
The preloaded firmware shipped on the mBuino Development Board.
Dependencies: mbed
main.cpp
00001 /* 00002 ** This software can be freely used, even comercially, as highlighted in the license. 00003 ** 00004 ** Copyright 2014 GHI Electronics, LLC 00005 ** 00006 ** Licensed under the Apache License, Version 2.0 (the "License"); 00007 ** you may not use this file except in compliance with the License. 00008 ** You may obtain a copy of the License at 00009 ** 00010 ** http://www.apache.org/licenses/LICENSE-2.0 00011 ** 00012 ** Unless required by applicable law or agreed to in writing, software 00013 ** distributed under the License is distributed on an "AS IS" BASIS, 00014 ** WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 ** See the License for the specific language governing permissions and 00016 ** limitations under the License. 00017 ** 00018 **/ 00019 00020 #include "mbed.h" 00021 00022 DigitalOut LED[] = {(LED1), (LED2), (LED3), (LED4), (LED5), (LED6), (LED7)};// declare 7 LEDs 00023 00024 float delayTime = .05; 00025 00026 int main() 00027 { 00028 while(1) 00029 { 00030 delayTime = 0.05; 00031 for(int x = 0; x < 7; x++) 00032 { 00033 LED[x] = 1; // turn on 00034 wait(.2); // delay 00035 00036 LED[x] = 0; // turn off 00037 wait(delayTime); // delay 00038 } 00039 for(int x = 6; x >= 0; x--) 00040 { 00041 LED[x] = 1; // turn on 00042 wait(.2); // delay 00043 00044 LED[x] = 0; // turn off 00045 wait(delayTime); // delay 00046 } 00047 00048 for(int x = 0; x < 7; x++) 00049 { 00050 LED[x] = 1; // turn on 00051 wait(delayTime); // delay 00052 } 00053 for(int x = 6; x >= 0; x--) 00054 { 00055 LED[x] = 0; // turn off 00056 wait(delayTime); // delay 00057 } 00058 00059 00060 } 00061 }
Generated on Sun Jul 17 2022 09:35:12 by
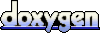