
POC1.5 prototype 2 x color sensor 2 x LM75B 3 x AnalogIn 1 x accel
Embed:
(wiki syntax)
Show/hide line numbers
PSE530.cpp
00001 #include "mbed.h" 00002 #include "PSE530.h" 00003 00004 /** 00005 * SMC PSE530 pressure sensor 00006 * analog output 1.0V - 5.0V 00007 * 1.0V : 0 00008 * 5.0V : 1MPa 00009 * (at 0.6V : -0.1MPa) 00010 * Our sensor I/F converts 0-5V to 0-3V 00011 * So we suppose V = Analog Float Value : Pressure 00012 * 0.6V = 0.2 : 0 00013 * 3.0V = 1.0 : 1MPa 00014 */ 00015 00016 /** 00017 * conversion from Pa to kgf/cm2 00018 * 98,066.5 Pa = 1 kgf/cm2 00019 * 1 Pa = 1 / 98066.6 kgf/cm2 00020 */ 00021 00022 PSE530::PSE530(AnalogIn *ain) 00023 { 00024 _ain = ain ; 00025 } 00026 00027 PSE530::~PSE530(void) 00028 { 00029 if (_ain) { 00030 delete _ain ; 00031 } 00032 } 00033 00034 /** 00035 * On FRDM-KL25Z ADC's AREF is about 3.28V 00036 * Where the converted pressure output is 0 to 3.21V 00037 * So we must map ADC output 0 to 3.21/3.28 as full scale 00038 * 00039 * Then according to the datasheet of PSE530 00040 * when full range is 0V to 5V 00041 * 1V is 0 and 5V is 1MPa which is converted to 00042 * 0.642/3.28 to 3.21/3.28 ~ 0.195731 to 0.9786585. 00043 * The linear equation of 00044 * y = a x + b 00045 * 0 = a * 0.195731 + b 00046 * 1 = a * 0.978658 + b 00047 * results a = 1.277, b = -0.250 00048 */ 00049 float PSE530::getPressure(void) 00050 { 00051 float coef_A = 1.277 ; 00052 float coef_B = -0.250 ; 00053 float av = 0.0 ; 00054 float value = 0.0 ; 00055 av = coef_A * _ain->read() + coef_B ; 00056 // printf("Pressure ADC = %.4f\n", av) ; 00057 value = 1000000 * av ; /* 1MPa at 1.0 */ 00058 value = value / 98066.5 ; /* Pa -> kgf/cm2 */ 00059 return( value ) ; 00060 }
Generated on Tue Jul 12 2022 21:13:41 by
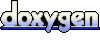