
pwm period is now 200us instead of the default 20ms veml6040 config is now AF_BIT | TRIG_BIT
Dependencies: mbed MMA8451Q USBDevice WakeUp vt100
Fork of afero_node_suntory_2017_06_15 by
MCUResetReason.cpp
00001 #include "MCUResetReason.hpp" 00002 00003 /** 00004 * System Reset Status Register 0 (RCM_SRS0) 0x4007_F000 00005 * 00006 * bit[7] : POR Power-On Reset 00007 * bit[6] : PIN External Reset Pin 00008 * bit[5] : WDOG Watchdog 00009 * bit[4] : (Reserved) 00010 * bit[3] : LOL Loss-of-Lock Reset 00011 * bit[2] : LOC Loss-of-Clock Reset 00012 * bit[1] : LVD Low-Voltage Detect Reset 00013 * bit[0] : WAKEUP Low Leakage Wakeup Reset 00014 */ 00015 #define REG_RCM_SRS0 (uint8_t *)0x4007F000 00016 #define POR_RESET_BIT 0x80 00017 #define PIN_RESET_BIT 0x40 00018 #define WDG_RESET_BIT 0x20 00019 #define LOL_RESET_BIT 0x08 00020 #define LOC_RESET_BIT 0x04 00021 #define LVD_RESET_BIT 0x02 00022 #define WUP_RESET_BIT 0x01 00023 /** 00024 * System Reset Status Register 1 (RCM_SRS1) 0x4007_F001 00025 * 00026 * bit[7:6] (Reserved) 00027 * bit[5] : SACKERR Stop Mode Acknowledge Error Reset 00028 * bit[4] : (Reserved) 00029 * bit[3] : MDM_AP MDM-AP System Reset Request 00030 * bit[2] : SW Software Reset 00031 * bit[1] : LOCKUP Core Lockup 00032 * bit[0] : (Reserved) 00033 */ 00034 #define REG_RCM_SRS1 (uint8_t *)0x4007F001 00035 #define SACK_RESET_BIT 0x20 00036 #define MDM_RESET_BIT 0x08 00037 #define SW_RESET_BIT 0x04 00038 #define LOCKUP_RESET_BIT 0x02 00039 00040 /** 00041 * Software Reset 00042 * 00043 * From Cortex-M0 Devices Generic User Guide 00044 * 4.3.4 Application Interrupt and Reset Control Register 00045 * 00046 * Bit[31:16] : VECTCKEY 00047 * Bit[15] : ENDIANESS 00048 * Bit[14:3] : (Reserved) 00049 * Bit[2] : SYSRESETREQ 00050 * Bit[1] : VECTCLRACTIVE (reserved for debug use) 00051 * Bit[0] : (Reserved) 00052 * 00053 * Note: To trigger software reset, both VECTKEY=0x05FA and SYSRESETREQ 00054 * must be written at once, therefore the value will be 00055 * 0x05FA0004 00056 */ 00057 00058 MCUResetReason *self = NULL; 00059 00060 const char *MCUResetReason::str_reset_reason[] = { 00061 "Power On Reset", 00062 "External Pin Reset", 00063 "Watch Dog Reset : Forgot to feed?", 00064 "Loss of Lock Reset", 00065 "Loss of Clock Reset", 00066 "Low-Voltage Detect Reset", 00067 "Low Leakage Wakeup Reset", 00068 "Stop Mode Acknowledge Error Reset", 00069 "MDM-AP System Reset Request", 00070 "Software Reset", 00071 "Core Lockup Reset" 00072 }; 00073 00074 MCUResetReason::MCUResetReason() 00075 { 00076 SRS[0] = *REG_RCM_SRS0 ; 00077 SRS[1] = *REG_RCM_SRS1 ; 00078 } 00079 00080 MCUResetReason* MCUResetReason::ref() 00081 { 00082 return self != NULL ? self : self = new MCUResetReason(); 00083 } 00084 00085 void MCUResetReason::clearFlag() 00086 { 00087 SRS[0] = PIN_RESET_BIT; 00088 SRS[1] = 0; 00089 } 00090 00091 MCUResetReason::RESET_REASON MCUResetReason::getResetReason(void) 00092 { 00093 RESET_REASON reason; 00094 00095 if (SRS[0] & POR_RESET_BIT) { 00096 reason = POWER_ON; 00097 } else if (SRS[0] & PIN_RESET_BIT) { 00098 reason = EXTERNAL_PIN; 00099 } else if (SRS[0] & WDG_RESET_BIT) { 00100 reason = WATCHDOG; 00101 } else if (SRS[0] & LOL_RESET_BIT) { 00102 reason = LOSS_OF_LOCK; 00103 } else if (SRS[0] & LOC_RESET_BIT) { 00104 reason = LOSS_OF_CLOCK; 00105 } else if (SRS[0] & LVD_RESET_BIT) { 00106 reason = LOW_VOLTAGE_DETECT; 00107 } else if (SRS[0] & WUP_RESET_BIT) { 00108 reason = LOW_LEAKAGE_WAKEUP; 00109 } else if (SRS[1] & SACK_RESET_BIT) { 00110 reason = STOP_MODE_ACK_ERROR; 00111 } else if (SRS[1] & MDM_RESET_BIT) { 00112 reason = MDM_AP_SYSTEM_RESET_REQUEST; 00113 } else if (SRS[1] & SW_RESET_BIT) { 00114 reason = SOFTWARE; 00115 } else if (SRS[1] & LOCKUP_RESET_BIT) { 00116 reason = CORE_LOCKUP; 00117 } 00118 return reason; 00119 } 00120 00121 const char* MCUResetReason::getResetReasonStr() 00122 { 00123 return str_reset_reason[getResetReason()]; 00124 } 00125
Generated on Thu Jul 14 2022 06:24:36 by
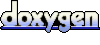