
pwm period is now 200us instead of the default 20ms veml6040 config is now AF_BIT | TRIG_BIT
Dependencies: mbed MMA8451Q USBDevice WakeUp vt100
Fork of afero_node_suntory_2017_06_15 by
GasPressure.cpp
00001 #include "GasPressure.hpp" 00002 00003 GasPressure::GasPressure() 00004 { 00005 ain = new AnalogIn((PinName)PINS::GAS::AIN); 00006 read(); 00007 } 00008 00009 GasPressure::~GasPressure() 00010 { 00011 delete ain; 00012 ain = NULL; 00013 } 00014 00015 float 00016 GasPressure::calc() 00017 { 00018 SERIAL_PRINT_DBG_FUNCNAME(); 00019 float &v_ref = SENSORS::AP_53A_KEYENCE::V_REF; 00020 float &shut_r = SENSORS::AP_53A_KEYENCE::SHUNT_R; 00021 float &lowest_cur = SENSORS::AP_53A_KEYENCE::LOWEST_CUR; 00022 float &pressure_coef = SENSORS::AP_53A_KEYENCE::PRESSURE_COEF; 00023 float &val = packet.pressure.val; 00024 float ret; 00025 short ret_s; 00026 ret = (v_ref * val / shut_r - lowest_cur) * pressure_coef; 00027 ret_s = ret *= 4096; 00028 ret /= ret_s / 4096; 00029 return ret < 0 ? 0 : ret; 00030 } 00031 00032 void 00033 GasPressure::toJSON(char* buf) 00034 { 00035 char* &fmt = SENSORS::AP_53A_KEYENCE::JSON_FMT; 00036 char* &type = SENSORS::AP_53A_KEYENCE::TYPE; 00037 char* &pn = SENSORS::AP_53A_KEYENCE::PN; 00038 char* &unit = SENSORS::AP_53A_KEYENCE::UNIT; 00039 SERIAL_PRINT_DBG_FUNCNAME(); 00040 time_t &time = packet.pressure.timeStamp; 00041 SERIAL_PRINT_DBG_FUNCNAME(); 00042 float f = calc(); 00043 SERIAL_PRINT_DBG("%f\n", f); 00044 // sprintf(buf,fmt,type,pn,time,f,unit); 00045 sprintf(buf,fmt,type,pn,f); 00046 } 00047 00048 void 00049 GasPressure::getBytes(uint8_t*) 00050 { 00051 } 00052 00053 void 00054 GasPressure::go() 00055 { 00056 SERIAL_PRINT_DBG_FUNCNAME(); 00057 timeout->attach(callback(this, &GasPressure::read), PREFERENCES::SENSING_INTERVAL[SENSORS::PRESSURE]); 00058 packet.pressure.val = ain->read(); 00059 SERIAL_PRINT_DBG_FUNCNAME(); 00060 RTC_GET_UTC(packet.pressure.timeStamp); 00061 ToDoQ::queuePut(this); 00062 backToNOP(); 00063 }
Generated on Thu Jul 14 2022 06:24:36 by
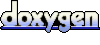