
pwm period is now 200us instead of the default 20ms veml6040 config is now AF_BIT | TRIG_BIT
Dependencies: mbed MMA8451Q USBDevice WakeUp vt100
Fork of afero_node_suntory_2017_06_15 by
AferoCommHndlr.cpp
00001 #include "AferoCommHndlr.h" 00002 00003 static AferoCommHndlr *self = NULL; 00004 00005 AferoCommHndlr::AferoCommHndlr() : flowControlEnabled(false) 00006 { 00007 deathWish = new Timeout(); 00008 setAttrCmpDelay = new Timeout(); 00009 _onGetTodoP = &AferoCommHndlr::_onGetTodo; 00010 } 00011 00012 void 00013 AferoCommHndlr::loop() 00014 { 00015 piafLib->loop(); 00016 todoQ->loop(); 00017 } 00018 00019 AferoCommHndlr* 00020 AferoCommHndlr::create(mbedSPI *spi) 00021 { 00022 SERIAL_PRINT_DBG("HELLO\n"); 00023 self = new AferoCommHndlr(); 00024 self->piafLib = iafLib::create((PinName)PINS::ASR_1::SPI::SR, 00025 AferoCommHndlr::fco_irq_fall, 00026 AferoCommHndlr::myOnAttributeSet, 00027 AferoCommHndlr::myOnAttributeSetComplete, spi); 00028 self->todoQ = ToDoQ::create(AferoCommHndlr::onGetTodo); 00029 SERIAL_PRINT_DBG("AferoCommHndlr init done\n"); 00030 self->piafLib->setAttribute8(1,1) != afSUCCESS ? SERIAL_PRINT_DBG("set attr failed!!\n") : NULL ; 00031 00032 return self; 00033 } 00034 00035 void 00036 AferoCommHndlr::myOnAttributeSet( 00037 const uint8_t requestId, 00038 const uint16_t attributeId, 00039 const uint16_t valueLen, 00040 const uint8_t *value) 00041 { 00042 self->piafLib->setAttributeComplete(requestId, attributeId, valueLen, value) != afSUCCESS ? SERIAL_PRINT_DBG("set attr failed!!\n") : NULL ; 00043 } 00044 00045 int32_t 00046 AferoCommHndlr::sanitizeSensingInterval(uint16_t attributeId, int32_t interval) 00047 { 00048 if(interval < 10) 00049 { 00050 interval = 10; 00051 self->piafLib->setAttribute32(attributeId, interval) != afSUCCESS ? SERIAL_PRINT_DBG("set attr failed!!\n") : NULL ; 00052 } 00053 return interval; 00054 } 00055 00056 void 00057 AferoCommHndlr::myOnAttributeSetComplete( 00058 const uint8_t requestId, 00059 const uint16_t attributeId, 00060 const uint16_t valueLen, 00061 const uint8_t *value) 00062 { 00063 self->_myOnAttributeSetComplete(requestId,attributeId,valueLen,value); 00064 } 00065 00066 void 00067 AferoCommHndlr::_myOnAttributeSetComplete( 00068 const uint8_t requestId, 00069 const uint16_t attributeId, 00070 const uint16_t valueLen, 00071 const uint8_t *value) 00072 { 00073 int32_t sensing_interval; 00074 char *buf = NULL; 00075 if(attributeId >= 4 && attributeId <= 8) 00076 { 00077 sensing_interval = *((int32_t*)value); 00078 } 00079 00080 if(attributeId==2) 00081 { 00082 PREFERENCES::CRC32.ui32 = *((uint32_t*)value); 00083 } 00084 else if(attributeId==4) 00085 { 00086 buf = "PREFERENCES::SENSING_INTERVAL[SENSORS::ACCELEROMETER]"; 00087 PREFERENCES::SENSING_INTERVAL[SENSORS::ACCELEROMETER] = sanitizeSensingInterval(attributeId,sensing_interval); 00088 } 00089 else if(attributeId==5) 00090 { 00091 buf = "PREFERENCES::SENSING_INTERVAL[SENSORS::COLOR]"; 00092 PREFERENCES::SENSING_INTERVAL[SENSORS::COLOR] = sanitizeSensingInterval(attributeId,sensing_interval); 00093 } 00094 else if(attributeId==6) 00095 { 00096 buf = "PREFERENCES::SENSING_INTERVAL[SENSORS::PRESSURE]"; 00097 PREFERENCES::SENSING_INTERVAL[SENSORS::PRESSURE] = sanitizeSensingInterval(attributeId,sensing_interval); 00098 } 00099 else if(attributeId==7) 00100 { 00101 buf = "PREFERENCES::SENSING_INTERVAL[SENSORS::CURRENT_TRANS]"; 00102 PREFERENCES::SENSING_INTERVAL[SENSORS::CURRENT_TRANS] = sanitizeSensingInterval(attributeId,sensing_interval); 00103 } 00104 else if(attributeId==8) 00105 { 00106 buf = "PREFERENCES::SENSING_INTERVAL[SENSORS::TEMPERATURE]"; 00107 PREFERENCES::SENSING_INTERVAL[SENSORS::TEMPERATURE] = sanitizeSensingInterval(attributeId,sensing_interval); 00108 } 00109 else if(attributeId==9) 00110 { 00111 SERIAL_PRINT_DBG("time stamp:%ld\n", *((uint32_t*)value)); 00112 time_t t; 00113 RTC_GET_UTC(t); 00114 if(*((uint32_t*)value) > ((uint32_t)t)) 00115 { 00116 RTC_Handler::getInstance()->setUTC(*((uint32_t*)value)); 00117 } 00118 } 00119 else if(attributeId==1024) 00120 { 00121 } 00122 00123 if(attributeId >= 4 && attributeId <= 8) 00124 { 00125 SERIAL_PRINT_DBG("%s=%ld\n",buf,sensing_interval); 00126 } 00127 //_onGetTodoP = &AferoCommHndlr::_onGetTodo; 00128 setAttrCmpDelay->attach(callback(this, &AferoCommHndlr::onSetAttrCmpDelay), 5); 00129 } 00130 00131 void 00132 AferoCommHndlr::fco_irq_fall() 00133 { 00134 if(self->piafLib) 00135 { 00136 self->piafLib->mcuISR(); 00137 } 00138 } 00139 00140 bool 00141 AferoCommHndlr::_onGetTodo(ToDo *todo) 00142 { 00143 _onGetTodoP = &AferoCommHndlr::_onGetTodoFalsy; 00144 char buf[255]; 00145 memset(buf,'\0',sizeof(buf)); 00146 if(todo!=NULL) 00147 { 00148 if(PREFERENCES::FLOW_CONTROL==true) 00149 { 00150 PREFERENCES::_crc32 crc32; 00151 todo->toBASE64(buf,&crc32); 00152 if(piafLib->setAttribute(ATTR_ID_SENSE_VAL,strlen(buf),buf) != afSUCCESS) 00153 { 00154 SERIAL_PRINT_DBG("set attr failed!!\n"); 00155 return false; 00156 } 00157 if(piafLib->setAttribute64(3,crc32.ui32) != afSUCCESS) 00158 { 00159 SERIAL_PRINT_DBG("set attr failed!!\n"); 00160 return false; 00161 } 00162 } 00163 else 00164 { 00165 todo->toJSON(buf); 00166 int ret = afSUCCESS; 00167 if((ret=piafLib->setAttribute(ATTR_ID_SENSE_VAL,strlen(buf),buf)) != afSUCCESS) 00168 { 00169 SERIAL_PRINT_DBG("set attr failed!! : %d\n", ret); 00170 todo->error_count_inc(); 00171 return false; 00172 } 00173 else 00174 { 00175 SERIAL_PRINT_DBG("set attr success!! : %d\n", ret); 00176 SERIAL_PRINT_DBG("%s\n",buf); 00177 todo->error_count_clear(); 00178 return true; 00179 } 00180 } 00181 } 00182 return true; 00183 } 00184 00185 bool 00186 AferoCommHndlr::_onGetTodoFalsy(ToDo *todo) 00187 { 00188 return false; 00189 } 00190 00191 bool 00192 AferoCommHndlr::onGetTodo(ToDo *todo) 00193 { 00194 return (self->*(self->_onGetTodoP))(todo); 00195 } 00196 00197 void 00198 AferoCommHndlr::onSetAttrCmpDelay() 00199 { 00200 SERIAL_PRINT_DBG("onSetAttrCmpDelay triggered\n"); 00201 _onGetTodoP = &AferoCommHndlr::_onGetTodo; 00202 }
Generated on Thu Jul 14 2022 06:24:36 by
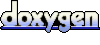