
pwm period is now 200us instead of the default 20ms veml6040 config is now AF_BIT | TRIG_BIT
Dependencies: mbed MMA8451Q USBDevice WakeUp vt100
Fork of afero_node_suntory_2017_06_15 by
mbedSPI.cpp
00001 #include "mbedSPI.h" 00002 #include "BitOrder.h" 00003 00004 mbedSPI::mbedSPI() : 00005 spi((PinName)PINS::ASR_1::SPI::MOSI, 00006 (PinName)PINS::ASR_1::SPI::MISO, 00007 (PinName)PINS::ASR_1::SPI::SCK), 00008 cs((PinName)PINS::ASR_1::SPI::CS, 00009 PINS::ASR_1::SPI::SIG::CS::DEASSERT) 00010 { 00011 spi.format( 00012 PINS::ASR_1::SPI::NUM_BITS_PER_FRAME, //SPI_NUM_BITS_PER_FRAME, 00013 PINS::ASR_1::SPI::MODE_0); 00014 spi.frequency(PINS::ASR_1::SPI::FREQUENCY); 00015 #if defined (TARGET_KL25Z) 00016 #ifndef SPI0_C1 00017 #define SPI0_C1 (*(uint8_t *)0x40076000) 00018 #endif 00019 SPI0_C1 |= 0x01 ; /* LSB First */ 00020 #elif defined (TARGET_TEENSY3_1) 00021 #define SPI0_CTAR0 ((uint32_t *)0x04002C00C) 00022 #define LSBFE_MASK 0x01000000 00023 *SPI0_CTAR0 |= LSBFE_MASK ; 00024 #endif 00025 } 00026 00027 void mbedSPI::begin() 00028 { 00029 } 00030 00031 void mbedSPI::beginSPI() /* settings are in this class */ 00032 { 00033 cs = PINS::ASR_1::SPI::SIG::CS::ASSERT; 00034 wait_us(1); 00035 } 00036 00037 void mbedSPI::endSPI() 00038 { 00039 cs = PINS::ASR_1::SPI::SIG::CS::DEASSERT; 00040 } 00041 00042 void mbedSPI::transfer(char *bytes,int len) 00043 { 00044 int i = 0; 00045 00046 for(;i<len;++i) 00047 { 00048 #if defined (TARGET_KL25Z) || (TARGET_TEENSY3_1) 00049 bytes[i] = spi.write(bytes[i]) ; 00050 #else 00051 char c = spi.write(BitOrder::flip(bytes[i])); 00052 bytes[i] = BitOrder::flip(c); 00053 #endif 00054 } 00055 }
Generated on Thu Jul 14 2022 06:24:36 by
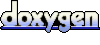