
pwm period is now 200us instead of the default 20ms veml6040 config is now AF_BIT | TRIG_BIT
Dependencies: mbed MMA8451Q USBDevice WakeUp vt100
Fork of afero_node_suntory_2017_06_15 by
AsymFIFO.hpp
00001 #ifndef _ASYMMETRICAL_FIFO_HPP_ 00002 #define _ASYMMETRICAL_FIFO_HPP_ 00003 00004 #include "mbed.h" 00005 #include "Preferences.hpp" 00006 #include "TimeEventHandler.hpp" 00007 #include "Accelerometer.hpp" 00008 #include "CheckSum.h" 00009 #include "base64.h" 00010 00011 #include <queue> 00012 00013 using namespace std; 00014 00015 namespace MaruSolSensorManager 00016 { 00017 class AsymFIFO 00018 { 00019 struct RECORD; 00020 queue<RECORD*> q_record; 00021 queue<char*> q_records; 00022 CheckSum *checkSum; 00023 struct RECORD 00024 { 00025 RECORD(time_t time, int16_t x, int16_t y, int16_t z) 00026 { 00027 this->data.time = time; 00028 this->data.x = x; 00029 this->data.y = y; 00030 this->data.z = z; 00031 } 00032 union 00033 { 00034 struct 00035 { 00036 int16_t x,y,z, dummy; 00037 time_t time; 00038 } data; 00039 uint8_t bytes[12]; 00040 }; 00041 }; 00042 uint32_t NUM_MAX_RECORD_Q; 00043 public: 00044 AsymFIFO() 00045 { 00046 checkSum = new CheckSum(); 00047 NUM_MAX_RECORD_Q = 6; 00048 } 00049 uint32_t buffed() 00050 { 00051 return q_records.size(); 00052 } 00053 void push(time_t time, short x, short y, short z) 00054 { 00055 RECORD *record = new RECORD(time,x,y,z); 00056 00057 q_record.push(record); 00058 00059 if(q_record.size()>=NUM_MAX_RECORD_Q) 00060 { 00061 char *pRecords = new char[sizeof(RECORD)*NUM_MAX_RECORD_Q]; 00062 memset(pRecords,'\0',sizeof(RECORD)*NUM_MAX_RECORD_Q); 00063 SERIAL_PRINT_DBG_FUNCNAME(); 00064 char *pRecords_crnt = pRecords; 00065 while(q_record.size()>0) 00066 { 00067 RECORD *rec = q_record.front(); 00068 memcpy(pRecords_crnt, rec->bytes, sizeof(rec->bytes)); 00069 delete rec; 00070 rec = NULL; 00071 q_record.pop(); 00072 pRecords_crnt += sizeof(RECORD); 00073 } 00074 q_records.push(pRecords); 00075 SERIAL_PRINT_DBG("q_records.size=%d\n",q_records.size()); 00076 if(q_records.size()>=100) 00077 { 00078 SERIAL_PRINT_DBG("Exceeded records number +100. Drop the last record\n"); 00079 char *lastRecords = q_records.front(); 00080 q_records.pop(); 00081 delete[] lastRecords; 00082 lastRecords = NULL; 00083 } 00084 } 00085 } 00086 void pop(uint8_t *buf, PREFERENCES::_crc32 *crc32) 00087 { 00088 char *p = NULL; 00089 p = q_records.front(); 00090 checkSum->reset(); 00091 checkSum->calc((uint8_t*)p,sizeof(RECORD)*NUM_MAX_RECORD_Q); 00092 PREFERENCES::_crc32 fCRC32 = checkSum->get(); 00093 SERIAL_PRINT_DBG("crc32 old:%08X new:%08X\n",PREFERENCES::CRC32.ui32, fCRC32.ui32); 00094 if(PREFERENCES::CRC32.ui32 == fCRC32.ui32) 00095 { 00096 q_records.pop(); 00097 delete[] p; 00098 p = q_records.front(); 00099 checkSum->calc((uint8_t*)p,sizeof(RECORD)*NUM_MAX_RECORD_Q); 00100 fCRC32 = checkSum->get(); 00101 } 00102 crc32->ui32 = 00103 fCRC32.ui32; 00104 uint8_t* workSpace = new uint8_t[255]; 00105 memset(workSpace,0,255); 00106 memcpy(workSpace, p, sizeof(RECORD)*NUM_MAX_RECORD_Q); 00107 memcpy(workSpace + sizeof(RECORD)*NUM_MAX_RECORD_Q, fCRC32.ui8, sizeof(fCRC32)); 00108 // for(uint32_t i = 0; i < 255; ++i) 00109 // { 00110 //SERIAL_PRINT_DBG("%02X-",workSpace[i]); 00111 // } 00112 size_t olen; 00113 size_t src_size = sizeof(RECORD)*NUM_MAX_RECORD_Q+sizeof(fCRC32); 00114 //SERIAL_PRINT_DBG("src_size=%d\n",src_size); 00115 mbedtls_base64_encode(buf, 255, &olen, workSpace,src_size); 00116 delete[] workSpace; 00117 } 00118 }; 00119 }; 00120 00121 #endif //_MARUSOL_SENSOR_MANAGER_TEMP_H_
Generated on Thu Jul 14 2022 06:24:36 by
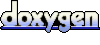