
NuMaker emWin HMI
Embed:
(wiki syntax)
Show/hide line numbers
SPINBOX.h
00001 /********************************************************************* 00002 * SEGGER Software GmbH * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2018 SEGGER Microcontroller GmbH * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.48 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed by SEGGER Software GmbH to Nuvoton Technology Corporationat the address: No. 4, Creation Rd. III, Hsinchu Science Park, Taiwan 00019 for the purposes of creating libraries for its 00020 Arm Cortex-M and Arm9 32-bit microcontrollers, commercialized and distributed by Nuvoton Technology Corporation 00021 under the terms and conditions of an End User 00022 License Agreement supplied with the libraries. 00023 Full source code is available at: www.segger.com 00024 00025 We appreciate your understanding and fairness. 00026 ---------------------------------------------------------------------- 00027 Licensing information 00028 Licensor: SEGGER Software GmbH 00029 Licensed to: Nuvoton Technology Corporation, No. 4, Creation Rd. III, Hsinchu Science Park, 30077 Hsinchu City, Taiwan 00030 Licensed SEGGER software: emWin 00031 License number: GUI-00735 00032 License model: emWin License Agreement, signed February 27, 2018 00033 Licensed platform: Cortex-M and ARM9 32-bit series microcontroller designed and manufactured by or for Nuvoton Technology Corporation 00034 ---------------------------------------------------------------------- 00035 Support and Update Agreement (SUA) 00036 SUA period: 2018-03-26 - 2019-03-27 00037 Contact to extend SUA: sales@segger.com 00038 ---------------------------------------------------------------------- 00039 File : SPINBOX.h 00040 Purpose : SPINBOX header file 00041 --------------------END-OF-HEADER------------------------------------- 00042 */ 00043 00044 #ifndef SPINBOX_H 00045 #define SPINBOX_H 00046 00047 #include "WM.h" 00048 #include "DIALOG_Intern.h" // Required for Create indirect data structure 00049 #include "WIDGET.h" 00050 #include "GUI_Debug.h" 00051 #include "EDIT.h" 00052 00053 #if GUI_WINSUPPORT 00054 00055 #if defined(__cplusplus) 00056 extern "C" { // Make sure we have C-declarations in C++ programs 00057 #endif 00058 00059 /********************************************************************* 00060 * 00061 * Defines 00062 * 00063 ********************************************************************** 00064 */ 00065 /********************************************************************* 00066 * 00067 * States 00068 */ 00069 #define SPINBOX_STATE_PRESSED(x) (U8)(1 << (U8)x) // These flags are stored in (SPINBOX_OBJ->State) | x must be 0 or 1 00070 #define SPINBOX_STATE_FOCUS WIDGET_STATE_FOCUS // This is read from (SPINBOX_OBJ->Widget.State) 00071 00072 #define SPINBOX_EDGE_RIGHT 0 00073 #define SPINBOX_EDGE_LEFT 1 00074 #define SPINBOX_EDGE_CENTER 2 00075 00076 #define SPINBOX_EM_STEP 0 00077 #define SPINBOX_EM_EDIT 1 00078 00079 #ifndef SPINBOX_EM_DEFAULT 00080 #define SPINBOX_EM_DEFAULT SPINBOX_EM_STEP 00081 #endif 00082 00083 /********************************************************************* 00084 * 00085 * Color indices 00086 */ 00087 #define SPINBOX_CI_DISABLED EDIT_CI_DISABLED 00088 #define SPINBOX_CI_ENABLED EDIT_CI_ENABLED 00089 #define SPINBOX_CI_PRESSED 2 00090 00091 /********************************************************************* 00092 * 00093 * Skinning property indices 00094 */ 00095 #define SPINBOX_SKIN_FLEX SPINBOX_DrawSkinFlex 00096 00097 #define SPINBOX_SKINFLEX_PI_PRESSED 0 00098 #define SPINBOX_SKINFLEX_PI_FOCUSED 1 00099 #define SPINBOX_SKINFLEX_PI_ENABLED 2 00100 #define SPINBOX_SKINFLEX_PI_DISABLED 3 00101 #define SPINBOX_SKIN_FLEX_RADIUS 2 00102 00103 /********************************************************************* 00104 * 00105 * Public Types 00106 * 00107 ********************************************************************** 00108 */ 00109 typedef WM_HMEM SPINBOX_Handle; 00110 00111 typedef struct { 00112 GUI_COLOR aColorFrame[2]; // [0] Outer color of surrounding frame. [1] Inner color of surrounding frame. 00113 GUI_COLOR aColorUpper[2]; // [0] Upper color of gradient for upper button. [1] Lower color of gradient for upper button. 00114 GUI_COLOR aColorLower[2]; // [0] Upper color of gradient for lower button. [1] Lower color of gradient for lower button. 00115 GUI_COLOR ColorArrow; // Color of the button arrow. 00116 GUI_COLOR ColorBk; // Color of the background. // See WIDGET_ITEM_CREATE in SPINBOX_DrawSkinFlex() 00117 GUI_COLOR ColorText; // Color of the text. // See WIDGET_ITEM_CREATE in SPINBOX_DrawSkinFlex() 00118 GUI_COLOR ColorButtonFrame; // Color of the button frame. 00119 } SPINBOX_SKINFLEX_PROPS; 00120 00121 /********************************************************************* 00122 * 00123 * Prototypes 00124 * 00125 ********************************************************************** 00126 */ 00127 /********************************************************************* 00128 * 00129 * Creation 00130 */ 00131 SPINBOX_Handle SPINBOX_CreateEx (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int WinFlags, int Id, int Min, int Max); 00132 SPINBOX_Handle SPINBOX_CreateUser (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int WinFlags, int Id, int Min, int Max, int NumExtraBytes); 00133 SPINBOX_Handle SPINBOX_CreateIndirect(const GUI_WIDGET_CREATE_INFO * pCreateInfo, WM_HWIN hWinParent, int x0, int y0, WM_CALLBACK * cb); 00134 00135 /********************************************************************* 00136 * 00137 * Callback, should be called only from within a custom callback. 00138 */ 00139 void SPINBOX_Callback(WM_MESSAGE * pMsg); 00140 00141 /********************************************************************* 00142 * 00143 * Get / Set properties 00144 */ 00145 void SPINBOX_EnableBlink (SPINBOX_Handle hObj, int Period, int OnOff); 00146 GUI_COLOR SPINBOX_GetBkColor (SPINBOX_Handle hObj, unsigned int Index); 00147 GUI_COLOR SPINBOX_GetButtonBkColor(SPINBOX_Handle hObj, unsigned int Index); 00148 EDIT_Handle SPINBOX_GetEditHandle (SPINBOX_Handle hObj); 00149 const GUI_FONT * SPINBOX_GetFont (SPINBOX_Handle hObj); 00150 GUI_COLOR SPINBOX_GetTextColor (SPINBOX_Handle hObj, unsigned int Index); 00151 int SPINBOX_GetUserData (SPINBOX_Handle hObj, void * pDest, int NumBytes); 00152 I32 SPINBOX_GetValue (SPINBOX_Handle hObj); 00153 void SPINBOX_SetBkColor (SPINBOX_Handle hObj, unsigned int Index, GUI_COLOR Color); 00154 void SPINBOX_SetButtonBkColor(SPINBOX_Handle hObj, unsigned int Index, GUI_COLOR Color); 00155 void SPINBOX_SetButtonSize (SPINBOX_Handle hObj, unsigned ButtonSize); 00156 void SPINBOX_SetEdge (SPINBOX_Handle hObj, U8 Edge); 00157 void SPINBOX_SetEditMode (SPINBOX_Handle hObj, U8 EditMode); 00158 void SPINBOX_SetFont (SPINBOX_Handle hObj, const GUI_FONT * pFont); 00159 void SPINBOX_SetRange (SPINBOX_Handle hObj, I32 Min, I32 Max); 00160 U16 SPINBOX_SetStep (SPINBOX_Handle hObj, U16 Step); 00161 void SPINBOX_SetTextColor (SPINBOX_Handle hObj, unsigned int Index, GUI_COLOR Color); 00162 int SPINBOX_SetUserData (SPINBOX_Handle hObj, const void * pSrc, int NumBytes); 00163 void SPINBOX_SetValue (SPINBOX_Handle hObj, I32 Value); 00164 00165 /********************************************************************* 00166 * 00167 * Managing default values 00168 */ 00169 U16 SPINBOX_GetDefaultButtonSize(void); 00170 void SPINBOX_SetDefaultButtonSize(U16 ButtonSize); 00171 00172 /********************************************************************* 00173 * 00174 * Skinning 00175 */ 00176 void SPINBOX_GetSkinFlexProps (SPINBOX_SKINFLEX_PROPS * pProps, int Index); 00177 void SPINBOX_SetSkinClassic (SPINBOX_Handle hObj); 00178 void SPINBOX_SetSkin (SPINBOX_Handle hObj, WIDGET_DRAW_ITEM_FUNC * pfDrawSkin); 00179 int SPINBOX_DrawSkinFlex (const WIDGET_ITEM_DRAW_INFO * pDrawItemInfo); 00180 void SPINBOX_SetSkinFlexProps (const SPINBOX_SKINFLEX_PROPS * pProps, int Index); 00181 void SPINBOX_SetDefaultSkinClassic(void); 00182 WIDGET_DRAW_ITEM_FUNC * SPINBOX_SetDefaultSkin(WIDGET_DRAW_ITEM_FUNC * pfDrawSkin); 00183 00184 #if defined(__cplusplus) 00185 } 00186 #endif 00187 00188 #endif // GUI_WINSUPPORT 00189 #endif // SPINBOX_H 00190 00191 /*************************** End of file ****************************/
Generated on Mon Mar 4 2024 07:48:13 by
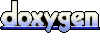