
POC1.5 prototype 2 x color sensor 2 x LM75B 3 x AnalogIn 1 x accel
Embed:
(wiki syntax)
Show/hide line numbers
afLib.h
00001 /** 00002 * Copyright 2015 Afero, Inc. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef AFLIB_H__ 00018 #define AFLIB_H__ 00019 00020 #include "mbed.h" 00021 #include "iafLib.h" 00022 #include "SPI.h" 00023 #include "Command.h" 00024 #include "StatusCommand.h" 00025 #include "afSPI.h" 00026 // #include "WatchDogWrapper.hpp" 00027 00028 #define STATE_IDLE 0 00029 #define STATE_STATUS_SYNC 1 00030 #define STATE_STATUS_ACK 3 00031 #define STATE_SEND_BYTES 4 00032 #define STATE_RECV_BYTES 5 00033 #define STATE_CMD_COMPLETE 6 00034 00035 #define SPI_FRAME_LEN 16 00036 00037 #define REQUEST_QUEUE_SIZE 10 00038 00039 typedef struct { 00040 uint8_t messageType; 00041 uint16_t attrId; 00042 uint8_t requestId; 00043 uint16_t valueLen; 00044 uint8_t *p_value; 00045 } request_t; 00046 00047 class afLib : public iafLib { 00048 public: 00049 afLib(PinName mcuInterrupt, isr isrWrapper, 00050 onAttributeSet attrSet, onAttributeSetComplete attrSetComplete, afSPI *theSPI); 00051 //wsugi 20161128 00052 ~afLib(); 00053 //wsugi 20161128 00054 00055 // motoo tanaka 20171116 00056 virtual int getRequestId(void) ; 00057 // motoo tanaka 20171116 00058 00059 virtual void loop(void); 00060 00061 virtual int getAttribute(const uint16_t attrId); 00062 00063 virtual int setAttributeBool(const uint16_t attrId, const bool value); 00064 00065 virtual int setAttribute8(const uint16_t attrId, const int8_t value); 00066 00067 virtual int setAttribute16(const uint16_t attrId, const int16_t value); 00068 00069 virtual int setAttribute32(const uint16_t attrId, const int32_t value); 00070 00071 virtual int setAttribute64(const uint16_t attrId, const int64_t value); 00072 00073 virtual int setAttribute(const uint16_t attrId, const string &value); 00074 00075 virtual int setAttribute(const uint16_t attrId, const uint16_t valueLen, const char *value); 00076 00077 virtual int setAttribute(const uint16_t attrId, const uint16_t valueLen, const uint8_t *value); 00078 00079 virtual int setAttributeComplete(uint8_t requestId, const uint16_t attrId, const uint16_t valueLen, const uint8_t *value); 00080 00081 virtual bool isIdle(); 00082 00083 virtual void mcuISR(); 00084 00085 private: 00086 Timeout deathWish; 00087 Timer *checkLastSync; 00088 static void kick_the_bucket(); 00089 afSPI *_theSPI; 00090 00091 //SPISettings _spiSettings; 00092 volatile int _interrupts_pending; 00093 int _state; 00094 uint16_t _bytesToSend; 00095 uint16_t _bytesToRecv; 00096 uint8_t _requestId; 00097 uint16_t _outstandingSetGetAttrId; 00098 00099 // Application Callbacks. 00100 onAttributeSet _onAttrSet; 00101 onAttributeSetComplete _onAttrSetComplete; 00102 00103 Command *_writeCmd; 00104 uint16_t _writeBufferLen; 00105 uint8_t *_writeBuffer; 00106 00107 Command *_readCmd; 00108 uint16_t _readBufferLen; 00109 uint8_t *_readBuffer; 00110 00111 int _writeCmdOffset; 00112 int _readCmdOffset; 00113 00114 StatusCommand *_txStatus; 00115 StatusCommand *_rxStatus; 00116 00117 request_t _request; 00118 request_t _requestQueue[REQUEST_QUEUE_SIZE]; 00119 00120 #ifdef ATTRIBUTE_CLI 00121 int parseCommand(const char *cmd); 00122 #endif 00123 00124 void sendCommand(void); 00125 00126 void runStateMachine(void); 00127 00128 void printState(int state); 00129 00130 //void beginSPI(); 00131 00132 //void endSPI(); 00133 00134 int exchangeStatus(StatusCommand *tx, StatusCommand *rx); 00135 00136 bool inSync(StatusCommand *tx, StatusCommand *rx); 00137 00138 int writeStatus(StatusCommand *c); 00139 00140 void sendBytes(); 00141 00142 void recvBytes(); 00143 00144 void dumpBytes(char *label, int len, uint8_t *bytes); 00145 00146 void updateIntsPending(int amount); 00147 00148 void queueInit(void); 00149 00150 int queuePut(uint8_t messageType, uint8_t requestId, const uint16_t attributeId, uint16_t valueLen, const uint8_t *value); 00151 00152 int queueGet(uint8_t *messageType, uint8_t *requestId, uint16_t *attributeId, uint16_t *valueLen, uint8_t **value); 00153 00154 int doGetAttribute(uint8_t requestId, uint16_t attrId); 00155 00156 int doSetAttribute(uint8_t requestId, uint16_t attrId, uint16_t valueLen, uint8_t *value); 00157 00158 int doUpdateAttribute(uint8_t requestId, uint16_t attrId, uint8_t status, uint16_t valueLen, uint8_t *value); 00159 00160 void onStateIdle(void); 00161 void onStateSync(void); 00162 void onStateAck(void); 00163 void onStateSendBytes(void); 00164 void onStateRecvBytes(void); 00165 void onStateCmdComplete(void); 00166 void printTransaction(uint8_t *rbytes, int len); 00167 InterruptIn fco; 00168 }; 00169 00170 #endif // AFLIB_H__
Generated on Tue Jul 12 2022 21:13:41 by
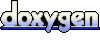