
NuMaker emWin HMI
Embed:
(wiki syntax)
Show/hide line numbers
M48XTouchPanel.c
00001 #if 0 // moved to the related target folders 00002 #include "M480.h" 00003 00004 //#include "stdlib.h" 00005 #include "GUI.h" 00006 //#include "W55FA93_adc.h" 00007 #include "M48XTouchPanel.h" 00008 //#include "lcdconf.h" 00009 00010 00011 00012 int Init_TouchPanel(void) 00013 { 00014 /* Enable peripheral clock */ 00015 CLK_EnableModuleClock(EADC_MODULE); 00016 00017 /* Peripheral clock source */ 00018 CLK_SetModuleClock(EADC_MODULE, 0, CLK_CLKDIV0_EADC(8)); 00019 00020 /* Init ADC for TP */ 00021 /* Set input mode as single-end and enable the A/D converter */ 00022 EADC_Open(EADC, EADC_CTL_DIFFEN_SINGLE_END); 00023 00024 return 1; 00025 } 00026 00027 static volatile uint32_t g_u32AdcIntFlag_TP; 00028 00029 /*-----------------------------------------------*/ 00030 // ADC01 ISR 00031 // 00032 /*-----------------------------------------------*/ 00033 void ADC01_IRQHandler(void) 00034 { 00035 /* Clear the A/D ADINT1 interrupt flag */ 00036 EADC_CLR_INT_FLAG(EADC, EADC_STATUS2_ADIF1_Msk); 00037 00038 g_u32AdcIntFlag_TP = 1; 00039 00040 } 00041 00042 /*-----------------------------------------------*/ 00043 // Get X Position from Touch Panel (ADC input) 00044 // 00045 /*-----------------------------------------------*/ 00046 uint16_t Get_TP_X(void) 00047 { 00048 uint16_t x_adc_in; 00049 00050 /*=== Get X from ADC input ===*/ 00051 GPIO_SetMode(PB, BIT9, GPIO_MODE_OUTPUT); // XR 00052 GPIO_SetMode(PH, BIT5, GPIO_MODE_INPUT); // YD 00053 GPIO_SetMode(PH, BIT4, GPIO_MODE_OUTPUT); // XL 00054 PB9 = 1; 00055 PH4 = 0; 00056 00057 /* Configure the GPB8 ADC analog input pins. */ 00058 SYS->GPB_MFPH &= ~(SYS_GPB_MFPH_PB8MFP_Msk | SYS_GPB_MFPH_PB9MFP_Msk); 00059 SYS->GPB_MFPH |= SYS_GPB_MFPH_PB8MFP_EADC0_CH8; 00060 00061 /* Disable the GPB8 digital input path to avoid the leakage current. */ 00062 GPIO_DISABLE_DIGITAL_PATH(PB, BIT8); 00063 00064 /* Configure the sample module 1 for analog input channel 8 and software trigger source.*/ 00065 EADC_ConfigSampleModule(EADC, 1, EADC_SOFTWARE_TRIGGER, 8); // YU 00066 00067 /* Clear the A/D ADINT1 interrupt flag for safe */ 00068 EADC_CLR_INT_FLAG(EADC, EADC_STATUS2_ADIF1_Msk); 00069 00070 /* Enable the sample module 1 interrupt. */ 00071 EADC_ENABLE_INT(EADC, BIT1); //Enable sample module A/D ADINT1 interrupt. 00072 EADC_ENABLE_SAMPLE_MODULE_INT(EADC, 1, BIT1); //Enable sample module 1 interrupt. 00073 NVIC_EnableIRQ(43); //for ADC1_IRQn and EADC01_IRQn 00074 00075 /* Reset the ADC interrupt indicator and trigger sample module 1 to start A/D conversion */ 00076 g_u32AdcIntFlag_TP = 0; 00077 EADC_START_CONV(EADC, BIT1); 00078 00079 /* Wait ADC interrupt (g_u32AdcIntFlag_TP will be set at IRQ_Handler function) */ 00080 //while(g_u32AdcIntFlag_TP == 0); 00081 EADC_CLR_INT_FLAG(EADC, EADC_STATUS2_ADIF1_Msk); 00082 g_u32AdcIntFlag_TP = 1; 00083 x_adc_in = EADC_GET_CONV_DATA(EADC, 1); 00084 return x_adc_in; 00085 00086 } 00087 00088 00089 /*-----------------------------------------------*/ 00090 // Get Y Position from Touch Panel (ADC input) 00091 // 00092 /*-----------------------------------------------*/ 00093 uint16_t Get_TP_Y(void) 00094 { 00095 uint16_t y_adc_in; 00096 00097 /*=== Get Y from ADC input ===*/ 00098 GPIO_SetMode(PB, BIT8, GPIO_MODE_OUTPUT); // YU 00099 GPIO_SetMode(PH, BIT5, GPIO_MODE_OUTPUT); // YD 00100 GPIO_SetMode(PH, BIT4, GPIO_MODE_INPUT); // XL 00101 PB8 = 1; 00102 PH5 = 0; 00103 00104 /* Configure the GPB9 ADC analog input pins. */ 00105 SYS->GPB_MFPH &= ~(SYS_GPB_MFPH_PB8MFP_Msk | SYS_GPB_MFPH_PB9MFP_Msk); 00106 SYS->GPB_MFPH |= SYS_GPB_MFPH_PB9MFP_EADC0_CH9; 00107 00108 /* Disable the GPB9 digital input path to avoid the leakage current. */ 00109 GPIO_DISABLE_DIGITAL_PATH(PB, BIT9); 00110 00111 /* Configure the sample module 2 for analog input channel 9 and software trigger source.*/ 00112 EADC_ConfigSampleModule(EADC, 2, EADC_SOFTWARE_TRIGGER, 9); // XR 00113 00114 /* Clear the A/D ADINT1 interrupt flag for safe */ 00115 EADC_CLR_INT_FLAG(EADC, EADC_STATUS2_ADIF1_Msk); 00116 00117 /* Enable the sample module 2 interrupt. */ 00118 EADC_ENABLE_INT(EADC, BIT2); //Enable sample module A/D ADINT1 interrupt. 00119 EADC_ENABLE_SAMPLE_MODULE_INT(EADC, 1, BIT2); //Enable sample module 2 interrupt. 00120 NVIC_EnableIRQ(43); //for ADC1_IRQn and EADC01_IRQn) 00121 00122 /* Reset the ADC interrupt indicator and trigger sample module 2 to start A/D conversion */ 00123 g_u32AdcIntFlag_TP = 0; 00124 EADC_START_CONV(EADC, BIT2); 00125 00126 /* Wait ADC interrupt (g_u32AdcIntFlag_TP will be set at IRQ_Handler function) */ 00127 //while(g_u32AdcIntFlag_TP == 0); 00128 EADC_CLR_INT_FLAG(EADC, EADC_STATUS2_ADIF1_Msk); 00129 g_u32AdcIntFlag_TP = 1; 00130 y_adc_in = EADC_GET_CONV_DATA(EADC, 2); 00131 return y_adc_in; 00132 00133 } 00134 00135 int Read_TouchPanel(int *x, int *y) 00136 { 00137 *x = Get_TP_X(); 00138 *y = Get_TP_Y(); 00139 if ( (*x == 0xFFF) || (*y == 0xFFF) ) 00140 return 0; 00141 else 00142 return 1; 00143 } 00144 00145 int Uninit_TouchPanel(void) 00146 { 00147 return 1; 00148 } 00149 00150 int Check_TouchPanel(void) 00151 { 00152 return 0; //Pen up; 00153 } 00154 #endif
Generated on Mon Mar 4 2024 07:48:13 by
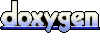