
POC1.5 prototype 2 x color sensor 2 x LM75B 3 x AnalogIn 1 x accel
Embed:
(wiki syntax)
Show/hide line numbers
edge_sensor.cpp
00001 #include "mbed.h" 00002 #include "vt100.h" 00003 #include "edge_time.h" 00004 #include "edge_sensor.h" 00005 00006 extern vt100 *tty ; 00007 static uint16_t id = 0 ; 00008 00009 edge_sensor::edge_sensor() 00010 { 00011 _interval = 0 ; 00012 _prev_status = EDGE_SENSOR_INACTIVE ; 00013 _status = EDGE_SENSOR_INACTIVE ; 00014 _id = id++ ; 00015 _enable = false ; 00016 _error_count = 0 ; 00017 } 00018 00019 edge_sensor::~edge_sensor() 00020 { 00021 } 00022 00023 void edge_sensor::reset(void) 00024 { 00025 _status = EDGE_SENSOR_INACTIVE ; 00026 } 00027 00028 void edge_sensor::enable(void) 00029 { 00030 _enable = true ; 00031 } 00032 00033 void edge_sensor::disable(void) 00034 { 00035 _enable = false ; 00036 } 00037 00038 bool edge_sensor::isEnabled(void) 00039 { 00040 return( _enable ) ; 00041 } 00042 00043 void edge_sensor::prepare(void) 00044 { 00045 // printf("Sensor %d prepare for sample\n", _id) ; 00046 } 00047 00048 void edge_sensor::sample(void) 00049 { 00050 // printf("Sensor %d sample\n", _id) ; 00051 } 00052 00053 int edge_sensor::deliver(void) 00054 { 00055 // printf("Sensor %d data delivered\n", _id) ; 00056 /* usually return( result == afSUCCESS ) ; */ 00057 return 1 ; /* return non zero for success */ 00058 } 00059 00060 void edge_sensor::toJson(char *buf) 00061 { 00062 sprintf(buf, "EDGE_SENSOR%d is not a real sensor", _id) ; 00063 } 00064 00065 void edge_sensor::setInterval(uint16_t interval) 00066 { 00067 _interval = interval ; 00068 } 00069 00070 uint16_t edge_sensor::getInterval(void) 00071 { 00072 return( _interval ) ; 00073 } 00074 00075 int edge_sensor::getStatus(void) 00076 { 00077 return( _status ) ; 00078 } 00079 00080 /* 00081 #define EDGE_SENSOR_INACTIVE 0 00082 #define EDGE_SENSOR_WAIT 1 00083 #define EDGE_SENSOR_READY 2 00084 #define EDGE_SENSOR_PREPARED 3 00085 #define EDGE_SENSOR_SAMPLED 4 00086 #define EDGE_SENSOR_DELIVERD 5 00087 */ 00088 00089 int edge_sensor::runStateMachine(void) 00090 { 00091 int result ; 00092 switch(_status) { 00093 case EDGE_SENSOR_INACTIVE: /* inactive */ 00094 if (isEnabled()) { 00095 _status = EDGE_SENSOR_WAIT ; 00096 } 00097 _prev_status = EDGE_SENSOR_INACTIVE ; 00098 break ; 00099 case EDGE_SENSOR_WAIT: /* wait for interval time expires */ 00100 if (_prev_status != _status) { 00101 _end_interval = edge_time + _interval ; 00102 } 00103 _prev_status = EDGE_SENSOR_WAIT ; 00104 if (edge_time >= _end_interval) { 00105 _status = EDGE_SENSOR_READY ; 00106 } 00107 break ; 00108 case EDGE_SENSOR_READY: /* prepare to sample */ 00109 sample() ; 00110 _prev_status = EDGE_SENSOR_READY ; 00111 _status = EDGE_SENSOR_SAMPLED ; 00112 break ; 00113 case EDGE_SENSOR_SAMPLED: /* data is ready, wait for delivery */ 00114 if (_prev_status != EDGE_SENSOR_SAMPLED) { 00115 _error_count = 0 ; 00116 } 00117 result = deliver() ; 00118 if (result) { 00119 _status = EDGE_SENSOR_INACTIVE ; 00120 } else { 00121 _error_count++ ; 00122 } 00123 _prev_status = EDGE_SENSOR_SAMPLED ; 00124 00125 break ; 00126 } 00127 return(_status) ; 00128 } 00129
Generated on Tue Jul 12 2022 21:13:41 by
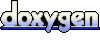