
PMT9123 OTS on Nucleo (Initial Release)
Dependencies: Pixart_9123_Nucleo_Library mbed
Fork of PMT9123_OTS_NUCLEO_Program by
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "OTC.h" 00019 #include <string> 00020 #include <ctype.h> 00021 using namespace std; 00022 00023 // Function prototypes 00024 void serialEvent(); 00025 void serialProcess(string input); 00026 bool IsParamValid(char *param, bool hex = true); 00027 bool IsDigit(char *p, bool hex); 00028 void Hex8(uint8_t data); 00029 void PrintHex8(uint8_t data); 00030 00031 Serial pc(USBTX,USBRX);//tx, rx 00032 I2C i2c(I2C_SDA, I2C_SCL); 00033 00034 DigitalOut led1(LED1); 00035 DigitalOut pwm(D9); 00036 PwmOut mypwm(D9); 00037 00038 // OTS object declaration 00039 Pixart_OTS *m_OTS = NULL; 00040 00041 // Serial string 00042 string strRead = ""; 00043 00044 // LED PWM setting 00045 #define LED_PERIOD_US 3000 // 3ms 00046 #define LED_MAX_DC 100 00047 // LED brightness control value in us 00048 int16_t LED_on_time_us = 0; 00049 int16_t Counts; 00050 int16_t max_on_period; 00051 00052 // LED control 00053 void LED_control(int16_t value) 00054 { 00055 int16_t led_on_time; 00056 00057 LED_on_time_us += value; 00058 led_on_time = LED_on_time_us; 00059 00060 if (LED_on_time_us < 5) // hysterisis so that the LED stay off 00061 led_on_time = 0; 00062 else if (LED_on_time_us > max_on_period) 00063 led_on_time = LED_on_time_us = max_on_period; 00064 00065 mypwm.pulsewidth_us(led_on_time); 00066 } 00067 00068 // Reset count and LED 00069 void ClearCount() 00070 { 00071 Counts = 0; 00072 pc.printf("Count = 0\n"); 00073 LED_on_time_us = 0; 00074 LED_control(0); 00075 } 00076 00077 void GetData(int16_t value) 00078 { 00079 Counts += value; 00080 pc.printf("Count = %d\n", Counts); 00081 00082 // Change brightness 00083 LED_control(value); 00084 } 00085 00086 // main() runs in its own thread in the OS 00087 // (note the calls to Thread::wait below for delays) 00088 int main() { 00089 00090 pc.format(8, Serial::None, 1); 00091 pc.baud(115200); 00092 pc.attach(&serialEvent); 00093 i2c.frequency(400000); 00094 00095 // LED control 00096 max_on_period = LED_MAX_DC*LED_PERIOD_US/100; 00097 mypwm.period_us(LED_PERIOD_US); 00098 LED_on_time_us = 0; // start with 0, which is off 00099 LED_control(0); 00100 00101 Counts = 0; // restart count to zero 00102 00103 bool Result = false; 00104 // Set to polling at 4ms 00105 m_OTS = new Pixart_OTS(&i2c,4,GetData,Result); 00106 00107 if(Result == true) 00108 { 00109 pc.printf("Initial Pixart OTS successful\n\r"); 00110 } 00111 else 00112 { 00113 pc.printf("Initial Pixart OTS fail\n\r"); 00114 } 00115 00116 00117 while (true) 00118 { 00119 ; 00120 } 00121 } 00122 00123 void serialEvent() { 00124 char inChar; 00125 00126 while (pc.readable()) 00127 { 00128 // get the new byte: 00129 inChar = (char)pc.getc(); 00130 //pc.putc(inChar); 00131 // if the incoming character is a newline, set a flag 00132 // so the main loop can do something about it: 00133 if ((inChar == '\n') || (inChar == '\r')) 00134 { 00135 //strRead.trim(); 00136 strRead += " "; // for tokenizing 00137 serialProcess(strRead); 00138 strRead = ""; 00139 } 00140 else if (inChar != 0xf0) 00141 // add it to the strRead 00142 strRead += inChar; 00143 } 00144 } 00145 00146 // Macro define for easily reading 00147 #define IsEqual(a,b) (!strcmp((a),(b))) 00148 00149 void serialProcess(string input) 00150 { 00151 int val; 00152 unsigned char Address,Value; 00153 00154 //pc.printf("cmd = %s\n", input); 00155 // ==================================================== 00156 // Single command goes here 00157 // NOTE: All command compare must have " " at the back 00158 // ==================================================== 00159 if (input == "c ") 00160 { 00161 ClearCount(); 00162 } 00163 // ==================================================== 00164 // Command with parameters goes here, like r 00, w 48 00 00165 // ==================================================== 00166 else if (input != " ") // not empty string, then proceed to decode the command 00167 { 00168 char buff[40]; 00169 size_t l = input.copy(buff, input.length(), 0); 00170 buff[l] = '\0'; 00171 00172 // Get the command 00173 char *tok = strtok(buff, " "); 00174 if (!tok) 00175 { 00176 pc.printf("empty command\n"); 00177 return; 00178 } 00179 00180 // Get parameters, up to 4 parameters, if more than that, individual command will need to extract it on their own 00181 char *param[4]; 00182 for (val = 0; val < 4; val++) 00183 { 00184 param[val] = strtok(NULL, " "); 00185 if (!param[val]) // break if we reach the end 00186 break; 00187 } 00188 00189 // convert the first parameter into decimal first, as it is most commonly used 00190 val = strtol(param[0], NULL, 10); 00191 00192 // switch for command 00193 if ((IsEqual(tok,"r")) || (IsEqual(tok,"w"))) 00194 { 00195 // Address is the first byte 00196 if (!IsParamValid(param[0])) 00197 { 00198 return; 00199 } 00200 // convert to integer 00201 Address = strtol(param[0], NULL, 16); 00202 if (Address > 0x7F) 00203 { 00204 pc.printf("Error (r/w): address > 0x7F\n"); 00205 return; 00206 } 00207 00208 if ((IsEqual(tok,"w"))) 00209 { 00210 // Value is second byte 00211 if (!IsParamValid(param[1])) 00212 { 00213 return; 00214 } 00215 // convert to integer 00216 Value = strtol(param[1], NULL, 16); 00217 if (Value > 0xFF) 00218 { 00219 pc.printf("Error (w): value > 0xFF\n"); 00220 return; 00221 } 00222 00223 val = m_OTS->Register_Write(Address, Value); 00224 } 00225 else 00226 { 00227 val = m_OTS->Register_Read(Address); 00228 } 00229 00230 pc.printf("ADDR %02X %02X\n", Address, val); 00231 } 00232 else 00233 { 00234 pc.printf("Bad command : "); 00235 pc.printf("%s\n", tok); 00236 } 00237 } 00238 } 00239 00240 // Check if param is not given or not hexa, print the error as well 00241 bool IsParamValid(char *param, bool hex) 00242 { 00243 if (!param) 00244 { 00245 return false; 00246 } 00247 if (!IsDigit(param, hex)) 00248 { 00249 pc.printf("Error : arg invalid\n"); 00250 return false; 00251 } 00252 return true; 00253 } 00254 00255 // Check whether is hexa for given char 00256 bool IsDigit(char *p, bool hex) 00257 { 00258 for (int i; i < strlen(p); i++) 00259 { 00260 if (hex) 00261 { 00262 if (!isxdigit(p[i])) 00263 return false; 00264 } 00265 else if (!isdigit(p[i])) 00266 return false; 00267 } 00268 return true; 00269 } 00270
Generated on Tue Jul 12 2022 16:33:31 by
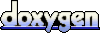