
pwm period is now 200us instead of the default 20ms veml6040 config is now AF_BIT | TRIG_BIT
Dependencies: mbed MMA8451Q USBDevice WakeUp vt100
Fork of afero_node_suntory_2017_06_15 by
Color_VEML6040.hpp
00001 #ifndef _MARUSOL_SENSOR_MANAGER_COLOR_VEML_6040_H_ 00002 #define _MARUSOL_SENSOR_MANAGER_COLOR_VEML_6040_H_ 00003 00004 #include "mbed.h" 00005 #include "VEML6040.h" 00006 #include "ToDoQ.h" 00007 #include "RTC_Handler.h" 00008 #include "TimeEventHandler.hpp" 00009 #include "Singletoned.hpp" 00010 #include "MCUResetReason.hpp" 00011 #include "WatchDogWrapper.hpp" 00012 00013 class Color_VEML6040 : 00014 public ToDo, 00015 public TimeEventHandler<Color_VEML6040>, 00016 public Singletoned<Color_VEML6040> 00017 { 00018 Timer *LED_Timer; 00019 Color_VEML6040(); 00020 ~Color_VEML6040(); 00021 VEML6040 *m_veml6040; 00022 PwmOut *LEDs[3]; 00023 void doLEDs(uint8_t R, uint8_t G, uint8_t B); 00024 void doLEDs_ui16(uint16_t R, uint16_t G, uint16_t B); 00025 void gogo(); 00026 void go(); 00027 void (Color_VEML6040::*callbackLED)(); 00028 void prepare_LED(); 00029 void registerForUpdate(); 00030 int32_t AHEAD_OF_TIME; 00031 class ResetSignal : public ToDo 00032 { 00033 bool bikkuri; 00034 public: 00035 ResetSignal(); 00036 virtual void toJSON(char* buf); 00037 virtual void getBytes(uint8_t*); 00038 }; 00039 ResetSignal rSignal; 00040 virtual void checkIntervalUpdate(); 00041 struct CountDownToSystemReset : 00042 public Singletoned<CountDownToSystemReset> 00043 { 00044 int count; 00045 public: 00046 friend class SignleToned; 00047 static void create(int count) 00048 { 00049 if(self != NULL) 00050 { 00051 delete self; 00052 } 00053 self = new CountDownToSystemReset(count); 00054 } 00055 CountDownToSystemReset(int count = 10) 00056 { 00057 this->count = count; 00058 } 00059 void countDown() 00060 { 00061 --count; 00062 if(count<=0) 00063 { 00064 WatchDogWrapper::getSelf()->kick_the_bucket(); 00065 count=0; 00066 } 00067 } 00068 }; 00069 public: 00070 friend class TimeEventHandler; 00071 friend class Singletoned; 00072 virtual void toJSON(char*); 00073 virtual void getBytes(uint8_t*); 00074 virtual void success(); 00075 }; 00076 00077 #endif //_MARUSOL_SENSOR_MANAGER_COLOR_VEML_6040_H_
Generated on Thu Jul 14 2022 06:24:36 by
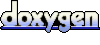