
fuck this
Dependencies: BMP280
Logging.h
00001 #ifndef __Logging__ 00002 #define __Logging__ 00003 00004 /* 00005 * This module provides the logging functionality of the system. 00006 * The LogEvent function is inserted into the code at key points, passing an 00007 * integer value which represents the state. These are listed in the #defines 00008 * below. 00009 * The values are then insterted into a mailbox, where they fetched by the 00010 * CheckLoggingQueue function. This returns a string which also has a suffix 00011 * indicating logging. This can then be outputted to an interface. This function 00012 * should be placed within a repeating thread. To use the function with a printf 00013 * statement, you must convert between string and char array using: 00014 00015 string QueueData = CheckLoggingQueue(); //Get the data from queue 00016 char char_array[QueueData.length()+1]; //Char array for message 00017 strcpy(char_array, QueueData.c_str()); //String must be converted to char array for printf 00018 PC.printf("%s\n\r",char_array); 00019 00020 * Functions incorporate a check on the state of Logging before acting. CheckLoggingQueue 00021 * will return an empty string if there is no logging. 00022 */ 00023 #include "mbed.h" 00024 #include "rtos.h" 00025 #include <string> 00026 00027 #define Log_SampleTicker 0 00028 #define Log_EnviromSampled 1 00029 #define Log_TimeSampled 2 00030 #define Log_IndexInc 3 00031 #define Log_OldestInc 4 00032 #define Log_LCDScroll 5 00033 #define Log_LCDMessage 6 00034 #define Log_LCDTime 7 00035 #define Log_LCDOverflow 8 00036 #define Log_LCDNoData 9 00037 #define Log_SetDateFail 10 00038 #define Log_SetTimeFail 11 00039 #define Log_EthConfig 12 00040 #define Log_EthRequest 13 00041 #define Log_SDInitFail 14 00042 #define Log_SDFileOpenFail 15 00043 #define Log_SDRemoved 16 00044 #define Log_SDInserted 17 00045 #define Log_SDDeInit 18 00046 #define Log_SDDismountFail 19 00047 00048 extern Mail<uint32_t,32> ActivityLog; //Mailbox that holds the activity 00049 00050 extern bool logging; 00051 //Has logging been enabled in the system? 00052 void LogEvent(uint32_t eventCode); 00053 //Producer function - adds state to queue 00054 string CheckLoggingQueue(void); 00055 //Consumer function - wraps the number into a string with a suffix. 00056 #endif
Generated on Tue Jul 26 2022 07:29:23 by
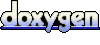