEXT_WIDTH*3; 00820 gb.display.cursorY = HEIGHT - TEXT_HEIGHT*2; 00821 gb.display.print(("PRESS \25")); 00822 gb.display.setColor(1); 00823 } 00824 00825 if( gb.buttons.pressed(BTN_A) ){ 00826 stage = 255; 00827 for( uint8_t i = 0; i < 8; i++ ){ 00828 lullCities[i] = 1; 00829 } 00830 nextStage(); //Reset to stage 0 00831 mode = MODE_GAME; 00832 } 00833 } 00834 00835 void loop() { 00836 if(gb.update()){ 00837 00838 switch( mode ){ 00839 case MODE_GAME: 00840 stepGame(); 00841 break; 00842 00843 case MODE_LULL: 00844 stepLull(); 00845 break; 00846 00847 case MODE_DEAD: 00848 case MODE_POSTDEAD: 00849 stepDead(); 00850 break; 00851 00852 case MODE_PREGAME: 00853 stepPregame(); 00854 break; 00855 } 00856 00857 checkMenu(); 00858 00859 counter++; 00860 } 00861 } 00862 00863 00864 void loadHighscores(){ 00865 00866 uint32_t defdata[] = {10000,7000,5000,4000,2000}; 00867 char defnames[NUM_HIGHSCORES][NAME_SIZE+1] = {"Crack Shot", "Defender", "Gunner", "We Tried", "No Hope"}; 00868 // Initialize the cookie 00869 gamedata.begin("ArmageDD",gamedata); 00870 00871 uint32_t allscores=0; 00872 for (int i=0;i<NUM_HIGHSCORES;i++) allscores += gamedata.highscores[i]; 00873 00874 if (gamedata.check_number != 12345) { 00875 //checknumber indicates that no highscores saved yet, so put in "defaults" 00876 for (int i=0;i<NUM_HIGHSCORES;i++) gamedata.highscores[i]=defdata[i]; 00877 for (int i=0;i<NUM_HIGHSCORES;i++) strcpy(gamedata.names[i],defnames[i]); 00878 gamedata.check_number = 12345; // put the checking number 00879 gamedata.saveCookie();//save the default values 00880 } 00881 /* 00882 for( uint8_t entry = 0; entry < NUM_HIGHSCORES; entry++ ){ 00883 for( uint8_t offset = 0; offset < ENTRY_SIZE; offset++ ){ 00884 if( offset < NAME_SIZE ){ 00885 names[entry][offset] = EEPROM.read( ENTRY_SIZE * entry + offset ); 00886 }else{ 00887 uint8_t* addr = (uint8_t*) &highscores[entry]; 00888 addr+=offset-NAME_SIZE; 00889 *addr = EEPROM.read( ENTRY_SIZE * entry + offset ); 00890 } 00891 } 00892 } 00893 */ 00894 00895 } 00896 00897 uint8_t isHighscore(uint32_t score){ 00898 if( score > gamedata.highscores[NUM_HIGHSCORES-1] ){ 00899 return 1; 00900 } 00901 return 0; 00902 } 00903 00904 void saveHighscore(uint32_t score, char* who){ 00905 uint8_t found = 0; 00906 uint32_t tmp_score = 0; 00907 char tmp_name[10]; 00908 for( uint8_t entry = 0; entry < NUM_HIGHSCORES; entry++ ){ 00909 if( score > gamedata.highscores[entry] ){ 00910 found = 1; 00911 } 00912 if( found ){ 00913 tmp_score = gamedata.highscores[entry]; 00914 strcpy(tmp_name,gamedata.names[entry]); 00915 00916 gamedata.highscores[entry] = score; 00917 strcpy(gamedata.names[entry], who); 00918 00919 score = tmp_score; 00920 strcpy(who, tmp_name); 00921 } 00922 } 00923 gamedata.saveCookie(); 00924 /*Writing to EEPROM directly is deprecated and not necessary 00925 for( uint8_t entry = 0; entry < NUM_HIGHSCORES; entry++ ){ 00926 for( uint8_t offset = 0; offset < ENTRY_SIZE; offset++ ){ 00927 if( offset < NAME_SIZE ){ 00928 EEPROM.write( ENTRY_SIZE * entry + offset, names[entry][offset] ); 00929 }else{ 00930 uint8_t* addr = (uint8_t*) &highscores[entry]; 00931 addr+=offset-NAME_SIZE; 00932 EEPROM.write( ENTRY_SIZE * entry + offset, *addr ); 00933 } 00934 } 00935 } 00936 */ 00937 } 00938 00939 void drawHighscores(){ 00940 gb.display.setColor(4); 00941 gb.display.cursorX = WIDTH/2 - TEXT_WIDTH*5; 00942 gb.display.cursorY = TEXT_HEIGHT; 00943 gb.display.print(("HIGHSCORES")); 00944 gb.display.setColor(1); 00945 00946 for( uint8_t entry = 0; entry < NUM_HIGHSCORES; entry++ ){ 00947 gb.display.cursorX = 0; 00948 gb.display.cursorY = TEXT_HEIGHT*3 + TEXT_HEIGHT*entry; 00949 gb.display.print(gamedata.names[entry]); 00950 gb.display.cursorX = WIDTH - TEXT_WIDTH*6; 00951 if( gamedata.highscores[entry] < 100000 ){ 00952 gb.display.print(0); 00953 } 00954 if( gamedata.highscores[entry] < 10000 ){ 00955 gb.display.print(0); 00956 } 00957 if( gamedata.highscores[entry] < 1000 ){ 00958 gb.display.print(0); 00959 } 00960 if( gamedata.highscores[entry] < 100 ){ 00961 gb.display.print(0); 00962 } 00963 if( gamedata.highscores[entry] < 10 ){ 00964 gb.display.print(0); 00965 } 00966 gb.display.print(gamedata.highscores[entry]); 00967 } 00968 } 00969 00970 // Wave, pitch, duration, arpeggio step duration, arpeggio step size 00971 const int8_t sounds[][5] = { 00972 { 0, 20, 5, 1, 1 }, //Player launch 00973 { 0, 25, 5, 1, -1 }, //Enemy launch 00974 { 1, 10, 5, 1, -1 }, //Detonating enemy missile 00975 { 1, 10, 2, 0, 0 }, //Score pips 00976 { 1, 2, 10, 1, -1 }, //A city dies 00977 { 0, 20, 14, 3, -1 }, //Lose 00978 }; 00979 00980 void initSound(){ 00981 gb.sound.command(CMD_VOLUME, 5, 0, 0); 00982 gb.sound.command(CMD_SLIDE, 0, 0, 0); 00983 } 00984 00985 void playSound(uint8_t i){ 00986 gb.sound.command(CMD_VOLUME, 5, 0, 0); 00987 gb.sound.command(CMD_SLIDE, 0, 0, 0); 00988 gb.sound.command(CMD_ARPEGGIO, sounds[i][3], sounds[i][4], 0); 00989 gb.sound.command(CMD_INSTRUMENT, sounds[i][0], 0, 0); 00990 gb.sound.playNote(sounds[i][1], sounds[i][2], 0); 00991 00992 //gb.sound.playNote(20, 5, 0); 00993 } 00994 00995
Generated on Sun Jul 17 2022 01:52:57 by
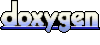