
pwm period is now 200us instead of the default 20ms veml6040 config is now AF_BIT | TRIG_BIT
Dependencies: mbed MMA8451Q USBDevice WakeUp vt100
Fork of afero_node_suntory_2017_06_15 by
Color_VEML6040.cpp
00001 #include "Color_VEML6040.hpp" 00002 00003 /* following 2 lines were added by Motoo Tanaka on 18-Oct-2017 */ 00004 #define AF_BIT 0x02 00005 #define TRIG_BIT 0x04 00006 00007 Color_VEML6040::Color_VEML6040() 00008 { 00009 CountDownToSystemReset::create(2); 00010 LED_Timer = new Timer(); 00011 AHEAD_OF_TIME = 1; 00012 LEDs[0] = new PwmOut((PinName)PINS::LED_4_COLOR::R) ; 00013 LEDs[1] = new PwmOut((PinName)PINS::LED_4_COLOR::G) ; 00014 LEDs[2] = new PwmOut((PinName)PINS::LED_4_COLOR::B) ; 00015 LEDs[0]->write(1.0) ; 00016 LEDs[1]->write(1.0) ; 00017 LEDs[2]->write(1.0) ; 00018 LEDs[0]->period_us(200) ; /* pwm period 200us */ 00019 LEDs[1]->period_us(200) ; /* pwm period 200us */ 00020 LEDs[2]->period_us(200) ; /* pwm period 200us */ 00021 m_veml6040 = new VEML6040((PinName)PINS::COLOR::SDA, (PinName)PINS::COLOR::SCL, SENSORS::VEML6040::ADDRESS); 00022 m_veml6040->setCOLORConf(0x00) ; /* default setting */ 00023 callbackLED = &Color_VEML6040::prepare_LED; 00024 int i = 0; 00025 for(;i<3;++i) 00026 { 00027 ToDoQ::queuePut(&rSignal); 00028 } 00029 read(); 00030 //interval_current = -1; 00031 } 00032 00033 Color_VEML6040::~Color_VEML6040() 00034 { 00035 delete LEDs[0]; 00036 LEDs[0] = NULL; 00037 delete LEDs[1]; 00038 LEDs[1] = NULL; 00039 delete LEDs[2]; 00040 LEDs[2] = NULL; 00041 delete m_veml6040; 00042 m_veml6040 = NULL; 00043 } 00044 00045 void 00046 Color_VEML6040::checkIntervalUpdate() 00047 { 00048 if( interval_current != PREFERENCES::SENSING_INTERVAL[SENSORS::COLOR]) 00049 { 00050 timeout->detach(); 00051 backToNOP(); 00052 callbackLED = &Color_VEML6040::prepare_LED; 00053 interval_current = PREFERENCES::SENSING_INTERVAL[SENSORS::COLOR]; 00054 int32_t time = interval_current <= 10 ? 10 : interval_current - AHEAD_OF_TIME; 00055 timeout->attach( callback( this, &Color_VEML6040::read), time ); 00056 SERIAL_PRINT_DBG("Color sensing interval updated : %d sec \n", time); 00057 } 00058 } 00059 00060 void 00061 Color_VEML6040::toJSON(char *buf) 00062 { 00063 if( 00064 m_veml6040->getRData(&packet.color.R) || 00065 m_veml6040->getGData(&packet.color.G) || 00066 m_veml6040->getBData(&packet.color.B) ) 00067 { 00068 CountDownToSystemReset::getSelf()->countDown(); 00069 char *fmt = "{\"DEVICE\":\"COLOR\",\"PN\":\"VEML6040\",\"VAL_R\":\"##\",\"VAL_G\":\"##\",\"VAL_B\":\"##\",\"UNIT\":\"mW/cm2\",\"S\":\"%d\",\"E\":\"%d\"}"; 00070 sprintf(buf,fmt,serialNum,error_count); 00071 } 00072 else 00073 { 00074 SERIAL_PRINT_DBG("RGB values read %.3f sec after LEDs are turned on\n", LED_Timer->read()); 00075 char* &fmt = SENSORS::VEML6040::JSON_FMT; 00076 uint16_t &r = packet.color.R; 00077 uint16_t &g = packet.color.G; 00078 uint16_t &b = packet.color.B; 00079 sprintf(buf,fmt,r,g,b,serialNum,error_count); 00080 } 00081 } 00082 00083 void 00084 Color_VEML6040::success() 00085 { 00086 doLEDs_ui16(0,0,0); 00087 SERIAL_PRINT_DBG("LED:TURNED OFF.\n"); 00088 ++serialNum; 00089 callbackLED = &Color_VEML6040::prepare_LED; 00090 int32_t delta = (int32_t)(LED_Timer->read()); 00091 LED_Timer->stop(); 00092 LED_Timer->reset(); 00093 timeout->attach(callback(this, &Color_VEML6040::read), interval_current < delta ? interval_current : (interval_current - delta) ); 00094 } 00095 00096 Color_VEML6040::ResetSignal::ResetSignal() 00097 { 00098 bikkuri = true; 00099 } 00100 00101 void 00102 Color_VEML6040::ResetSignal::toJSON(char *buf) 00103 { 00104 char *str; 00105 00106 if(bikkuri==true) 00107 { 00108 bikkuri = false; 00109 str = "{\"DEVICE\":\"COLOR\",\"PN\":\"VEML6040\",\"VAL_R\":\"%s\",\"VAL_G\":\"!!\",\"VAL_B\":\"!!\",\"UNIT\":\"!!\"}"; 00110 } else { 00111 bikkuri = true; 00112 str = "{\"DEVICE\":\"COLOR\",\"PN\":\"VEML6040\",\"VAL_R\":\"%s\",\"VAL_G\":\"**\",\"VAL_B\":\"**\",\"UNIT\":\"**\"}"; 00113 } 00114 00115 sprintf( 00116 buf, 00117 str, 00118 MCUResetReason::ref()->getResetReasonStr()); 00119 } 00120 00121 void 00122 Color_VEML6040::ResetSignal::getBytes(uint8_t *buf) 00123 { 00124 } 00125 00126 void 00127 Color_VEML6040::getBytes(uint8_t *buf) 00128 { 00129 00130 } 00131 00132 void 00133 Color_VEML6040::doLEDs(uint8_t R, uint8_t G, uint8_t B) 00134 { 00135 float fr, fg, fb ; 00136 fr = (float)(255-R)/255.0 ; 00137 fg = (float)(255-G)/255.0 ; 00138 fb = (float)(255-B)/255.0 ; 00139 LEDs[0]->write(fr) ; 00140 LEDs[1]->write(fg) ; 00141 LEDs[2]->write(fb) ; 00142 } 00143 00144 void 00145 Color_VEML6040::doLEDs_ui16(uint16_t R, uint16_t G, uint16_t B) 00146 { 00147 float fr, fg, fb ; 00148 fr = (float)(65535-R)/65535.0; 00149 fg = (float)(65535-G)/65535.0; 00150 fb = (float)(65535-B)/65535.0; 00151 LEDs[0]->write(fr) ; 00152 LEDs[1]->write(fg) ; 00153 LEDs[2]->write(fb) ; 00154 } 00155 00156 void 00157 Color_VEML6040::prepare_LED() 00158 { 00159 timeout->attach(callback(this,&Color_VEML6040::read),AHEAD_OF_TIME); 00160 callbackLED = &Color_VEML6040::registerForUpdate; //read_sensor_and_turn_off_LED; 00161 doLEDs_ui16( 00162 SENSORS::VEML6040::PWM_LED_R_UI16, 00163 SENSORS::VEML6040::PWM_LED_G_UI16, 00164 SENSORS::VEML6040::PWM_LED_B_UI16); 00165 m_veml6040->setCOLORConf(AF_BIT | TRIG_BIT) ; /* Force mode with Trigger */ 00166 SERIAL_PRINT_DBG("LED:TURNED ON. Timer start\n"); 00167 LED_Timer->start(); 00168 } 00169 00170 void 00171 Color_VEML6040::registerForUpdate() 00172 { 00173 ToDoQ::queuePut(this); 00174 } 00175 00176 void 00177 Color_VEML6040::go() 00178 { 00179 (this->*callbackLED)(); 00180 backToNOP(); 00181 }
Generated on Thu Jul 14 2022 06:24:36 by
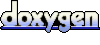