
NuMaker emWin HMI
Embed:
(wiki syntax)
Show/hide line numbers
KNOB_Private.h
00001 /********************************************************************* 00002 * SEGGER Software GmbH * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2018 SEGGER Microcontroller GmbH * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.48 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed by SEGGER Software GmbH to Nuvoton Technology Corporationat the address: No. 4, Creation Rd. III, Hsinchu Science Park, Taiwan 00019 for the purposes of creating libraries for its 00020 Arm Cortex-M and Arm9 32-bit microcontrollers, commercialized and distributed by Nuvoton Technology Corporation 00021 under the terms and conditions of an End User 00022 License Agreement supplied with the libraries. 00023 Full source code is available at: www.segger.com 00024 00025 We appreciate your understanding and fairness. 00026 ---------------------------------------------------------------------- 00027 Licensing information 00028 Licensor: SEGGER Software GmbH 00029 Licensed to: Nuvoton Technology Corporation, No. 4, Creation Rd. III, Hsinchu Science Park, 30077 Hsinchu City, Taiwan 00030 Licensed SEGGER software: emWin 00031 License number: GUI-00735 00032 License model: emWin License Agreement, signed February 27, 2018 00033 Licensed platform: Cortex-M and ARM9 32-bit series microcontroller designed and manufactured by or for Nuvoton Technology Corporation 00034 ---------------------------------------------------------------------- 00035 Support and Update Agreement (SUA) 00036 SUA period: 2018-03-26 - 2019-03-27 00037 Contact to extend SUA: sales@segger.com 00038 ---------------------------------------------------------------------- 00039 File : KNOB.h 00040 Purpose : KNOB include 00041 --------------------END-OF-HEADER------------------------------------- 00042 */ 00043 00044 #ifndef KNOB_PRIVATE_H 00045 #define KNOB_PRIVATE_H 00046 00047 #include "KNOB.h" 00048 #include "GUI_Private.h" 00049 00050 #if (GUI_SUPPORT_MEMDEV && GUI_WINSUPPORT) 00051 00052 /********************************************************************* 00053 * 00054 * Object definition 00055 * 00056 ********************************************************************** 00057 */ 00058 typedef struct { 00059 I32 Snap; // Position where the knob snaps 00060 I32 Period; // Time it takes to stop the knob in ms 00061 GUI_COLOR BkColor; // The Bk color 00062 I32 Offset; // the offset 00063 I32 MinRange; 00064 I32 MaxRange; 00065 I32 TickSize; // Minimum movement range in 1/10 of degree 00066 I32 KeyValue; // Range of movement for one key push 00067 } KNOB_PROPS; 00068 00069 typedef struct { 00070 WIDGET Widget; 00071 KNOB_PROPS Props; 00072 WM_HMEM hContext; 00073 I32 Angle; 00074 I32 Value; 00075 int xSize; 00076 int ySize; 00077 GUI_MEMDEV_Handle hMemSrc; 00078 GUI_MEMDEV_Handle hMemDst; 00079 GUI_MEMDEV_Handle hMemBk; 00080 } KNOB_OBJ; 00081 00082 /********************************************************************* 00083 * 00084 * Macros for internal use 00085 * 00086 ********************************************************************** 00087 */ 00088 #if GUI_DEBUG_LEVEL >= GUI_DEBUG_LEVEL_CHECK_ALL 00089 #define KNOB_INIT_ID(p) p->Widget.DebugId = KNOB_ID 00090 #else 00091 #define KNOB_INIT_ID(p) 00092 #endif 00093 00094 #if GUI_DEBUG_LEVEL >= GUI_DEBUG_LEVEL_CHECK_ALL 00095 KNOB_OBJ * KNOB_LockH(KNOB_Handle h); 00096 #define KNOB_LOCK_H(h) KNOB_LockH(h) 00097 #else 00098 #define KNOB_LOCK_H(h) (KNOB_OBJ *)GUI_LOCK_H(h) 00099 #endif 00100 00101 /********************************************************************* 00102 * 00103 * Module internal data 00104 * 00105 ********************************************************************** 00106 */ 00107 extern KNOB_PROPS KNOB__DefaultProps; 00108 00109 #endif // (GUI_SUPPORT_MEMDEV && GUI_WINSUPPORT) 00110 #endif // KNOB_PRIVATE_H
Generated on Mon Mar 4 2024 07:48:13 by
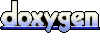