PixArt Optical Track Sensor, OTS, library initial release v1.0. Supports PAT9125, PAT9126, PAT9130, PAA5101. Future to support PAT9150.
Fork of Pixart_OTS by
Pixart_OTS_GrabData.cpp
00001 /* PixArt Optical Finger Navigation, OFN, sensor driver. 00002 * By PixArt Imaging Inc. 00003 * Primary Engineer: Hill Chen (PixArt USA) 00004 * 00005 * License: Apache-2.0; http://www.apache.org/licenses/LICENSE-2.0 00006 */ 00007 00008 #include "Pixart_OTS_GrabData.h" 00009 00010 Pixart_OTS_OtsData Pixart_OTS_GrabData_12bitXy::grab(Pixart_ComPort &com_port) 00011 { 00012 int16_t deltaX_low = com_port.readRegister(0x03); 00013 int16_t deltaY_low = com_port.readRegister(0x04); 00014 int16_t deltaX_high = 0, deltaY_high = 0; 00015 00016 int16_t deltaXY_h = com_port.readRegister(0x12); 00017 deltaX_high = (deltaXY_h << 4) & 0xF00; 00018 deltaY_high = (deltaXY_h << 8) & 0xF00; 00019 00020 if (deltaX_high & 0x800) 00021 deltaX_high |= 0xf000; 00022 if (deltaY_high & 0x800) 00023 deltaY_high |= 0xf000; 00024 00025 Pixart_OTS_OtsData otsData; 00026 otsData.x = deltaX_high | deltaX_low; 00027 otsData.y = deltaY_high | deltaY_low; 00028 return otsData; 00029 } 00030 00031 Pixart_OTS_OtsData Pixart_OTS_GrabData_16bitXy::grab(Pixart_ComPort &com_port) 00032 { 00033 int16_t deltaX_low = com_port.readRegister(0x03); 00034 int16_t deltaY_low = com_port.readRegister(0x04); 00035 int16_t deltaX_high = 0, deltaY_high = 0; 00036 00037 deltaX_high = com_port.readRegister(0x11) << 8; 00038 deltaY_high = com_port.readRegister(0x12) << 8; 00039 00040 Pixart_OTS_OtsData otsData; 00041 otsData.x = deltaX_high | deltaX_low; 00042 otsData.y = deltaY_high | deltaY_low; 00043 return otsData; 00044 } 00045 00046 Pixart_OTS_OtsData Pixart_OTS_GrabData_16bitXOnly::grab(Pixart_ComPort &com_port) 00047 { 00048 int16_t deltaX_low = com_port.readRegister(0x03); 00049 int16_t deltaX_high = 0; 00050 deltaX_high = com_port.readRegister(0x04) << 8; 00051 00052 Pixart_OTS_OtsData otsData; 00053 otsData.x = deltaX_high | deltaX_low; 00054 otsData.y = 0; 00055 return otsData; 00056 }
Generated on Thu Jul 14 2022 20:52:37 by
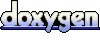