
v1
Dependencies: MQTTSN mbed-http
MQTTSN_func.h
00001 int connack_rc = 0; 00002 int retryAttempt = 0; 00003 int connectTimeout = 1000; 00004 int rc = 0; 00005 00006 enum connack_return_codes 00007 { 00008 MQTTSN_CONNECTION_ACCEPTED = 0, 00009 MQTTSN_REJECTED_CONGESTION = 1, 00010 MQTTSN_REJECTED_INVALID_TOPIC_ID = 2, 00011 MQTTSN_REJECTED_NOT_SUPPORTED= 3, 00012 }; 00013 00014 static volatile bool isMessageArrived = false; 00015 int arrivedcount = 0; 00016 00017 int connect(MQTTSN::Client<MQTTSNUDP, Countdown> *client, MQTTSNUDP* ipstack) 00018 { 00019 int rc; 00020 MQTTSNPacket_connectData data = MQTTSNPacket_connectData_initializer; 00021 data.clientID.cstring = MQTT_CLIENT_ID; 00022 data.duration = 60; 00023 if ((rc = client->connect(data)) == 0) { 00024 printf ("--->MQTT-SN Connected\n\r"); 00025 } 00026 else { 00027 printf("MQTT-SN connect returned %d\n", rc); 00028 } 00029 if (rc >= 0) 00030 connack_rc = rc; 00031 return rc; 00032 } 00033 00034 int getConnTimeout(int attemptNumber) 00035 { // First 10 attempts try within 3 seconds, next 10 attempts retry after every 1 minute 00036 // after 20 attempts, retry every 10 minutes 00037 return (attemptNumber < 10) ? 3 : (attemptNumber < 20) ? 60 : 600; 00038 } 00039 00040 void attemptConnect(MQTTSN::Client<MQTTSNUDP, Countdown> *client, MQTTSNUDP* ipstack) 00041 { 00042 while (connect(client, ipstack) != MQTTSN_CONNECTION_ACCEPTED) 00043 { 00044 int timeout = getConnTimeout(++retryAttempt); 00045 printf("Retry attempt number %d waiting %d\n", retryAttempt, timeout); 00046 00047 // if ipstack and client were on the heap we could deconstruct and goto a label where they are constructed 00048 // or maybe just add the proper members to do this disconnect and call attemptConnect(...) 00049 // this works - reset the system when the retry count gets to a threshold 00050 if (retryAttempt == 5) 00051 NVIC_SystemReset(); 00052 else 00053 wait(timeout); 00054 } 00055 } 00056 00057 void messageArrived(MQTTSN::MessageData& md) 00058 { 00059 MQTTSN::Message &message = md.message; 00060 printf("Message arrived: qos %d, retained %d, dup %d, packetid %d\n, Number: %d", message.qos, message.retained, message.dup, message.id, arrivedcount); 00061 memcpy(messageBuffer, (char*)message.payload, message.payloadlen); 00062 messageBuffer[message.payloadlen] = '\0'; 00063 printf("Payload %.*s\n", message.payloadlen, (char*)message.payload); 00064 printf("Payload length %d\n",message.payloadlen); 00065 ++arrivedcount; 00066 process_msg(); 00067 isMessageArrived = true; 00068 } 00069 00070 int subscribe(MQTTSN::Client<MQTTSNUDP, Countdown> *client, MQTTSNUDP* ipstack, MQTTSN_topicid& topicid) 00071 { 00072 //MQTTSN_topicid topicid; 00073 topicid.type = MQTTSN_TOPIC_TYPE_NORMAL; 00074 topicid.data.long_.name = MQTT_TOPIC; 00075 topicid.data.long_.len = strlen(MQTT_TOPIC); 00076 MQTTSN::QoS grantedQoS; 00077 return client->subscribe(topicid, MQTTSN::QOS1, grantedQoS, messageArrived); 00078 } 00079 00080 int publish(MQTTSN::Client<MQTTSNUDP, Countdown> *client, MQTTSNUDP* ipstack, MQTTSN_topicid& topicid2) 00081 { 00082 int rc; 00083 if(PUB_REG == false) 00084 { 00085 if ((rc = subscribe(client, ipstack, topicid2)) != 0) 00086 printf("rc from MQTT subscribe is %d\n", rc); 00087 else{ 00088 printf("Subscribed to Topic %s\n", MQTT_TOPIC); 00089 PUB_REG = true; 00090 } 00091 } 00092 00093 MQTTSN::Message message; 00094 message.qos = MQTTSN::QOS1; 00095 message.retained = false; 00096 message.dup = false; 00097 message.payload = (void*)buf; 00098 message.payloadlen = strlen(buf)+1; 00099 return client->publish(topicid2, message); 00100 }
Generated on Thu Jul 14 2022 08:11:13 by
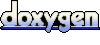