PixArt Optical Track Sensor, OTS, library initial release v1.0. Supports PAT9125, PAT9126, PAT9130, PAA5101. Future to support PAT9150.
Fork of Pixart_OTS by
Pixart_OTS_InitSetting.h
00001 /* PixArt Optical Finger Navigation, OFN, sensor driver. 00002 * By PixArt Imaging Inc. 00003 * Primary Engineer: Hill Chen (PixArt USA) 00004 * 00005 * License: Apache-2.0; http://www.apache.org/licenses/LICENSE-2.0 00006 */ 00007 00008 #pragma once 00009 #include <stdint.h> 00010 00011 static const uint8_t Pixart_OTS_9125_InitSetting[][2] = 00012 { 00013 { 0x7F, 0x00 }, //Switch to bank0 00014 { 0x09, 0x5A }, //Disables write-protect. 00015 { 0x0D, 0x65 }, //Sets X-axis resolution (necessary value here will depend on physical design and application). 00016 { 0x0E, 0xFF }, //Sets Y-axis resolution (necessary value here will depend on physical design and application). 00017 { 0x19, 0x04 }, //Sets 12-bit X/Y data format. 00018 //{ 0x4B, 0x04 }, //Needed for when using low-voltage rail (1.7-1.9V): Power saving configuration. 00019 { 0x09, 0x00 }, //Enable write protect 00020 }; 00021 00022 static const uint8_t Pixart_OTS_9126_InitSetting[][2] = 00023 { 00024 { 0x09, 0x5A }, //Disables write-protect. 00025 { 0x0D, 0x65 }, //Sets X-axis resolution (necessary value here will depend on physical design and application). 00026 //{ 0x0E, 0x00 }, //Sets Y-axis resolution (necessary value here will depend on physical design and application). 00027 { 0x0E, 0x05 }, //Sets Y-axis resolution to a small number for demo, set 0 to avoid Y count. 00028 { 0x19, 0x04 }, //Sets 12-bit X/Y data format. 00029 //{ 0x4B, 0x04 }, //Needed for when using low-voltage rail (1.7-1.9V): Power saving configuration. 00030 { 0x7C, 0x82 }, 00031 { 0x2B, 0x6D }, 00032 { 0x2D, 0x00 }, 00033 { 0x09, 0x00 }, //Enable write protect 00034 }; 00035 00036 static const uint8_t Pixart_OTS_9150_InitSetting[][2] = 00037 { 00038 { 0x7F, 0x00 }, //Switch to bank0 00039 { 0x09, 0x5A }, //Disables write-protect. 00040 { 0x0A, 0x0F }, // set X-axis resolution (depends on application) 00041 { 0x09, 0x00 }, //Enable write protect 00042 }; 00043 00044 static const uint8_t Pixart_OTS_9130_InitSetting[][2] = 00045 { 00046 { 0x7F, 0x00 }, // switch to bank0, not allowed to perform SPIWriteRead 00047 { 0x05, 0xA8 }, // sleep mode disabled 00048 { 0x09, 0x5A }, // disable write protect 00049 { 0x51, 0x06 }, // set LD current source to 6 00050 { 0x0D, 0x1F }, // CPI resolution setting for X-direction 00051 { 0x0E, 0x1F }, // CPI resolution setting for Y-direction 00052 { 0x07, 0x00 }, 00053 { 0x1B, 0x42 }, 00054 { 0x2E, 0x40 }, 00055 { 0x32, 0x40 }, 00056 { 0x33, 0x02 }, 00057 { 0x34, 0x00 }, 00058 { 0x36, 0xE0 }, 00059 { 0x38, 0xA0 }, 00060 { 0x39, 0x01 }, 00061 { 0x3E, 0x14 }, 00062 { 0x44, 0x02 }, 00063 { 0x4A, 0xE0 }, 00064 { 0x4F, 0x02 }, 00065 { 0x52, 0x0D }, // Turn off internal VDDA 00066 { 0x57, 0x03 }, 00067 { 0x59, 0x03 }, 00068 { 0x5B, 0x03 }, 00069 { 0x5C, 0xFF }, 00070 00071 { 0x7F, 0x01 }, // switch to bank1, not allowed to perform SPIWriteRead 00072 { 0x00, 0x25 }, 00073 { 0x07, 0x78 }, 00074 { 0x20, 0x00 }, 00075 { 0x21, 0x40 }, 00076 { 0x23, 0x00 }, 00077 { 0x2F, 0x64 }, 00078 { 0x37, 0x30 }, 00079 { 0x3B, 0x64 }, 00080 { 0x43, 0x0A }, 00081 { 0x59, 0x01 }, 00082 { 0x5A, 0x01 }, 00083 { 0x5C, 0x04 }, 00084 { 0x5E, 0x04 }, 00085 00086 { 0x7F, 0x02 }, // switch to bank2, not allowed to perform SPIWriteRead 00087 { 0x51, 0x02 }, 00088 00089 { 0x7F, 0x03 }, // switch to bank3, not allowed to perform SPIWriteRead 00090 { 0x05, 0x0C }, 00091 { 0x06, 0x0C }, 00092 { 0x07, 0x0C }, 00093 { 0x08, 0x0C }, 00094 00095 { 0x7F, 0x04 }, // switch to bank4, not allowed to perform SPIWriteRead 00096 { 0x05, 0x01 }, 00097 { 0x53, 0x08 }, 00098 00099 { 0x7F, 0x05 }, // switch to bank5, not allowed to perform SPIWriteRead 00100 { 0x00, 0x02 }, 00101 { 0x09, 0x01 }, 00102 { 0x0A, 0x1C }, 00103 { 0x0B, 0x24 }, 00104 { 0x0C, 0x1C }, 00105 { 0x0D, 0x24 }, 00106 { 0x12, 0x08 }, 00107 { 0x14, 0x02 }, 00108 { 0x16, 0x02 }, 00109 { 0x18, 0x1C }, 00110 { 0x19, 0x24 }, 00111 { 0x1A, 0x1C }, 00112 { 0x1B, 0x24 }, 00113 { 0x20, 0x08 }, 00114 { 0x22, 0x02 }, 00115 { 0x24, 0x02 }, 00116 { 0x26, 0x88 }, 00117 { 0x2F, 0x7C }, 00118 { 0x30, 0x07 }, 00119 { 0x3D, 0x00 }, 00120 { 0x3E, 0x98 }, 00121 00122 { 0x7F, 0x06 }, // switch to bank6, not allowed to perform SPIWriteRead 00123 { 0x34, 0x03 }, 00124 00125 { 0x7F, 0x07 }, // switch to bank7, not allowed to perform SPIWriteRead 00126 { 0x00, 0x01 }, 00127 { 0x02, 0xC4 }, 00128 { 0x03, 0x13 }, 00129 { 0x06, 0x0C }, 00130 { 0x0F, 0x0A }, 00131 { 0x14, 0x02 }, 00132 { 0x35, 0x39 }, 00133 { 0x36, 0x3F }, 00134 { 0x46, 0x03 }, 00135 { 0x47, 0x0F }, 00136 { 0x4B, 0x97 }, 00137 00138 { 0x7F, 0x00 }, // switch to bank0, not allowed to perform SPIWriteRead 00139 { 0x09, 0x00 }, // enable write protect 00140 }; 00141 00142 static const uint8_t Pixart_OTS_5101_InitSetting[][2] = 00143 { 00144 { 0x09,0x5A }, // disable write protect 00145 { 0x51,0x06 }, // To set LD power first, power should be <= 6 00146 { 0x7F,0x00 }, // Bank0, not allowed to perform SPIWriteRead 00147 { 0x05,0xA8 }, 00148 { 0x07,0xCC }, 00149 { 0x0A,0x17 }, 00150 { 0x0D,0x05 }, 00151 { 0x0E,0x05 }, 00152 { 0x1B,0x43 }, 00153 { 0x25,0x2E }, 00154 { 0x26,0x35 }, 00155 { 0x2E,0x40 }, 00156 { 0x32,0x40 }, 00157 { 0x33,0x02 }, 00158 { 0x34,0x00 }, 00159 { 0x36,0xE0 }, 00160 { 0x3E,0x14 }, 00161 { 0x44,0x02 }, 00162 { 0x51,0x06 }, 00163 { 0x52,0x0C }, 00164 { 0x57,0x05 }, 00165 { 0x59,0x03 }, 00166 { 0x5B,0x04 }, 00167 { 0x5D,0x3B }, 00168 { 0x7C,0xC8 }, 00169 00170 { 0x7F,0x01 }, // Bank1, not allowed to perform SPIWriteRead 00171 { 0x00,0x2F }, 00172 { 0x08,0x1C }, 00173 { 0x0A,0x02 }, 00174 { 0x19,0x40 }, 00175 { 0x1B,0x10 }, 00176 { 0x1D,0x18 }, 00177 { 0x1F,0x12 }, 00178 { 0x20,0x00 }, 00179 { 0x21,0x80 }, 00180 { 0x23,0x60 }, 00181 { 0x25,0x64 }, 00182 { 0x27,0x64 }, 00183 { 0x2B,0x78 }, 00184 { 0x2F,0x78 }, 00185 { 0x39,0x78 }, 00186 { 0x3B,0x78 }, 00187 { 0x3D,0x78 }, 00188 { 0x3F,0x78 }, 00189 { 0x44,0x7E }, 00190 { 0x45,0xF4 }, 00191 { 0x46,0x01 }, 00192 { 0x47,0x2C }, 00193 { 0x49,0x90 }, 00194 { 0x4A,0x05 }, 00195 { 0x4B,0xDC }, 00196 { 0x4C,0x07 }, 00197 { 0x4D,0x08 }, 00198 { 0x51,0x02 }, 00199 { 0x52,0xBC }, 00200 { 0x53,0x02 }, 00201 { 0x54,0xBC }, 00202 { 0x55,0x07 }, 00203 { 0x56,0x08 }, 00204 { 0x57,0x07 }, 00205 { 0x58,0x08 }, 00206 { 0x59,0x08 }, 00207 { 0x5A,0x08 }, 00208 00209 { 0x7F,0x02 }, // Bank2, not allowed to perform SPIWriteRead 00210 { 0x07,0x1B }, 00211 { 0x08,0x1F }, 00212 { 0x09,0x23 }, 00213 { 0x51,0x01 }, 00214 00215 { 0x7F,0x03 }, // Bank3, not allowed to perform SPIWriteRead 00216 { 0x07,0x07 }, 00217 { 0x08,0x06 }, 00218 { 0x2F,0x00 }, 00219 { 0x30,0x20 }, 00220 { 0x32,0x59 }, 00221 { 0x33,0xD8 }, 00222 { 0x34,0x4E }, 00223 { 0x35,0x20 }, 00224 { 0x36,0x5B }, 00225 { 0x37,0xCC }, 00226 { 0x38,0x50 }, 00227 { 0x39,0x14 }, 00228 00229 { 0x7F,0x04 }, // Bank4, not allowed to perform SPIWriteRead 00230 { 0x05,0x01 }, 00231 { 0x2C,0x06 }, 00232 { 0x2E,0x0C }, 00233 { 0x30,0x0C }, 00234 { 0x32,0x06 }, 00235 { 0x34,0x03 }, 00236 { 0x38,0x17 }, 00237 { 0x39,0x71 }, 00238 { 0x3A,0x18 }, 00239 { 0x3B,0x4D }, 00240 { 0x3C,0x18 }, 00241 { 0x3D,0x4D }, 00242 { 0x3E,0x14 }, 00243 { 0x3F,0xD1 }, 00244 { 0x40,0x14 }, 00245 { 0x41,0xDD }, 00246 { 0x42,0x0A }, 00247 { 0x43,0x6C }, 00248 { 0x44,0x08 }, 00249 { 0x45,0xAD }, 00250 { 0x46,0x06 }, 00251 { 0x47,0xF2 }, 00252 { 0x48,0x06 }, 00253 { 0x49,0xEC }, 00254 { 0x4A,0x06 }, 00255 { 0x4B,0xEC }, 00256 { 0x53,0x08 }, 00257 00258 { 0x7F,0x05 }, // Bank5, not allowed to perform SPIWriteRead 00259 { 0x03,0x00 }, 00260 { 0x09,0x01 }, 00261 { 0x0B,0xFF }, 00262 { 0x0D,0xFF }, 00263 { 0x0F,0xFF }, 00264 { 0x11,0xFF }, 00265 { 0x12,0xD2 }, 00266 { 0x13,0xD2 }, 00267 { 0x19,0xFF }, 00268 { 0x1B,0xFF }, 00269 { 0x1D,0xFF }, 00270 { 0x1F,0xFF }, 00271 { 0x20,0xD2 }, 00272 { 0x21,0xD2 }, 00273 { 0x2F,0x7C }, 00274 { 0x30,0x05 }, 00275 { 0x41,0x02 }, 00276 { 0x53,0xFF }, 00277 { 0x5F,0x02 }, 00278 00279 { 0x7F,0x06 }, // Bank6, not allowed to perform SPIWriteRead 00280 { 0x2A,0x05 }, // Write ONLY address, not allowed to perform SPIWriteRead 00281 { 0x35,0x19 }, 00282 00283 { 0x7F,0x07 }, // Bank7, not allowed to perform SPIWriteRead 00284 { 0x00,0x01 }, 00285 { 0x14,0x03 }, 00286 { 0x15,0x14 }, 00287 { 0x46,0x03 }, 00288 00289 { 0x7F,0x00 }, // Bank0, not allowed to perform SPIWriteRead 00290 };
Generated on Thu Jul 14 2022 20:52:37 by
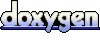