
NuMaker emWin HMI
Embed:
(wiki syntax)
Show/hide line numbers
tslib.h
00001 #ifndef _TSLIB_H_ 00002 #define _TSLIB_H_ 00003 /* 00004 * tslib/src/tslib.h 00005 * 00006 * Copyright (C) 2001 Russell King. 00007 * 00008 * This file is placed under the LGPL. 00009 * 00010 * $Id: tslib.h,v 1.4 2005/02/26 01:47:23 kergoth Exp $ 00011 * 00012 * Touch screen library interface definitions. 00013 */ 00014 #ifdef __cplusplus 00015 extern "C" { 00016 #endif /* __cplusplus */ 00017 #if 0 00018 #include <stdarg.h> 00019 //#include <sys/time.h> 00020 00021 #ifdef WIN32 00022 #define TSIMPORT __declspec(dllimport) 00023 #define TSEXPORT __declspec(dllexport) 00024 #define TSLOCAL 00025 #else 00026 #define TSIMPORT 00027 #ifdef GCC_HASCLASSVISIBILITY 00028 #define TSEXPORT __attribute__ ((visibility("default"))) 00029 #define TSLOCAL __attribute__ ((visibility("hidden"))) 00030 #else 00031 #define TSEXPORT 00032 #define TSLOCAL 00033 #endif 00034 #endif 00035 00036 #ifdef TSLIB_INTERNAL 00037 #define TSAPI TSEXPORT 00038 #else 00039 #define TSAPI TSIMPORT 00040 #endif // TSLIB_INTERNAL 00041 00042 struct tsdev; 00043 00044 struct ts_sample 00045 { 00046 int x; 00047 int y; 00048 unsigned int pressure; 00049 // struct timeval tv; 00050 }; 00051 00052 /* 00053 * Close the touchscreen device, free all resources. 00054 */ 00055 TSAPI int ts_close(struct tsdev *); 00056 00057 /* 00058 * Configure the touchscreen device. 00059 */ 00060 TSAPI int ts_config(struct tsdev *); 00061 00062 /* 00063 * Change this hook to point to your custom error handling function. 00064 */ 00065 extern TSAPI int (*ts_error_fn)(const char *fmt, va_list ap); 00066 00067 /* 00068 * Returns the file descriptor in use for the touchscreen device. 00069 */ 00070 TSAPI int ts_fd(struct tsdev *); 00071 00072 /* 00073 * Load a filter/scaling module 00074 */ 00075 TSAPI int ts_load_module(struct tsdev *, const char *mod, const char *params); 00076 00077 /* 00078 * Open the touchscreen device. 00079 */ 00080 TSAPI struct tsdev *ts_open(const char *dev_name, int nonblock); 00081 00082 /* 00083 * Return a scaled touchscreen sample. 00084 */ 00085 TSAPI int ts_read(struct tsdev *, struct ts_sample *, int); 00086 00087 /* 00088 * Returns a raw, unscaled sample from the touchscreen. 00089 */ 00090 TSAPI int ts_read_raw(struct tsdev *, struct ts_sample *, int); 00091 00092 int ts_calibrate(int xsize, int ysize); 00093 00094 int ts_phy2log(int *sumx, int *sumy); 00095 #endif 00096 #ifdef __cplusplus 00097 } 00098 #endif /* __cplusplus */ 00099 #endif /* _TSLIB_H_ */
Generated on Mon Mar 4 2024 07:48:13 by
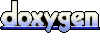