
Initial Release of Program for PAT9125 OTS on L476RG Platform
Dependencies: mbed
Fork of PAT9125_OTS_L476RG by
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "pixart_lcm.h" 00019 #include "pat9125_i2c.h" 00020 #include "pat9125_mbed.h" 00021 00022 pat9125_mbed_state_s g_pat9125_mbed_state ; 00023 pat9125_mbed *gp_pat9125_mbed ; 00024 pixart_lcm *gp_pixart_lcm = NULL; 00025 00026 SPI spi(SPI_MOSI, SPI_MISO, SPI_SCK); 00027 Serial pc(USBTX, USBRX); 00028 00029 #define I2C_SDA_PIN I2C_SDA 00030 #define I2C_SCL_PIN I2C_SCL 00031 //DigitalIn sdaDummy(I2C_SDA_PIN, PullUp); 00032 //DigitalIn sclDummy(I2C_SCL_PIN, PullUp); 00033 pat9125_i2c *gp_pat9125_i2c;//(I2C_SDA0, I2C_SCL0); 00034 00035 00036 DigitalOut PIN_LCM_CSB(PB_6); 00037 DigitalOut PIN_LCM_RSTB(PA_9); 00038 DigitalOut PIN_LCM_RS(PC_7); 00039 00040 InterruptIn PIN_SEN_MOTION(PB_3); 00041 DigitalIn motionDummy(PB_3, PullUp); 00042 00043 DigitalIn PIN_BTN_L(PA_0); 00044 DigitalIn BTN_L_Dummy(PA_0, PullUp); 00045 DigitalIn PIN_BTN_R(PA_1); 00046 DigitalIn BTN_R_Dummy(PA_1, PullUp); 00047 00048 DigitalOut PIN_GLED(PA_10); 00049 DigitalOut PIN_RLED(PB_4); 00050 #define I2C_ADDRESS 0x73 00051 00052 //----------------------------------------------------------------------- 00053 int main(void) 00054 { 00055 char addr = 0; 00056 char data ; 00057 // pc.set_flow_control(SerialBase::Disabled) ; 00058 pc.baud(115200); 00059 pc.printf("---------- Pixart PAT9125 Demo\n"); 00060 00061 // +++++++ LCM Initialization +++++++ // 00062 // Chip must be deselected 00063 PIN_LCM_CSB = 1 ; 00064 00065 // Setup the spi for 8 bit data, high steady state clock, 00066 // second edge capture, with a 1MHz clock rate 00067 spi.format(8,3); 00068 spi.frequency(1000000); 00069 gp_pixart_lcm = new pixart_lcm(&spi, &PIN_LCM_CSB, &PIN_LCM_RSTB, &PIN_LCM_RS) ; 00070 // ------- LCM Initialization ------- // 00071 00072 // +++++++ PAT9125 Initialization +++++++ // 00073 gp_pat9125_i2c = new pat9125_i2c(I2C_SDA_PIN, I2C_SCL_PIN); 00074 gp_pat9125_i2c->frequency(400000); 00075 g_pat9125_mbed_state.p_i2c = gp_pat9125_i2c; 00076 g_pat9125_mbed_state.p_pc = &pc; 00077 g_pat9125_mbed_state.pBTN_L = &PIN_BTN_L ; 00078 g_pat9125_mbed_state.pBTN_R = &PIN_BTN_R ; 00079 g_pat9125_mbed_state.pINT = &PIN_SEN_MOTION; 00080 g_pat9125_mbed_state.pRLED = &PIN_RLED; 00081 g_pat9125_mbed_state.pGLED = &PIN_GLED; 00082 g_pat9125_mbed_state.pLCM = gp_pixart_lcm; 00083 g_pat9125_mbed_state.slave_id = (I2C_ADDRESS << 1); 00084 g_pat9125_mbed_state.sen_status = 0; 00085 00086 gp_pat9125_mbed = new pat9125_mbed(&g_pat9125_mbed_state) ; 00087 gp_pixart_lcm->LCM_DisplayString_Boot(g_pat9125_mbed_state.sen_status); 00088 00089 if(g_pat9125_mbed_state.sen_status == true) 00090 { 00091 pc.printf("Initial Sensor ... Done\n"); 00092 } 00093 else 00094 { 00095 pc.printf("Initial Sensor ... Fail\n"); 00096 } 00097 while(true) 00098 { 00099 gp_pat9125_mbed->task(); 00100 } 00101 }
Generated on Thu Jul 14 2022 03:30:10 by
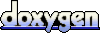