
pwm period is now 200us instead of the default 20ms veml6040 config is now AF_BIT | TRIG_BIT
Dependencies: mbed MMA8451Q USBDevice WakeUp vt100
Fork of afero_node_suntory_2017_06_15 by
VEML6040.h
00001 /* 00002 * File description 00003 * 00004 */ 00005 00006 #ifndef _VEML6040_H_ 00007 #define _VEML6040_H_ 00008 00009 #include "mbed.h" 00010 #include "Prefs.hpp" 00011 /** 00012 * RGBW Color Sensor with I2C Interface 00013 * I2C 7bit address: 0x10 00014 * 00015 */ 00016 00017 class VEML6040 00018 { 00019 public: 00020 /** 00021 * constructor 00022 * 00023 * @param sda SDA pin 00024 * @param scl SCL pin 00025 * @param addr address of the I2C peripheral 00026 */ 00027 VEML6040(PinName sda, PinName scl, int addr) ; 00028 00029 ~VEML6040() ; 00030 00031 /* 00032 * some member functions here (yet to be written) 00033 */ 00034 /** 00035 * get Red 00036 * @param none 00037 * @returns float value of Red 00038 */ 00039 float getR(void) ; // return float value of Red 00040 00041 /** 00042 * get Green 00043 * @param none 00044 * @returns float value of Green 00045 */ 00046 float getG(void) ; // return float value of Green 00047 00048 /** 00049 * get Blue 00050 * @param none 00051 * @returns float value of Blue 00052 */ 00053 float getB(void) ; // return float value of Blue 00054 00055 /** 00056 * get White 00057 * @param none 00058 * @returns float value of White 00059 */ 00060 float getW(void) ; // return float value of White 00061 00062 /** 00063 * get CCT(McCAMY FORMULA) value X 00064 * @param none 00065 * @returns float CCT value X 00066 */ 00067 float getX(void) ; // return float value of X 00068 00069 /** 00070 * get CCT(McCAMY FOMULA) value Y 00071 * @param none 00072 * @returns float CCT value Y 00073 */ 00074 float getY(void) ; // return float value of Y 00075 00076 /** 00077 * get CCT(McCAMY FOMULA) value Z 00078 * @param none 00079 * @returns float CCT value Z 00080 */ 00081 float getZ(void) ; // return float value of Z 00082 00083 /** 00084 * get CIE1931 X 00085 * @param none 00086 * @returns float CIE1931 X 00087 */ 00088 float getCIEX(void) ; // return float value of CIE1931_x 00089 00090 /** 00091 * get CIE1931 Y 00092 * @param none 00093 * @returns float CIE1931 Y 00094 */ 00095 float getCIEY(void) ; // return float value of CIE1931_y 00096 00097 void getCOLORConf(uint8_t *colorconf) ; 00098 void setCOLORConf(uint8_t colorconf) ; 00099 // void getX(uint8_t *X) ; 00100 // void getY(uint8_t *Y) ; 00101 // void getZ(uint8_t *Z) ; 00102 /** 00103 * get raw Red data 00104 * @param uint16_t *rdata 00105 * @returns i2c status 0: success non-0: failure 00106 */ 00107 int getRData(uint16_t *rdata) ; 00108 00109 /** 00110 * get raw Green data 00111 * @param uint16_t *gdata 00112 * @returns i2c status 0: success non-0: failure 00113 */ 00114 int getGData(uint16_t *gdata) ; 00115 00116 /** 00117 * get raw Blue data 00118 * @param uint16_t *bdata 00119 * @returns i2c status 0: success non-0: failure 00120 */ 00121 int getBData(uint16_t *bdata) ; 00122 00123 /** 00124 * get raw White data 00125 * @param uint16_t *wdata 00126 * @returns i2c status 0: success non-0: failure 00127 */ 00128 int getWData(uint16_t *wdata) ; 00129 00130 // void getCCTiData(uint16_t *cctidata) ; 00131 /** 00132 * get CCTi data for CCT (EMPIRICAL APPROACH) 00133 * @param none 00134 * @returns float CCTi data 00135 */ 00136 float getCCTiData(void) ; 00137 // void getCCTData(uint16_t *cctdata) ; 00138 00139 /** 00140 * get CCT data (EMPIRICAL APPROACH) 00141 * @param none 00142 * @returns float CCD data 00143 */ 00144 float getCCTData(void) ; 00145 // void cmdRead(uint8_t cmd, uint8_t *data, uint8_t numdata = 2) ; 00146 // void cmdWrite(uint8_t cmd, uint8_t *data, uint8_t numdata = 2) ; 00147 00148 private: 00149 I2C m_i2c; 00150 int m_addr; 00151 int readRegs(int addr, uint8_t * data, int len); 00152 int writeRegs(uint8_t * data, int len); 00153 } ; 00154 #endif /* _VEML6040_H_ */
Generated on Thu Jul 14 2022 06:24:36 by
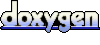