
POC1.5 prototype 2 x color sensor 2 x LM75B 3 x AnalogIn 1 x accel
Embed:
(wiki syntax)
Show/hide line numbers
Command.cpp
00001 /** 00002 * Copyright 2015 Afero, Inc. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 //wsugi #include "Arduino.h" 00018 #include "mbed.h" 00019 #include <stdio.h> 00020 #include "Command.h" 00021 #include "msg_types.h" 00022 #include "DebugIO.hpp" 00023 00024 #define CMD_HDR_LEN 4 // 4 byte header on all commands 00025 #define CMD_VAL_LEN 2 // 2 byte value length for commands that have a value 00026 00027 const char *CMD_NAMES[] = {"SET ", "GET ", "UPDATE"}; 00028 00029 00030 Command::Command(uint16_t len, uint8_t *bytes) { 00031 int index = 0; 00032 00033 _cmd = bytes[index++]; 00034 _requestId = bytes[index++]; 00035 _attrId = bytes[index + 0] | bytes[index + 1] << 8; 00036 index += 2; 00037 00038 if (_cmd == MSG_TYPE_GET) { 00039 return; 00040 } 00041 if (_cmd == MSG_TYPE_UPDATE) { 00042 _status = bytes[index++]; 00043 _reason = bytes[index++]; 00044 } 00045 00046 _valueLen = bytes[index + 0] | bytes[index + 1] << 8; 00047 index += 2; 00048 _value = new uint8_t[_valueLen]; 00049 for (int i = 0; i < _valueLen; i++) { 00050 _value[i] = bytes[index + i]; 00051 } 00052 } 00053 00054 Command::Command(uint8_t requestId, const char *str) { 00055 _requestId = requestId & 0xff; 00056 00057 char *cp; //wsugi = strdup(str); 00058 //wsugi 00 start 00059 { 00060 int length = strlen(str)+1; 00061 cp = (char*)malloc(length); 00062 strcpy(cp,str); 00063 } 00064 //wsugi 00 end 00065 char *tok = strtok(cp, " "); 00066 _cmd = strToCmd(tok); 00067 00068 tok = strtok(NULL, " "); 00069 _attrId = strToAttrId(tok); 00070 00071 if (_cmd == MSG_TYPE_GET) { 00072 _valueLen = 0; 00073 _value = NULL; 00074 } else { 00075 tok = strtok(NULL, " "); 00076 _valueLen = strlen(tok) / 2; 00077 _value = new uint8_t[_valueLen]; 00078 strToValue(tok, _value); 00079 } 00080 00081 free(cp); 00082 } 00083 00084 Command::Command(uint8_t requestId, uint8_t cmd, uint16_t attrId) { 00085 _requestId = requestId; 00086 _cmd = cmd; 00087 _attrId = attrId; 00088 _valueLen = 0; 00089 _value = NULL; 00090 } 00091 00092 Command::Command(uint8_t requestId, uint8_t cmd, uint16_t attrId, uint16_t valueLen, uint8_t *value) { 00093 _requestId = requestId; 00094 _cmd = cmd; 00095 _attrId = attrId; 00096 _valueLen = valueLen; 00097 _value = new uint8_t[_valueLen]; 00098 memcpy(_value, value, valueLen); 00099 } 00100 00101 Command::Command(uint8_t requestId, uint8_t cmd, uint16_t attrId, uint8_t status, uint8_t reason, uint16_t valueLen, 00102 uint8_t *value) { 00103 _requestId = requestId; 00104 _cmd = cmd; 00105 _attrId = attrId; 00106 _status = status; 00107 _reason = reason; 00108 _valueLen = valueLen; 00109 _value = new uint8_t[_valueLen]; 00110 memcpy(_value, value, valueLen); 00111 } 00112 00113 Command::Command() { 00114 } 00115 00116 Command::~Command() { 00117 if (_value != NULL) { 00118 delete[] _value; //wsugi delete (_value); 00119 } 00120 } 00121 00122 int Command::strToValue(char *valueStr, uint8_t *value) { 00123 for (int i = 0; i < (int) (strlen(valueStr) / 2); i++) { 00124 int j = i * 2; 00125 value[i] = getVal(valueStr[j + 1]) + (getVal(valueStr[j]) << 4); 00126 } 00127 00128 return 0; 00129 } 00130 00131 uint16_t Command::strToAttrId(char *attrIdStr) { 00132 return atoi(attrIdStr); 00133 //return String(attrIdStr).toInt(); 00134 } 00135 00136 uint8_t Command::strToCmd(char *cmdStr) { 00137 char c = cmdStr[0]; 00138 if (c == 'g' || c == 'G') { 00139 return MSG_TYPE_GET; 00140 } else if (c == 's' || c == 'S') { 00141 return MSG_TYPE_SET; 00142 } else if (c == 'u' || c == 'U') { 00143 return MSG_TYPE_UPDATE; 00144 } 00145 00146 return 0xFF ; 00147 } 00148 00149 uint8_t Command::getCommand() { 00150 return _cmd; 00151 } 00152 00153 uint8_t Command::getReqId() { 00154 return _requestId; 00155 } 00156 00157 uint16_t Command::getAttrId() { 00158 return _attrId; 00159 } 00160 00161 uint16_t Command::getValueLen() { 00162 return _valueLen; 00163 } 00164 00165 void Command::getValue(uint8_t *value) { 00166 for (int i = 0; i < _valueLen; i++) { 00167 value[i] = _value[i]; 00168 } 00169 } 00170 00171 uint8_t *Command::getValueP() { 00172 return _value; 00173 } 00174 00175 uint16_t Command::getSize() { 00176 uint16_t len = CMD_HDR_LEN; 00177 00178 if (_cmd != MSG_TYPE_GET) { 00179 len += CMD_VAL_LEN + _valueLen; 00180 } 00181 00182 if (_cmd == MSG_TYPE_UPDATE) { 00183 len += 2; // status byte + reason byte 00184 } 00185 00186 return len; 00187 } 00188 00189 uint16_t Command::getBytes(uint8_t *bytes) { 00190 uint16_t len = getSize(); 00191 00192 int index = 0; 00193 00194 bytes[index++] = (_cmd); 00195 00196 bytes[index++] = (_requestId); 00197 00198 bytes[index++] = (_attrId & 0xff); 00199 bytes[index++] = ((_attrId >> 8) & 0xff); 00200 00201 if (_cmd == MSG_TYPE_GET) { 00202 return len; 00203 } 00204 00205 if (_cmd == MSG_TYPE_UPDATE) { 00206 bytes[index++] = (_status); 00207 bytes[index++] = (_reason); 00208 } 00209 00210 bytes[index++] = (_valueLen & 0xff); 00211 bytes[index++] = ((_valueLen >> 8) & 0xff); 00212 00213 for (int i = 0; i < _valueLen; i++) { 00214 bytes[index++] = (_value[i]); 00215 } 00216 00217 return len; 00218 } 00219 00220 bool Command::isValid() { 00221 return (_cmd == MSG_TYPE_SET) || (_cmd == MSG_TYPE_GET) || (_cmd == MSG_TYPE_UPDATE); 00222 } 00223 00224 void Command::dumpBytes() { 00225 uint16_t len = getSize(); 00226 uint8_t bytes[len]; 00227 getBytes(bytes); 00228 00229 _printBuf[0] = 0; 00230 sprintf(_printBuf, "len: %d value: ", len); 00231 for (int i = 0; i < len; i++) { 00232 int b = bytes[i] & 0xff; 00233 sprintf(&_printBuf[strlen(_printBuf)], "%02x", b); 00234 } 00235 SERIAL_PRINT_DBG_ASR("%s\n",_printBuf); 00236 } 00237 00238 void Command::dump() { 00239 _printBuf[0] = 0; 00240 sprintf(_printBuf, "cmd: %s attr: %d value: ", 00241 CMD_NAMES[_cmd - MESSAGE_CHANNEL_BASE - 1], 00242 _attrId 00243 ); 00244 if (_cmd != MSG_TYPE_GET) { 00245 for (int i = 0; i < _valueLen; i++) { 00246 int b = _value[i] & 0xff; 00247 sprintf(&_printBuf[strlen(_printBuf)], "%02x", b); 00248 } 00249 } 00250 SERIAL_PRINT_DBG_ASR("%s\n",_printBuf); 00251 } 00252 00253 uint8_t Command::getVal(char c) { 00254 if (c >= '0' && c <= '9') 00255 return (uint8_t)(c - '0'); 00256 else if (c >= 'A' && c <= 'F') 00257 return (uint8_t)(c - 'A' + 10); 00258 else if (c >= 'a' && c <= 'f') 00259 return (uint8_t)(c - 'a' + 10); 00260 00261 SERIAL_PRINT_DBG_ASR("bad hex char: %c\n",c); 00262 00263 return 0; 00264 }
Generated on Tue Jul 12 2022 21:13:41 by
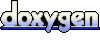