
pwm period is now 200us instead of the default 20ms veml6040 config is now AF_BIT | TRIG_BIT
Dependencies: mbed MMA8451Q USBDevice WakeUp vt100
Fork of afero_node_suntory_2017_06_15 by
StatusCommand.h
00001 /** 00002 * Copyright 2015 Afero, Inc. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef STATUS_COMMAND_H__ 00018 #define STATUS_COMMAND_H__ 00019 00020 #include "mbed.h" 00021 #include "DebugIO.hpp" 00022 00023 class StatusCommand { 00024 public: 00025 00026 StatusCommand(); 00027 00028 StatusCommand(uint16_t bytesToSend); 00029 00030 ~StatusCommand(); 00031 00032 uint16_t getSize(); 00033 00034 uint16_t getBytes(int *bytes); 00035 00036 uint8_t calcChecksum(); 00037 00038 void setChecksum(uint8_t checksum); 00039 00040 uint8_t getChecksum(); 00041 00042 void setAck(bool ack); 00043 00044 void setBytesToSend(uint16_t bytesToSend); 00045 00046 uint16_t getBytesToSend(); 00047 00048 void setBytesToRecv(uint16_t bytesToRecv); 00049 00050 uint16_t getBytesToRecv(); 00051 00052 bool equals(StatusCommand *statusCommand); 00053 00054 bool isValid(); 00055 00056 void dump(); 00057 00058 void dumpBytes(); 00059 00060 private: 00061 00062 uint8_t _cmd; 00063 uint16_t _bytesToSend; 00064 uint16_t _bytesToRecv; 00065 uint8_t _checksum; 00066 }; 00067 00068 #endif // STATUS_COMMAND_H__
Generated on Thu Jul 14 2022 06:24:36 by
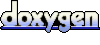