
simple tester for i2c on kl46z talking to ds1307 rtc module
Dependencies: mbed
main.cpp
00001 #include "mbed.h" 00002 00003 DigitalOut myled(LED1); 00004 I2C myi2c(PTE0,PTE1); 00005 Serial pc(USBTX,USBRX); 00006 00007 const int ds1307=0xD0; 00008 char data; 00009 00010 00011 int bcd2int(unsigned int bcd) 00012 { 00013 int pos = sizeof(bcd) * 8; 00014 int digit; 00015 int val = 0; 00016 00017 do { 00018 pos -= 4; 00019 digit = (bcd >> pos) & 0xf; 00020 val = val * 10 + digit; 00021 } while (pos > 0); 00022 00023 return val; 00024 } 00025 00026 00027 00028 00029 00030 int main() 00031 { 00032 int p; 00033 myi2c.frequency(100000); 00034 00035 myi2c.write(ds1307,0x00,1); // tell the ds1307 we want to talk to address 00036 for(p=0; p<8; p++) { 00037 myi2c.read(ds1307,&data,1); // read one byte into data 00038 00039 pc.printf("item: %d\t\tread:%d\t \n\r",p,(int)data); 00040 wait(0.01); 00041 } 00042 00043 pc.printf("\n==========\n\r\nStarting the clock now\n\r"); 00044 char cmd[2]; 00045 cmd[0]=0; 00046 cmd[1]=0; 00047 myi2c.write(ds1307,cmd,2); // tell the ds1307 we want to talk to address 00 00048 00049 00050 while(1) { 00051 myled = 1; 00052 wait(0.8); 00053 myled = 0; 00054 00055 myi2c.write(ds1307,0x00,1); // tell the ds1307 we want to talk to address 0 00056 for(p=0; p<8; p++) { 00057 myi2c.read(ds1307,&data,1); // read one byte into data 00058 00059 pc.printf("item: %d\t\tread:%d\t VAL:%d \n\r",p,(int)data,bcd2int(data)); 00060 wait(0.01); 00061 } 00062 wait(5); 00063 pc.printf("\n==========================\n\r"); 00064 } 00065 } 00066
Generated on Thu Jul 14 2022 21:06:29 by
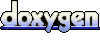