
pwm period is now 200us instead of the default 20ms veml6040 config is now AF_BIT | TRIG_BIT
Dependencies: mbed MMA8451Q USBDevice WakeUp vt100
Fork of afero_node_suntory_2017_06_15 by
Preferences.hpp
00001 #ifndef _PREFERENCES_HPP_ 00002 #define _PREFERENCES_HPP_ 00003 00004 #include "mbed.h" 00005 00006 typedef union 00007 { 00008 bool b; 00009 int16_t i_16; 00010 uint16_t ui_16; 00011 struct 00012 { 00013 short X, Y, Z; 00014 }; 00015 struct 00016 { 00017 uint16_t R, G, B; 00018 }; 00019 } VAR; 00020 00021 template<typename A> struct SENSORS_BASE 00022 { 00023 enum INDEX 00024 { 00025 ACCELEROMETER = 0, 00026 COLOR, 00027 PRESSURE, 00028 CURRENT_TRANS, 00029 TEMPERATURE, 00030 SIZE, 00031 }; 00032 static bool ACTIVE[SIZE]; 00033 static bool DELTA[SIZE]; 00034 }; 00035 00036 struct SENSORS : public SENSORS_BASE<SENSORS> 00037 { 00038 template<typename A> struct COMMONPARAMS; 00039 struct MAX44008; 00040 struct VEML6040; 00041 struct MMA8451Q; 00042 template<typename A> struct SEMITEC_502AT_11_CALIB; 00043 template<typename A> struct DEV_TEMP; 00044 struct DEV_TEMP_INST; 00045 struct SEMITEC_502AT_11; 00046 struct SR_3702_150N_14Z; 00047 template<typename A> struct KEYENCE_PRESSURE_COMMON_PARAMS; 00048 struct AP_53A_KEYENCE; //AP-53A KEYENCE gas 00049 struct GP_M010_KEYENCE; //"GP-M010 KEYENCE" liquid 00050 struct NXP_LM75B; 00051 }; 00052 00053 template<typename A> struct SENSORS::COMMONPARAMS 00054 { 00055 static char* TYPE; 00056 static char* PN; 00057 static char* UNIT; 00058 static char* JSON_FMT; 00059 }; 00060 00061 template<typename A> struct SENSORS::KEYENCE_PRESSURE_COMMON_PARAMS 00062 { 00063 static float V_REF; 00064 static float PRESSURE_INTERVAL; 00065 static float SHUNT_R; 00066 static float LOWEST_CUR; 00067 static float PRESSURE_COEF; 00068 }; 00069 00070 template<typename A> float SENSORS::KEYENCE_PRESSURE_COMMON_PARAMS<A>::V_REF = 3.3; 00071 template<typename A> float SENSORS::KEYENCE_PRESSURE_COMMON_PARAMS<A>::PRESSURE_INTERVAL = 1.0; 00072 template<typename A> float SENSORS::KEYENCE_PRESSURE_COMMON_PARAMS<A>::SHUNT_R = 165; 00073 template<typename A> float SENSORS::KEYENCE_PRESSURE_COMMON_PARAMS<A>::LOWEST_CUR = 0.004; 00074 template<typename A> float SENSORS::KEYENCE_PRESSURE_COMMON_PARAMS<A>::PRESSURE_COEF = 62500; 00075 00076 struct SENSORS::MAX44008 : public SENSORS::COMMONPARAMS<SENSORS::MAX44008> 00077 { 00078 enum 00079 { 00080 ADDRESS = 0x41, 00081 AMB_CONFIG = 0x00, // most sensitive gain 00082 RAW_MODE = 0x20, // MODE_CLEAR_RGB_IR 00083 PWM_LED_B = 0x96 00084 }; 00085 struct TRIM 00086 { 00087 enum 00088 { 00089 R = 0x50, 00090 G = 0x01, 00091 B = 0x20 00092 }; 00093 }; 00094 }; 00095 00096 template<> char* SENSORS::COMMONPARAMS<SENSORS::MAX44008>::TYPE = "COLOR"; 00097 template<> char* SENSORS::COMMONPARAMS<SENSORS::MAX44008>::PN = "MAX44008"; 00098 template<> char* SENSORS::COMMONPARAMS<SENSORS::MAX44008>::UNIT = "mW/cm2"; 00099 template<> char* SENSORS::COMMONPARAMS<SENSORS::MAX44008>::JSON_FMT( 00100 "{\"DEVICE\":\"%s\",\"PN\":\"%s\",\"DATA\":[{\"TIME\":\"%ld\",\"VAL\":\"%d\",\"UNIT\":\"%s\"}]}" 00101 ); 00102 00103 00104 struct SENSORS::VEML6040 : public SENSORS::COMMONPARAMS<SENSORS::VEML6040> 00105 { 00106 enum 00107 { 00108 ADDRESS = 0x10, 00109 AMB_CONFIG = 0x00, // most sensitive gain 00110 RAW_MODE = 0x20, // MODE_CLEAR_RGB_IR 00111 PWM_LED_B = 0xFA, 00112 PWM_LED_R_UI16 = 0x5FA2, 00113 PWM_LED_G_UI16 = 0xB09B, 00114 PWM_LED_B_UI16 = 0x83EF 00115 }; 00116 struct TRIM 00117 { 00118 enum 00119 { 00120 R = 0x50, 00121 G = 0x01, 00122 B = 0x20 00123 }; 00124 }; 00125 }; 00126 00127 template<> char* SENSORS::COMMONPARAMS<SENSORS::VEML6040>::JSON_FMT( 00128 "{\"DEVICE\":\"COLOR\",\"PN\":\"VEML6040\",\"VAL_R\":\"%d\",\"VAL_G\":\"%d\",\"VAL_B\":\"%d\",\"UNIT\":\"mW/cm2\",\"S\":\"%d\",\"E\":\"%d\"}" 00129 ); 00130 00131 struct SENSORS::MMA8451Q : public SENSORS::COMMONPARAMS<SENSORS::MMA8451Q> 00132 { 00133 enum 00134 { 00135 #if defined (TARGET_KL25Z) 00136 ADDRESS = 0x1D 00137 #elif defined (TARGET_TEENSY3_1) 00138 ADDRESS = 0x1C 00139 #endif 00140 }; 00141 }; 00142 00143 template<> char* SENSORS::COMMONPARAMS<SENSORS::MMA8451Q>::JSON_FMT( 00144 // "{\"DEVICE\":\"ACCEL\",\"PN\":\"MMA8451Q\",\"VAL_X\":\"%.3f\",\"VAL_Y\":\"%.3f\",\"VAL_Z\":\"%.3f\",\"UNIT\":\"g\",\"N\":\"%d\",\"RET\":\"%d\"}" 00145 "{\"DEVICE\":\"ACCEL\",\"PN\":\"MMA8451Q\",\"VAL_X\":\"%.3f\",\"VAL_Y\":\"0\",\"VAL_Z\":\"0\",\"UNIT\":\"g\",\"S\":\"%d\",\"E\":\"%d\"}" 00146 ); 00147 00148 template<typename A> struct SENSORS::SEMITEC_502AT_11_CALIB 00149 { 00150 static float B; 00151 static float T0; 00152 static float R0; 00153 static float R1; 00154 }; 00155 template<> float SENSORS::SEMITEC_502AT_11_CALIB<SENSORS::SEMITEC_502AT_11>::B = 3324; 00156 template<> float SENSORS::SEMITEC_502AT_11_CALIB<SENSORS::SEMITEC_502AT_11>::T0 = 298.15; 00157 template<> float SENSORS::SEMITEC_502AT_11_CALIB<SENSORS::SEMITEC_502AT_11>::R0 = 5.0; //kOhm 00158 template<> float SENSORS::SEMITEC_502AT_11_CALIB<SENSORS::SEMITEC_502AT_11>::R1 = 4.95; //kOhm 00159 00160 template<typename A> struct SENSORS::DEV_TEMP 00161 { 00162 enum 00163 { 00164 TUBE = 0, 00165 BEFORE_COOLING, 00166 AFTER_COOLING, 00167 SIZE 00168 }; 00169 static char* JSON_FMT; 00170 static char* SUFFIXES[SIZE]; 00171 static char* PART_NUMBERS[SIZE]; 00172 static char* POSITION[SIZE]; 00173 static char* STATUS[SIZE]; 00174 }; 00175 00176 struct SENSORS::DEV_TEMP_INST : 00177 public SENSORS::DEV_TEMP<SENSORS::DEV_TEMP_INST> 00178 { 00179 }; 00180 00181 template<> char* SENSORS::DEV_TEMP<SENSORS::DEV_TEMP_INST>::SUFFIXES[SIZE] = { 00182 "01", "02", "03" 00183 }; 00184 00185 template<> char* SENSORS::DEV_TEMP<SENSORS::DEV_TEMP_INST>::POSITION[SIZE] = { 00186 "NOT COOLED", "NOT COOLED", "BEING COOLED" 00187 }; 00188 00189 template<> char* SENSORS::DEV_TEMP<SENSORS::DEV_TEMP_INST>::STATUS[SIZE] = { 00190 "TUBE", "IN", "IN" 00191 }; 00192 00193 template<> char* SENSORS::DEV_TEMP<SENSORS::DEV_TEMP_INST>::PART_NUMBERS[SIZE] = { 00194 "LM75B", "SEMITEC 502AT-11", "SEMITEC 502AT-11" 00195 }; 00196 00197 template<> char* SENSORS::DEV_TEMP<SENSORS::DEV_TEMP_INST>::JSON_FMT( 00198 // "{\"DEVICE\":\"TEMP%s\",\"PN\":\"%s\",\"VAL\":\"%0.1f\",\"UNIT\":\"degC\"}" 00199 // "{\"DEVICE\":\"TEMP%s\",\"PN\":\"%s\",\"POS\":\"%s\",\"STAT\":\"%s\",\"VAL\":\"%0.1f\",\"UNIT\":\"degC\"}" 00200 "{\"DEVICE\":\"TEMP%s\",\"PN\":\"%s\",\"POS\":\"%s\",\"STAT\":\"%s\",\"VAL\":\"%0.1f\",\"UNIT\":\"degC\",\"S\":\"%d\",\"E\":\"%d\"}" 00201 ); 00202 00203 struct SENSORS::SEMITEC_502AT_11 : 00204 public SENSORS::COMMONPARAMS<SENSORS::SEMITEC_502AT_11>, 00205 public SENSORS::SEMITEC_502AT_11_CALIB<SENSORS::SEMITEC_502AT_11> 00206 { 00207 }; 00208 00209 //template<> char* SENSORS::COMMONPARAMS<SENSORS::SEMITEC_502AT_11>::TYPE = "TEMP"; 00210 template<> char* SENSORS::COMMONPARAMS<SENSORS::SEMITEC_502AT_11>::PN = "SEMITEC 502AT-11"; 00211 //template<> char* SENSORS::COMMONPARAMS<SENSORS::SEMITEC_502AT_11>::UNIT = "degC"; 00212 00213 //template<> char* SENSORS::COMMONPARAMS<SENSORS::SEMITEC_502AT_11>::JSON_FMT( 00214 // "{\"DEVICE\":\"%s\",\"PN\":\"%s\",\"DATA\":[{\"TIME\":\"%ld\",\"VAL\":\"%0.1f\",\"UNIT\":\"%s\",\"POS\":\"%s\"}]}" 00215 //); 00216 00217 struct SENSORS::NXP_LM75B : 00218 public SENSORS::COMMONPARAMS<SENSORS::NXP_LM75B> 00219 { 00220 enum 00221 { 00222 ADDRESS = 0x48, 00223 REG_Conf = 0x01, 00224 REG_Temp = 0x00, 00225 REG_Tos = 0x03, 00226 REG_Thyst = 0x02 00227 }; 00228 }; 00229 00230 template<> char* SENSORS::COMMONPARAMS<SENSORS::NXP_LM75B>::PN = "LM75B"; 00231 00232 struct SENSORS::SR_3702_150N_14Z : public SENSORS::COMMONPARAMS<SENSORS::SR_3702_150N_14Z>{}; 00233 00234 template<> char* SENSORS::COMMONPARAMS<SENSORS::SR_3702_150N_14Z>::TYPE = "CURRENT_TRANS";; 00235 template<> char* SENSORS::COMMONPARAMS<SENSORS::SR_3702_150N_14Z>::PN = "SR-3702-150N/14Z"; 00236 template<> char* SENSORS::COMMONPARAMS<SENSORS::SR_3702_150N_14Z>::UNIT = "V"; 00237 template<> char* SENSORS::COMMONPARAMS<SENSORS::SR_3702_150N_14Z>::JSON_FMT( 00238 "{\"DEVICE\":\"%s\",\"PN\":\"%s\",\"DATA\":[{\"TIME\":\"%ld\",\"VAL\":\"%0.1f\",\"UNIT\":\"%s\"}]}" 00239 ); 00240 struct SENSORS::AP_53A_KEYENCE : 00241 public SENSORS::COMMONPARAMS<SENSORS::AP_53A_KEYENCE>, 00242 public SENSORS::KEYENCE_PRESSURE_COMMON_PARAMS<SENSORS::AP_53A_KEYENCE> {}; 00243 template<> char *SENSORS::COMMONPARAMS<SENSORS::AP_53A_KEYENCE>::TYPE = "GAS PRESSURE"; 00244 template<> char *SENSORS::COMMONPARAMS<SENSORS::AP_53A_KEYENCE>::PN = "AP-53A KEYENCE"; 00245 template<> char *SENSORS::COMMONPARAMS<SENSORS::AP_53A_KEYENCE>::UNIT = "KPa"; 00246 template<> char* SENSORS::COMMONPARAMS<SENSORS::AP_53A_KEYENCE>::JSON_FMT( 00247 //"{\"DEVICE\":\"%s\",\"PN\":\"%s\",\"DATA\":[{\"TIME\":\"%ld\",\"VAL\":\"%0.1f\",\"UNIT\":\"%s\"}]}" 00248 "{\"DEVICE\":\"%s\",\"PN\":\"%s\",\"VAL\":\"%0.1f\"}" 00249 ); 00250 00251 struct SENSORS::GP_M010_KEYENCE : 00252 public SENSORS::COMMONPARAMS<SENSORS::GP_M010_KEYENCE>, 00253 public SENSORS::KEYENCE_PRESSURE_COMMON_PARAMS<SENSORS::GP_M010_KEYENCE> {}; 00254 template<> char *SENSORS::COMMONPARAMS<SENSORS::GP_M010_KEYENCE>::TYPE = "LIQUID PRESSURE"; 00255 template<> char *SENSORS::COMMONPARAMS<SENSORS::GP_M010_KEYENCE>::PN = "AGP-M010 KEYENCE"; 00256 template<> char *SENSORS::COMMONPARAMS<SENSORS::GP_M010_KEYENCE>::UNIT = "KPa"; 00257 template<> char* SENSORS::COMMONPARAMS<SENSORS::GP_M010_KEYENCE>::JSON_FMT( 00258 //"{\"DEVICE\":\"%s\",\"PN\":\"%s\",\"DATA\":[{\"TIME\":\"%ld\",\"VAL\":\"%0.1f\",\"UNIT\":\"%s\"}]}" 00259 "{\"DEVICE\":\"%s\",\"PN\":\"%s\",\"VAL\":\"%0.1f\"}" 00260 ); 00261 00262 struct PINS 00263 { 00264 struct LED_4_COLOR; 00265 struct COLOR; 00266 struct ACCELEROMETER; 00267 struct CURRENT_TRANS; 00268 struct TEMPERATURE; 00269 struct UART; 00270 struct ASR_1; 00271 struct GAS; 00272 struct LIQUID; 00273 }; 00274 00275 struct PINS::LED_4_COLOR 00276 { 00277 enum 00278 { 00279 R = PTA5, 00280 G = PTA4, 00281 B = PTA12 00282 }; 00283 }; 00284 00285 struct PINS::COLOR 00286 { 00287 enum 00288 { 00289 SDA = PTE0, 00290 SCL = PTE1 00291 }; 00292 }; 00293 00294 struct PINS::ACCELEROMETER 00295 { 00296 enum 00297 { 00298 #if defined (TARGET_KL25Z) 00299 SDA = PTE25, 00300 SCL = PTE24 00301 #elif defined (TARGET_TEENSY3_1) 00302 SDA = PTB3, 00303 SCL = PTB2 00304 #endif 00305 }; 00306 }; 00307 00308 struct PINS::CURRENT_TRANS 00309 { 00310 enum 00311 { 00312 AIN = PTB0 00313 }; 00314 }; 00315 00316 struct PINS::TEMPERATURE 00317 { 00318 enum 00319 { 00320 BEFORE_COOLING = PTB0, // la suno shield 00321 // BEFORE_COOLING = PTC2, // Hibiki only 00322 AFTER_COOLING = PTB1, 00323 SDA = PTE0, 00324 SCL = PTE1 00325 }; 00326 }; 00327 00328 struct PINS::GAS 00329 { 00330 enum 00331 { 00332 AIN = PTB2 00333 }; 00334 }; 00335 00336 struct PINS::LIQUID 00337 { 00338 enum 00339 { 00340 AIN = PTB3 00341 }; 00342 }; 00343 00344 struct PINS::ASR_1 00345 { 00346 struct SPI; 00347 enum 00348 { 00349 #if defined (TARGET_KL25Z) 00350 RESET = PTC9 //PSOC 00351 //RESET = PTA20 00352 #elif defined (TARGET_TEENSY3_1) 00353 RESET = PTD6 00354 #endif 00355 }; 00356 struct SIG 00357 { 00358 template<typename A> struct RESET_BASE 00359 { 00360 static time_t INTERVAL; 00361 }; 00362 struct RESET : public RESET_BASE<RESET> 00363 { 00364 enum 00365 { 00366 ASSERT = 0, 00367 DEASSERT = 1 00368 }; 00369 }; 00370 }; 00371 }; 00372 00373 template<> time_t PINS::ASR_1::SIG::RESET_BASE<PINS::ASR_1::SIG::RESET>::INTERVAL = 60*5; 00374 00375 struct PINS::ASR_1::SPI 00376 { 00377 enum 00378 { 00379 #if defined (TARGET_KL25Z) 00380 MOSI = PTD2, 00381 MISO = PTD3, 00382 SCK = PTD1, 00383 CS = PTD0, 00384 SR = PTD4 // request from slave 00385 #elif defined (TARGET_TEENSY3_1) 00386 MOSI = PTC6, 00387 MISO = PTC7, 00388 SCK = PTC5, 00389 CS = PTC4, 00390 SR = PTD1 // request from slave 00391 #endif 00392 }; 00393 struct SIG 00394 { 00395 struct CS 00396 { 00397 enum 00398 { 00399 ASSERT = 0, 00400 DEASSERT = 1 00401 }; 00402 }; 00403 }; 00404 enum 00405 { 00406 NUM_BITS_PER_FRAME = 8, 00407 MODE_0 = 0, 00408 FREQUENCY = 1*1000*1000 00409 }; 00410 }; 00411 00412 struct PINS::UART 00413 { 00414 enum 00415 { 00416 BAUD_RATE = 115200 00417 }; 00418 #if defined (TARGET_TEENSY3_1) 00419 enum 00420 { 00421 uart0_tx = PTA2, 00422 uart0_rx = PTA1 00423 }; 00424 #endif 00425 #if defined (TARGET_KL25Z) && (WICED_SMART_UART_CONNECTION) 00426 struct WICED 00427 { 00428 struct TAG3 00429 { 00430 enum 00431 { 00432 TO_RX = PTE22 // -> TAG3 J7-1 00433 ,TO_TX = PTE23 // -> TAG3 J8-5 00434 //power 3v3 -> TAG J9-1 00435 //GND -> TAGJ9-4 00436 }; 00437 }; 00438 }; 00439 #endif 00440 }; 00441 00442 template<typename A> struct PREFERENCES_BASE 00443 { 00444 static int32_t SENSING_INTERVAL[SENSORS::SIZE]; 00445 static time_t EPOCH_UTC; 00446 typedef union 00447 { 00448 uint32_t ui32; 00449 uint8_t ui8[sizeof(uint32_t)]; 00450 } _crc32; 00451 static _crc32 CRC32; 00452 static bool FLOW_CONTROL; 00453 static bool DBG_PRINT_ENABLED; 00454 static bool DBG_PRINT_ASR_ENABLED; 00455 struct AFERO_ATTRIBUTE 00456 { 00457 uint32_t ATTR_CRC32; 00458 }; 00459 }; 00460 00461 struct PREFERENCES : PREFERENCES_BASE<PREFERENCES> 00462 { 00463 struct NOTIFICATION; 00464 struct DATA_TRANSFER_MODE 00465 { 00466 enum 00467 { 00468 JSON = 0, 00469 BASE64 = 1 00470 }; 00471 }; 00472 struct THRESHOLD 00473 { 00474 struct RANGE 00475 { 00476 enum 00477 { 00478 MIN = 0, 00479 MAX, 00480 DELTA, 00481 SIZE 00482 }; 00483 }; 00484 }; 00485 template<typename A> struct NOTIFICATION_BASE 00486 { 00487 static bool ENABLED[SENSORS::SIZE][THRESHOLD::RANGE::SIZE]; 00488 static VAR THRESHOLD[SENSORS::SIZE][THRESHOLD::RANGE::SIZE]; // not used ... for now. 00489 }; 00490 }; 00491 00492 template<> int32_t PREFERENCES_BASE<PREFERENCES>::SENSING_INTERVAL[SENSORS::SIZE] = { 30, 30, 30, 30, 30 }; 00493 template<> time_t PREFERENCES_BASE<PREFERENCES>::EPOCH_UTC = 0; 00494 template<> PREFERENCES_BASE<PREFERENCES>::_crc32 PREFERENCES_BASE<PREFERENCES>::CRC32 = { 0 }; 00495 00496 template<> bool PREFERENCES_BASE<PREFERENCES>::FLOW_CONTROL = false; 00497 00498 template<> bool PREFERENCES_BASE<PREFERENCES>::DBG_PRINT_ENABLED = true; 00499 template<> bool PREFERENCES_BASE<PREFERENCES>::DBG_PRINT_ASR_ENABLED = true; 00500 00501 struct PREFERENCES::NOTIFICATION : public PREFERENCES::NOTIFICATION_BASE<PREFERENCES::NOTIFICATION> 00502 { 00503 }; 00504 00505 template<> bool PREFERENCES::NOTIFICATION_BASE<PREFERENCES::NOTIFICATION>::ENABLED[SENSORS::SIZE][THRESHOLD::RANGE::SIZE]; 00506 template<> VAR PREFERENCES::NOTIFICATION_BASE<PREFERENCES::NOTIFICATION>::THRESHOLD[SENSORS::SIZE][THRESHOLD::RANGE::SIZE]; 00507 00508 typedef union 00509 { 00510 typedef struct 00511 { 00512 time_t timeStamp; 00513 SENSORS::INDEX index; 00514 } PACKET_BASE; 00515 struct : PACKET_BASE 00516 { 00517 short x,y,z; 00518 } accelerometer; 00519 struct : PACKET_BASE 00520 { 00521 uint16_t R, G, B; 00522 } color; 00523 struct : PACKET_BASE 00524 { 00525 float val; 00526 } temperature; 00527 struct : PACKET_BASE 00528 { 00529 float val; 00530 } current_trans; 00531 struct : PACKET_BASE 00532 { 00533 float val; 00534 } pressure; 00535 } PACKET; 00536 00537 //template<> bool SENSORS_BASE<SENSORS>::ACTIVE[SENSORS::SIZE] = { false, false, false, false, true }; // temperature 00538 //template<> bool SENSORS_BASE<SENSORS>::ACTIVE[SENSORS::SIZE] = { false, true, false, false, false }; // color 00539 template<> bool SENSORS_BASE<SENSORS>::ACTIVE[SENSORS::SIZE] = { true, true, false, false, true }; // color + temp + accelerometer 00540 //template<> bool SENSORS_BASE<SENSORS>::ACTIVE[SENSORS::SIZE] = { true, false, false, false, true }; // temp + accelerometer 00541 //template<> bool SENSORS_BASE<SENSORS>::ACTIVE[SENSORS::SIZE] = { false, true, false, false, true }; // color + temp 00542 //template<> bool SENSORS_BASE<SENSORS>::ACTIVE[SENSORS::SIZE] = { false, true, false, true, true }; // sensor box 00543 //template<> bool SENSORS_BASE<SENSORS>::ACTIVE[SENSORS::SIZE] = { false, false, true, false, false }; // pressure 00544 //template<> bool SENSORS_BASE<SENSORS>::ACTIVE[SENSORS::SIZE] = { true, false, false, false, false }; // accelerometer 00545 //template<> bool SENSORS_BASE<SENSORS>::ACTIVE[SENSORS::SIZE] = { false, true, false, false, false }; // color only ... sensor box 00546 //template<> bool SENSORS_BASE<SENSORS>::ACTIVE[SENSORS::SIZE] = { false, false, false, true, false }; // CT only ... sensor box 00547 00548 template<> bool SENSORS_BASE<SENSORS>::DELTA[SENSORS::SIZE] = { true, false, false, false, false }; // accelerometer 00549 00550 #endif //_PREFERENCES_HPP_
Generated on Thu Jul 14 2022 06:24:36 by
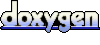