
POC1.5 prototype 2 x color sensor 2 x LM75B 3 x AnalogIn 1 x accel
Embed:
(wiki syntax)
Show/hide line numbers
edge_time.cpp
00001 #include "mbed.h" 00002 #include "edge_time.h" 00003 00004 static const uint8_t daysInMonth[12] = { 00005 31, 28, 31, 30, 00006 31, 30, 31, 31, 00007 30, 31, 30, 31 00008 } ; 00009 00010 const char *nameOfDay[7] = { 00011 "Sunday", "Monday", "Tuesday", "Wednesday", 00012 "Thursday", "Friday", "Saturday" 00013 } ; 00014 00015 uint32_t edge_time = 0 ; 00016 uint32_t utc_offset = 9 * 60 * 60 ; 00017 tm current_time ; 00018 Ticker *tokei = 0 ; 00019 00020 void inc_sec(void) 00021 { 00022 edge_time++ ; 00023 } 00024 00025 void init_timer(void) 00026 { 00027 tokei = new Ticker() ; 00028 tokei->attach(inc_sec, 1.0) ; 00029 } 00030 00031 void set_time(const uint16_t valueLen, const uint8_t *value) 00032 { 00033 uint32_t tmp_timestamp = 0 ; 00034 for (int i = 0 ; i < valueLen ; i++ ) { 00035 tmp_timestamp |= (value[i] & 0xFF) << (i * 8) ; 00036 } 00037 edge_time = tmp_timestamp ; 00038 ts2tm(edge_time, ¤t_time) ; 00039 // ts2time(edge_time, ¤t_time) ; 00040 } 00041 00042 void ts2time(uint32_t timestamp, struct tm *tm) 00043 { 00044 uint32_t seconds, minutes, hours, days, month, year ; 00045 uint32_t dayOfWeek ; 00046 00047 // timestamp += (3600 * 9) ; /* +9 hours for JST */ 00048 timestamp += utc_offset ; 00049 00050 seconds = timestamp % 60 ; 00051 minutes = timestamp / 60 ; 00052 hours = minutes / 60 ; /* +9 for JST */ 00053 minutes -= hours * 60 ; 00054 days = hours / 24 ; 00055 hours -= days * 24 ; 00056 00057 tm->tm_sec = seconds ; 00058 tm->tm_min = minutes ; 00059 tm->tm_hour = hours ; 00060 tm->tm_mday = days + 1 ; 00061 // tm->tm_mon = month ; 00062 // tm->tm_year = year ; 00063 // tm->tm_wday = dayOfWeek ; 00064 } 00065 00066 void ts2tm(uint32_t timestamp, struct tm *tm) 00067 { 00068 uint32_t seconds, minutes, hours, days, month, year ; 00069 uint32_t dayOfWeek ; 00070 00071 // timestamp += (3600 * 9) ; /* +9 hours for JST */ 00072 timestamp += utc_offset ; 00073 00074 seconds = timestamp % 60 ; 00075 minutes = timestamp / 60 ; 00076 hours = minutes / 60 ; /* +9 for JST */ 00077 minutes -= hours * 60 ; 00078 days = hours / 24 ; 00079 hours -= days * 24 ; 00080 00081 /* Unix timestamp start 1-Jan-1970 Thursday */ 00082 year = 1970 ; 00083 dayOfWeek = 4 ; /* Thursday */ 00084 00085 while(1) { 00086 bool isLeapYear = 00087 (((year % 4) == 0) 00088 &&(((year % 100) != 0) 00089 || ((year % 400) == 0))) ; 00090 uint16_t daysInYear = isLeapYear ? 366 : 365 ; 00091 if (days >= daysInYear) { 00092 dayOfWeek += isLeapYear ? 2 : 1 ; 00093 days -= daysInYear ; 00094 if (dayOfWeek >= 7) { 00095 dayOfWeek -= 7 ; 00096 } 00097 year++ ; 00098 } else { 00099 tm->tm_yday = days ; 00100 dayOfWeek += days ; 00101 dayOfWeek %= 7 ; 00102 00103 /* calc the month and the day */ 00104 for (month = 0 ; month < 12 ; month++) { 00105 uint8_t dim = daysInMonth[month] ; 00106 00107 /* add a day to feburary if this is a leap year */ 00108 if ((month == 1) && (isLeapYear)) { 00109 dim++ ; 00110 } 00111 00112 if (days >= dim) { 00113 days -= dim ; 00114 } else { 00115 break ; 00116 } 00117 } 00118 break ; 00119 } 00120 } 00121 tm->tm_sec = seconds ; 00122 tm->tm_min = minutes ; 00123 tm->tm_hour = hours ; 00124 tm->tm_mday = days + 1 ; 00125 tm->tm_mon = month ; 00126 tm->tm_year = year ; 00127 tm->tm_wday = dayOfWeek ; 00128 } 00129 00130 void print_time(struct tm *tm) 00131 { 00132 printf("%02d:%02d:%02d", 00133 tm->tm_hour, 00134 tm->tm_min, 00135 tm->tm_sec ) ; 00136 } 00137 00138 void print_time(uint32_t thetime) 00139 { 00140 struct tm timestruct ; 00141 ts2time(thetime, ×truct) ; 00142 print_time(×truct) ; 00143 } 00144 00145 void print_time(void) 00146 { 00147 struct tm timestruct ; 00148 ts2time(edge_time, ×truct) ; 00149 print_time(×truct) ; 00150 } 00151 00152 void print_date(struct tm *tm) 00153 { 00154 printf("%d/%d/%d %02d:%02d:%02d", 00155 tm->tm_year, 00156 tm->tm_mon + 1, 00157 tm->tm_mday, 00158 tm->tm_hour, 00159 tm->tm_min, 00160 tm->tm_sec 00161 ) ; 00162 } 00163 00164 void print_date_wd(struct tm *tm) 00165 { 00166 printf("%d/%d/%d %02d:%02d:%02d (%s)", 00167 tm->tm_year, 00168 tm->tm_mon + 1, 00169 tm->tm_mday, 00170 tm->tm_hour, 00171 tm->tm_min, 00172 tm->tm_sec, 00173 nameOfDay[tm->tm_wday] 00174 ) ; 00175 } 00176 00177 void time2str(struct tm *tm, char *timestr) 00178 { 00179 sprintf(timestr, "%02d:%02d:%02d", 00180 tm->tm_hour, 00181 tm->tm_min, 00182 tm->tm_sec ) ; 00183 } 00184 00185 void time2str(char *timestr) 00186 { 00187 struct tm timestruct ; 00188 ts2time(edge_time, ×truct) ; 00189 time2str(×truct, timestr) ; 00190 } 00191 00192 int32_t time2seq(uint32_t timestamp) 00193 { 00194 struct tm timestruct ; 00195 int32_t result ; 00196 ts2time(timestamp, ×truct) ; 00197 result = timestruct.tm_hour * 10000 00198 + timestruct.tm_min * 100 00199 + timestruct.tm_sec ; 00200 return(result) ; 00201 } 00202 00203 void time2seq(uint32_t timestamp, char *timestr) 00204 { 00205 struct tm timestruct ; 00206 ts2tm(timestamp, ×truct) ; 00207 sprintf(timestr, "%d%02d%02d%02d%02d%02d", 00208 timestruct.tm_year, 00209 timestruct.tm_mon + 1, 00210 timestruct.tm_mday, 00211 timestruct.tm_hour, 00212 timestruct.tm_min, 00213 timestruct.tm_sec 00214 ) ; 00215 } 00216 00217 void time2date(struct tm *tm, char *datestr) 00218 { 00219 sprintf(datestr, "%d/%d/%d %02d:%02d:%02d (%s)", 00220 tm->tm_year, 00221 tm->tm_mon + 1, 00222 tm->tm_mday, 00223 tm->tm_hour, 00224 tm->tm_min, 00225 tm->tm_sec, 00226 nameOfDay[tm->tm_wday] 00227 ) ; 00228 }
Generated on Tue Jul 12 2022 21:13:41 by
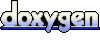