
NuMaker emWin HMI
Embed:
(wiki syntax)
Show/hide line numbers
GUI_X.c
00001 /********************************************************************* 00002 * SEGGER Software GmbH * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2018 SEGGER Microcontroller GmbH * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.48 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed by SEGGER Software GmbH to Nuvoton Technology Corporationat the address: No. 4, Creation Rd. III, Hsinchu Science Park, Taiwan 00019 for the purposes of creating libraries for its 00020 Arm Cortex-M and Arm9 32-bit microcontrollers, commercialized and distributed by Nuvoton Technology Corporation 00021 under the terms and conditions of an End User 00022 License Agreement supplied with the libraries. 00023 Full source code is available at: www.segger.com 00024 00025 We appreciate your understanding and fairness. 00026 ---------------------------------------------------------------------- 00027 Licensing information 00028 Licensor: SEGGER Software GmbH 00029 Licensed to: Nuvoton Technology Corporation, No. 4, Creation Rd. III, Hsinchu Science Park, 30077 Hsinchu City, Taiwan 00030 Licensed SEGGER software: emWin 00031 License number: GUI-00735 00032 License model: emWin License Agreement, signed February 27, 2018 00033 Licensed platform: Cortex-M and ARM9 32-bit series microcontroller designed and manufactured by or for Nuvoton Technology Corporation 00034 ---------------------------------------------------------------------- 00035 Support and Update Agreement (SUA) 00036 SUA period: 2018-03-26 - 2019-03-27 00037 Contact to extend SUA: sales@segger.com 00038 ---------------------------------------------------------------------- 00039 File : GUI_X.C 00040 Purpose : Config / System dependent externals for GUI 00041 ---------------------------END-OF-HEADER------------------------------ 00042 */ 00043 00044 #include "GUI.h" 00045 00046 /********************************************************************* 00047 * 00048 * Global data 00049 */ 00050 volatile GUI_TIMER_TIME OS_TimeMS; 00051 00052 /********************************************************************* 00053 * 00054 * Timing: 00055 * GUI_X_GetTime() 00056 * GUI_X_Delay(int) 00057 00058 Some timing dependent routines require a GetTime 00059 and delay function. Default time unit (tick), normally is 00060 1 ms. 00061 */ 00062 00063 GUI_TIMER_TIME GUI_X_GetTime(void) { 00064 return OS_TimeMS; 00065 } 00066 00067 void GUI_X_Delay(int ms) { 00068 int tEnd = OS_TimeMS + ms; 00069 while ((tEnd - OS_TimeMS) > 0); 00070 } 00071 00072 /********************************************************************* 00073 * 00074 * GUI_X_Init() 00075 * 00076 * Note: 00077 * GUI_X_Init() is called from GUI_Init is a possibility to init 00078 * some hardware which needs to be up and running before the GUI. 00079 * If not required, leave this routine blank. 00080 */ 00081 00082 void GUI_X_Init(void) {} 00083 00084 00085 /********************************************************************* 00086 * 00087 * GUI_X_ExecIdle 00088 * 00089 * Note: 00090 * Called if WM is in idle state 00091 */ 00092 00093 void GUI_X_ExecIdle(void) {} 00094 00095 /********************************************************************* 00096 * 00097 * Logging: OS dependent 00098 00099 Note: 00100 Logging is used in higher debug levels only. The typical target 00101 build does not use logging and does therefor not require any of 00102 the logging routines below. For a release build without logging 00103 the routines below may be eliminated to save some space. 00104 (If the linker is not function aware and eliminates unreferenced 00105 functions automatically) 00106 00107 */ 00108 00109 void GUI_X_Log (const char *s) { GUI_USE_PARA(s); } 00110 void GUI_X_Warn (const char *s) { GUI_USE_PARA(s); } 00111 void GUI_X_ErrorOut(const char *s) { GUI_USE_PARA(s); } 00112 00113 /********************************************************************* 00114 * 00115 * Multitasking: 00116 * 00117 * GUI_X_InitOS() 00118 * GUI_X_GetTaskId() 00119 * GUI_X_Lock() 00120 * GUI_X_Unlock() 00121 * 00122 * Note: 00123 * The following routines are required only if emWin is used in a 00124 * true multi task environment, which means you have more than one 00125 * thread using the emWin API. 00126 * In this case the 00127 * #define GUI_OS 1 00128 * needs to be in GUIConf.h 00129 */ 00130 00131 void GUI_X_InitOS(void) { } 00132 void GUI_X_Unlock(void) { } 00133 void GUI_X_Lock(void) { } 00134 U32 GUI_X_GetTaskId(void) { return 1; } 00135 00136 00137 /********************************************************************* 00138 * 00139 * Event driving (optional with multitasking) 00140 * 00141 * GUI_X_WaitEvent() 00142 * GUI_X_WaitEventTimed() 00143 * GUI_X_SignalEvent() 00144 */ 00145 00146 void GUI_X_WaitEvent(void) { } 00147 void GUI_X_SignalEvent(void) { } 00148 void GUI_X_WaitEventTimed(int Period) { } 00149 00150 /*************************** End of file ****************************/
Generated on Mon Mar 4 2024 07:48:13 by
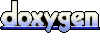