
POC1.5 prototype 2 x color sensor 2 x LM75B 3 x AnalogIn 1 x accel
Embed:
(wiki syntax)
Show/hide line numbers
Command.h
00001 /** 00002 * Copyright 2015 Afero, Inc. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef COMMAND_H__ 00018 #define COMMAND_H__ 00019 00020 #include "mbed.h" //wsugi 00021 #include <string> //wsugi 00022 00023 using namespace std; //wsugi 00024 00025 #define SPI_CMD_MAX_LEN 256 00026 00027 #define UPDATE_REASON_UNKNOWN 0 00028 #define UPDATE_REASON_LOCAL_UPDATE 1 00029 #define UPDATE_REASON_SERVICE_SET 2 00030 #define UPDATE_REASON_MCU_SET 3 00031 #define UPDATE_REASON_RELINK 4 00032 #define UPDATE_REASON_REBOOT 5 00033 00034 class Command { 00035 public: 00036 Command(uint16_t len, uint8_t *bytes); 00037 00038 Command(uint8_t requestId, const char *str); 00039 00040 Command(uint8_t requestId, uint8_t cmd, uint16_t attrId); 00041 00042 Command(uint8_t requestId, uint8_t cmd, uint16_t attrId, uint16_t valueLen, uint8_t *value); 00043 00044 Command(uint8_t requestId, uint8_t cmd, uint16_t attrId, uint8_t status, uint8_t reason, uint16_t valueLen, 00045 uint8_t *value); 00046 00047 Command(); 00048 00049 ~Command(); 00050 00051 uint8_t getCommand(); 00052 00053 uint8_t getReqId(); 00054 00055 uint16_t getAttrId(); 00056 00057 uint16_t getValueLen(); 00058 00059 void getValue(uint8_t *value); 00060 00061 uint8_t *getValueP(); 00062 00063 uint16_t getSize(); 00064 00065 uint16_t getBytes(uint8_t *bytes); 00066 00067 bool isValid(); 00068 00069 void dump(); 00070 00071 void dumpBytes(); 00072 00073 private: 00074 uint8_t getVal(char c); 00075 int strToValue(char *valueStr, uint8_t *value); 00076 00077 uint8_t strToCmd(char *cmdStr); 00078 00079 uint16_t strToAttrId(char *attrIdStr); 00080 00081 uint16_t _len; 00082 uint8_t _cmd; 00083 uint8_t _requestId; 00084 uint16_t _attrId; 00085 uint8_t _status; 00086 uint8_t _reason; 00087 uint16_t _valueLen; 00088 uint8_t *_value; 00089 00090 char _printBuf[256]; 00091 00092 }; 00093 00094 #endif // COMMAND_H__
Generated on Tue Jul 12 2022 21:13:41 by
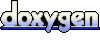