
pwm period is now 200us instead of the default 20ms veml6040 config is now AF_BIT | TRIG_BIT
Dependencies: mbed MMA8451Q USBDevice WakeUp vt100
Fork of afero_node_suntory_2017_06_15 by
USPool.hpp
00001 #ifndef _ULTRA_SIMPLE_POOL_ 00002 #define _ULTRA_SIMPLE_POOL_ 00003 00004 template<typename T> class USPool 00005 { 00006 private: bool pool_usage[]; 00007 private: T pool[]; 00008 private: int len; 00009 public: USPool(T init, int _size) 00010 { 00011 pool_usage = new bool[_size]; 00012 pool = new pool[_size]; 00013 len = _size; 00014 for(int i=0; i<len; ++i) 00015 { 00016 pool_usage[i] = false; 00017 pool[i] = init; 00018 } 00019 } 00020 public: ~USPool() 00021 { 00022 delete[] pool; 00023 pool = NULL; 00024 delete[] pool_usage; 00025 pool_usage = NULL; 00026 } 00027 public: T obtain(T unavail) 00028 { 00029 T ret = unavail; 00030 for(int i=0; i<len; ++i) 00031 { 00032 if(pool_usage[i] == false) 00033 { 00034 pool_usage[i] = true; 00035 ret = pool[i]; 00036 break; 00037 } 00038 } 00039 return ret; 00040 } 00041 public: void release(T *released) 00042 { 00043 for(int i=0; i<len; ++i) 00044 { 00045 if(pool[i] == released) 00046 { 00047 pool_usage[i] = false; 00048 } 00049 } 00050 } 00051 }; 00052 00053 #endif //_ULTRA_SIMPLE_POOL_
Generated on Thu Jul 14 2022 06:24:36 by
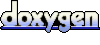