
POC1.5 prototype 2 x color sensor 2 x LM75B 3 x AnalogIn 1 x accel
Embed:
(wiki syntax)
Show/hide line numbers
SO1602A.cpp
00001 #include "mbed.h" 00002 #include "SO1602A.h" 00003 00004 #define BIT_DISPLAY_CTRL 0x08 00005 #define BIT_DISPLAY_ON 0x04 00006 #define BIT_CURSOR_ON 0x02 00007 #define BIT_BLINKING_ON 0x01 00008 00009 #define CLEAR_DISPLAY 0x01 00010 #define RETURN_HOME 0x02 00011 00012 void SO1602A::test(void) 00013 { 00014 char test_str[] = "I2C OLED WHITE1234" ; 00015 int i ; 00016 for (i = 0 ; test_str[i] != 0 ; i++ ) { 00017 writeData(test_str[i]) ; 00018 } 00019 writeCommand(0x20+0x80) ; // line 2 column 1 00020 for (i = 0 ; i < 10 ; i++ ) { 00021 writeData(i+0xB1) ; 00022 } 00023 contrast_max() ; 00024 writeCommand(0x0F) ; 00025 wait_ms(10) ; 00026 } 00027 00028 SO1602A::SO1602A(I2C *i2c, int addr) : m_addr(addr<<1) { 00029 p_i2c = i2c ; 00030 init_oled() ; 00031 } 00032 00033 SO1602A::~SO1602A(void) 00034 { 00035 } 00036 00037 void SO1602A::locate(int x, int y) 00038 { 00039 uint8_t data ; 00040 if (x < 0) x = 0 ; 00041 if (x > 0xf) x = 0xf ; 00042 if (y < 0) y = 0 ; 00043 if (y > 1) y = 1 ; 00044 data = 0x80 + x ; 00045 if (y == 1) { 00046 data |= 0x20 ; 00047 } 00048 writeCommand(data) ; 00049 } 00050 00051 void SO1602A::putStr(char *str) 00052 { 00053 int i ; 00054 for (i = 0 ; str[i] ; i++ ) { 00055 writeData(str[i]) ; 00056 } 00057 wait_ms(10) ; 00058 } 00059 00060 void SO1602A::writeData(uint8_t data) 00061 { 00062 int result ; 00063 00064 uint8_t t[2] ; 00065 t[0] = 0x40 ; 00066 t[1] = data ; 00067 writeRegs(t, 2) ; 00068 00069 wait_ms(1) ; 00070 } 00071 00072 void SO1602A::writeCommand(uint8_t command) 00073 { 00074 int result ; 00075 00076 uint8_t t[2] ; 00077 t[0] = 0 ; 00078 t[1] = command ; 00079 writeRegs(t, 2) ; 00080 wait_ms(10) ; 00081 } 00082 00083 void SO1602A::contrast_max(void) 00084 { 00085 writeCommand(0x2A) ; // RE=1 00086 writeCommand(0x79) ; // SD=1 00087 writeCommand(0x81) ; // set Contrast 00088 writeCommand(0xFF) ; // brightness MAX 00089 writeCommand(0x78) ; // SD=0 00090 writeCommand(0x28) ; // 2C = High char 28 = Normal 00091 wait_ms(100) ; 00092 } 00093 00094 void SO1602A::init_oled(void) 00095 { 00096 wait_ms(100) ; 00097 writeCommand(CLEAR_DISPLAY) ; // Clear Display 00098 wait_ms(20) ; 00099 writeCommand(RETURN_HOME) ; // Return Home 00100 wait_ms(2) ; 00101 // writeCommand( 0x0F ) ; 00102 writeCommand( 0x0C ) ; 00103 wait_ms(2) ; 00104 writeCommand(CLEAR_DISPLAY) ; 00105 wait_ms(100) ; 00106 } 00107 00108 void SO1602A::cls(void) 00109 { 00110 writeCommand(CLEAR_DISPLAY) ; 00111 wait_ms(20) ; 00112 } 00113 00114 void SO1602A::returnHome(void) 00115 { 00116 writeCommand(RETURN_HOME) ; 00117 wait_ms(2) ; 00118 } 00119 00120 void SO1602A::displayCTRL(uint8_t data) 00121 { 00122 writeCommand(BIT_DISPLAY_CTRL | data) ; 00123 wait_ms(2) ; 00124 } 00125 00126 void SO1602A::SO1602A::displayOff(void) 00127 { 00128 writeCommand(BIT_DISPLAY_CTRL) ; 00129 wait_ms(2) ; 00130 } 00131 00132 int SO1602A::readRegs(int addr, uint8_t * data, int len) 00133 { 00134 int result ; 00135 char t[1] = {addr}; 00136 result = p_i2c->write(m_addr, t, 1, true); 00137 if (result == 0) { 00138 result = p_i2c->read(m_addr, (char *)data, len); 00139 } 00140 return( result ) ; 00141 } 00142 00143 int SO1602A::writeRegs(uint8_t * data, int len) { 00144 int result ; 00145 result = p_i2c->write(m_addr, (char *)data, len); 00146 return(result) ; 00147 }
Generated on Tue Jul 12 2022 21:13:41 by
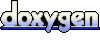