
POC1.5 prototype 2 x color sensor 2 x LM75B 3 x AnalogIn 1 x accel
Embed:
(wiki syntax)
Show/hide line numbers
StatusCommand.cpp
00001 /** 00002 * Copyright 2015 Afero, Inc. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "StatusCommand.h" 00018 00019 StatusCommand::StatusCommand(uint16_t bytesToSend) { 00020 _cmd = 0x30; 00021 _bytesToSend = bytesToSend; 00022 _bytesToRecv = 0; 00023 } 00024 00025 StatusCommand::StatusCommand() { 00026 _cmd = 0x30; 00027 _bytesToSend = 0; 00028 _bytesToRecv = 0; 00029 } 00030 00031 StatusCommand::~StatusCommand() { 00032 } 00033 00034 uint16_t StatusCommand::getSize() { 00035 return sizeof(_cmd) + sizeof(_bytesToSend) + sizeof(_bytesToRecv); 00036 } 00037 00038 uint16_t StatusCommand::getBytes(int *bytes) { 00039 int index = 0; 00040 00041 bytes[index++] = (_cmd); 00042 bytes[index++] = (_bytesToSend & 0xff); 00043 bytes[index++] = ((_bytesToSend >> 8) & 0xff); 00044 bytes[index++] = (_bytesToRecv & 0xff); 00045 bytes[index++] = ((_bytesToRecv >> 8) & 0xff); 00046 00047 return index; 00048 } 00049 00050 uint8_t StatusCommand::calcChecksum() { 00051 uint8_t result = 0; 00052 00053 result += (_cmd); 00054 result += (_bytesToSend & 0xff); 00055 result += ((_bytesToSend >> 8) & 0xff); 00056 result += (_bytesToRecv & 0xff); 00057 result += ((_bytesToRecv >> 8) & 0xff); 00058 00059 return result; 00060 } 00061 00062 void StatusCommand::setChecksum(uint8_t checksum) { 00063 _checksum = checksum; 00064 } 00065 00066 uint8_t StatusCommand::getChecksum() { 00067 uint8_t result = 0; 00068 00069 result += (_cmd); 00070 result += (_bytesToSend & 0xff); 00071 result += ((_bytesToSend >> 8) & 0xff); 00072 result += (_bytesToRecv & 0xff); 00073 result += ((_bytesToRecv >> 8) & 0xff); 00074 00075 return result; 00076 } 00077 00078 void StatusCommand::setAck(bool ack) { 00079 _cmd = ack ? 0x31 : 0x30; 00080 } 00081 00082 void StatusCommand::setBytesToSend(uint16_t bytesToSend) { 00083 _bytesToSend = bytesToSend; 00084 } 00085 00086 uint16_t StatusCommand::getBytesToSend() { 00087 return _bytesToSend; 00088 } 00089 00090 void StatusCommand::setBytesToRecv(uint16_t bytesToRecv) { 00091 _bytesToRecv = bytesToRecv; 00092 } 00093 00094 uint16_t StatusCommand::getBytesToRecv() { 00095 return _bytesToRecv; 00096 } 00097 00098 bool StatusCommand::equals(StatusCommand *statusCommand) { 00099 return (_cmd == statusCommand->_cmd && _bytesToSend == statusCommand->_bytesToSend && 00100 _bytesToRecv == statusCommand->_bytesToRecv); 00101 } 00102 00103 bool StatusCommand::isValid() { 00104 return (_checksum == calcChecksum()) && (_cmd == 0x30 || _cmd == 0x31); 00105 } 00106 00107 void StatusCommand::dumpBytes() { 00108 int len = getSize(); 00109 int bytes[len]; 00110 getBytes(bytes); 00111 00112 SERIAL_PRINT_DBG_ASR("len : %d\n",len); 00113 SERIAL_PRINT_DBG_ASR("data : "); 00114 for (int i = 0; i < len; i++) { 00115 if (i > 0) { 00116 SERIAL_PRINT_DBG_ASR(", "); 00117 } 00118 int b = bytes[i] & 0xff; 00119 if (b < 0x10) { 00120 SERIAL_PRINT_DBG_ASR("0x%08x",b); 00121 } else { 00122 SERIAL_PRINT_DBG_ASR("0x%08x",b); 00123 } 00124 } 00125 SERIAL_PRINT_DBG_ASR("\n"); 00126 } 00127 00128 void StatusCommand::dump() { 00129 SERIAL_PRINT_DBG_ASR("cmd : %s\n",_cmd == 0x30 ? "STATUS" : "STATUS_ACK"); 00130 SERIAL_PRINT_DBG_ASR("bytes to send : %d\n",_bytesToSend); 00131 SERIAL_PRINT_DBG_ASR("bytes to receive : %d\n",_bytesToRecv); 00132 } 00133
Generated on Tue Jul 12 2022 21:13:41 by
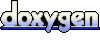