
pwm period is now 200us instead of the default 20ms veml6040 config is now AF_BIT | TRIG_BIT
Dependencies: mbed MMA8451Q USBDevice WakeUp vt100
Fork of afero_node_suntory_2017_06_15 by
LM75B.cpp
00001 #include "mbed.h" 00002 #include "LM75B.h" 00003 00004 /* Register list */ 00005 #define PTR_CONF 0x01 00006 #define PTR_TEMP 0x00 00007 #define PTR_TOS 0x03 00008 #define PTR_THYST 0x02 00009 00010 /* Configuration register */ 00011 /* B[7:5] : Reserved */ 00012 /* B[4:3] : OS_F_QUE[1:0] OS fault queue value */ 00013 #define CONFIG_QUE_1 0x00 00014 #define CONFIG_QUE_2 (0x01 << 3) 00015 #define CONFIG_QUE_4 (0x10 << 3) 00016 #define CONFIG_QUE_6 (0x11 << 3) 00017 /* B[2] : OS_POL 0 = OS active LOW, 1 = OS active HIGH */ 00018 #define CONFIG_OS_POL_L 0x00 00019 #define CONFIG_OS_POL_H (0x01 << 2) 00020 /* B[1] : OS_COMP_INT 0 = OS comparator, 1 = OS interrupt */ 00021 #define CONFIG_OS_COMP 0x00 00022 #define CONFIG_OS_INT (0x01 << 1) 00023 /* B[0] : SHUTDOWN 0 = normal, 1 = shutdown */ 00024 #define CONFIG_NORMARL 0x00 00025 #define CONFIG_SHUTDOWN 0x01 00026 00027 /* Temperature register */ 00028 /* D[15:5] = 11 bit data 0.125 * temp data */ 00029 /* D[4:0] : reserved */ 00030 00031 /* Tos register */ 00032 /* D[15:7] = 9 bit data */ 00033 /* D[6:0] : reserved */ 00034 00035 /* Thyst register */ 00036 /* D[15:7] = 9 ibt data */ 00037 /* D[6:0] : reserved */ 00038 00039 LM75B::LM75B(PinName sda, PinName scl, int addr) : m_i2c(sda, scl), m_addr(addr<<1) { 00040 m_i2c.frequency(I2C_FREQ_100KHZ); 00041 // activate the peripheral 00042 } 00043 00044 LM75B::~LM75B() { } 00045 00046 int8_t LM75B::temp(void) 00047 { 00048 char t[1] = { 0x00 } ; 00049 int8_t temp ; 00050 m_i2c.write(m_addr, t, 1, true) ; 00051 m_i2c.read(m_addr, t, 1) ; 00052 temp = (int8_t)t[0] ; 00053 return( temp ) ; 00054 } 00055 00056 void LM75B::getTemp(float *temp) 00057 { 00058 char t[2] ; 00059 int16_t iTemp = 0 ; 00060 m_i2c.read(m_addr, t, 2) ; /* read MSB, LSB */ 00061 iTemp = (t[0] << 8) | t[1] ; 00062 iTemp >>= 5 ; 00063 *temp = 0.125 * iTemp ; 00064 } 00065 00066 uint8_t LM75B::getConfig(uint8_t ptr_byte) 00067 { 00068 char config ; 00069 m_i2c.write(m_addr, (char*)(&ptr_byte), 1, true) ; 00070 m_i2c.read(m_addr, &config, 1) ; 00071 return( config ) ; 00072 } 00073 00074 void LM75B::setConfig(uint8_t ptr_byte, uint8_t config_data) 00075 { 00076 char t[2] ; 00077 t[0] = ptr_byte ; 00078 t[1] = config_data ; 00079 m_i2c.write(m_addr, t, 2, true) ; 00080 } 00081 00082 void LM75B::readRegs(int addr, uint8_t * data, int len) { 00083 char t[1] = {addr}; 00084 m_i2c.write(m_addr, t, 1, true); 00085 m_i2c.read(m_addr, (char *)data, len); 00086 } 00087 00088 void LM75B::writeRegs(uint8_t * data, int len) { 00089 m_i2c.write(m_addr, (char *)data, len); 00090 }
Generated on Thu Jul 14 2022 06:24:36 by
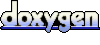