Update vom 10.05.15
Dependents: 19_Taster_BSW_oo 19_Taster_a
Fork of timer0 by
timer0.cpp
00001 00002 #include "timer0.h" 00003 00004 //-------------------------------------------------------- 00005 // Construktor initialisiert den Timer 00006 timer0::timer0() 00007 { 00008 uint8_t i; 00009 00010 // Initialize countdown timers 00011 for (i=0; i < TIMER0_NUM_COUNTDOWNTIMERS; i++) 00012 CountDownTimers[i].status = 0xFF; 00013 00014 ticker.attach_us(this, &timer0::func, 1000); 00015 00016 countMillisecond = 0; 00017 } 00018 00019 //-------------------------------------------------------- 00020 // Interruptroutine wird jede ms aufgerufen 00021 void timer0::func(void) 00022 { 00023 uint8_t i; 00024 00025 countMillisecond++; 00026 00027 if(counter != 0) counter--; 00028 00029 // ----- count down timers in ms ------------------------------------------------- 00030 00031 for (i=0; i<TIMER0_NUM_COUNTDOWNTIMERS; i++) 00032 { 00033 if (CountDownTimers[i].status == 1) 00034 { 00035 if (CountDownTimers[i].count_timer > 0) 00036 CountDownTimers[i].count_timer -- ; 00037 if (CountDownTimers[i].count_timer == 0) 00038 CountDownTimers[i].status = 0; 00039 } 00040 } 00041 00042 //--------------------------------------------------------- 00043 // count down timer für Sekunden 00044 00045 if (countMillisecond >= 1000) 00046 { 00047 countMillisecond = 0; // ----- count down timers in Sekunden ------------------------------------------------- 00048 00049 for (i=0; i<TIMER0_NUM_COUNTDOWNTIMERS; i++) 00050 { 00051 if (CountDownTimers[i].status == 2) 00052 { 00053 if (CountDownTimers[i].count_timer > 0) 00054 CountDownTimers[i].count_timer -- ; 00055 if (CountDownTimers[i].count_timer == 0) 00056 CountDownTimers[i].status = 0; 00057 } 00058 } 00059 } 00060 00061 } 00062 00063 //-------------------------------------------------------- 00064 // Abfrage nach freiem Timer 00065 // 00066 // wenn alle Timer belegt sind wird 0xFF zurückgegebne 00067 00068 uint8_t timer0::AllocateCountdownTimer (void) 00069 { 00070 uint8_t i; 00071 00072 for (i=0; i<TIMER0_NUM_COUNTDOWNTIMERS; i++) 00073 if (CountDownTimers[i].status == 0xFF) 00074 { 00075 CountDownTimers[i].status = 0x00; // Timer reserviert, nicht gestartet 00076 // printf_P(PSTR("\rallocate timer [%03d] %d\n"),i,ListPointer); 00077 00078 return i; 00079 } 00080 00081 return 0xFF; 00082 } 00083 00084 //-------------------------------------------------------- 00085 // Timer wieder freigeben 00086 void timer0::RemoveCountdownTimer(uint8_t timer) 00087 { 00088 CountDownTimers[timer].status = 0xFF; 00089 } 00090 00091 //-------------------------------------------------------- 00092 // Abfrage ob Timer 0 erreicht hat 00093 uint8_t timer0::GetTimerStatus(uint8_t timer) 00094 { 00095 return CountDownTimers[timer].status; 00096 } 00097 00098 //-------------------------------------------------------- 00099 // Abfrage der verbleibenden Zeit 00100 uint16_t timer0::GetTimerZeit(uint8_t timer) 00101 { 00102 return CountDownTimers[timer].count_timer; 00103 } 00104 00105 //-------------------------------------------------------- 00106 // Timer aktivieren 00107 void timer0::SetCountdownTimer(uint8_t timer, uint8_t status, uint16_t value) 00108 { 00109 CountDownTimers[timer].count_timer = value; 00110 CountDownTimers[timer].status = status; 00111 }
Generated on Mon Jul 18 2022 01:30:50 by
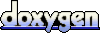