Nordic nrf51 sdk sources. Mirrored from https://github.com/ARMmbed/nrf51-sdk.
Embed:
(wiki syntax)
Show/hide line numbers
ble_srv_common.c
00001 /* 00002 * Copyright (c) Nordic Semiconductor ASA 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, this 00009 * list of conditions and the following disclaimer. 00010 * 00011 * 2. Redistributions in binary form must reproduce the above copyright notice, this 00012 * list of conditions and the following disclaimer in the documentation and/or 00013 * other materials provided with the distribution. 00014 * 00015 * 3. Neither the name of Nordic Semiconductor ASA nor the names of other 00016 * contributors to this software may be used to endorse or promote products 00017 * derived from this software without specific prior written permission. 00018 * 00019 * 00020 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00021 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00022 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00023 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR 00024 * ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00025 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00026 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON 00027 * ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00028 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00029 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00030 * 00031 */ 00032 00033 /* Attention! 00034 * To maintain compliance with Nordic Semiconductor ASA's Bluetooth profile 00035 * qualification listings, this section of source code must not be modified. 00036 */ 00037 00038 #include "ble_srv_common.h " 00039 #include <string.h> 00040 #include "nordic_common.h" 00041 #include "app_error.h " 00042 #include "nrf_ble.h" 00043 00044 uint8_t ble_srv_report_ref_encode(uint8_t * p_encoded_buffer, 00045 const ble_srv_report_ref_t * p_report_ref) 00046 { 00047 uint8_t len = 0; 00048 00049 p_encoded_buffer[len++] = p_report_ref->report_id; 00050 p_encoded_buffer[len++] = p_report_ref->report_type; 00051 00052 APP_ERROR_CHECK_BOOL(len == BLE_SRV_ENCODED_REPORT_REF_LEN); 00053 return len; 00054 } 00055 00056 00057 void ble_srv_ascii_to_utf8(ble_srv_utf8_str_t * p_utf8, char * p_ascii) 00058 { 00059 p_utf8->length = (uint16_t)strlen(p_ascii); 00060 p_utf8->p_str = (uint8_t *)p_ascii; 00061 } 00062 00063 00064 /**@brief Function for setting security requirements of a characteristic. 00065 * 00066 * @param[in] level required security level. 00067 * @param[out] p_perm Characteristic security requirements. 00068 * 00069 * @return encoded security level and security mode. 00070 */ 00071 static inline void set_security_req(security_req_t level, ble_gap_conn_sec_mode_t * p_perm) 00072 { 00073 00074 00075 BLE_GAP_CONN_SEC_MODE_SET_NO_ACCESS(p_perm); 00076 switch (level) 00077 { 00078 case SEC_NO_ACCESS: 00079 BLE_GAP_CONN_SEC_MODE_SET_NO_ACCESS(p_perm); 00080 break; 00081 case SEC_OPEN: 00082 BLE_GAP_CONN_SEC_MODE_SET_OPEN(p_perm); 00083 break; 00084 case SEC_JUST_WORKS: 00085 BLE_GAP_CONN_SEC_MODE_SET_ENC_NO_MITM(p_perm); 00086 break; 00087 case SEC_MITM: 00088 BLE_GAP_CONN_SEC_MODE_SET_ENC_WITH_MITM(p_perm); 00089 break; 00090 case SEC_SIGNED: 00091 BLE_GAP_CONN_SEC_MODE_SET_SIGNED_NO_MITM(p_perm); 00092 break; 00093 case SEC_SIGNED_MITM: 00094 BLE_GAP_CONN_SEC_MODE_SET_SIGNED_WITH_MITM(p_perm); 00095 break; 00096 } 00097 return; 00098 } 00099 00100 00101 uint32_t characteristic_add(uint16_t service_handle, 00102 ble_add_char_params_t * p_char_props, 00103 ble_gatts_char_handles_t * p_char_handle) 00104 { 00105 ble_gatts_char_md_t char_md; 00106 ble_gatts_attr_t attr_char_value; 00107 ble_uuid_t char_uuid; 00108 ble_gatts_attr_md_t attr_md; 00109 ble_gatts_attr_md_t user_descr_attr_md; 00110 ble_gatts_attr_md_t cccd_md; 00111 00112 if (p_char_props->uuid_type == 0) 00113 { 00114 char_uuid.type = BLE_UUID_TYPE_BLE; 00115 } 00116 else 00117 { 00118 char_uuid.type = p_char_props->uuid_type; 00119 } 00120 char_uuid.uuid = p_char_props->uuid; 00121 00122 memset(&attr_md, 0, sizeof(ble_gatts_attr_md_t)); 00123 set_security_req(p_char_props->read_access, &attr_md.read_perm); 00124 set_security_req(p_char_props->write_access, & attr_md.write_perm); 00125 attr_md.rd_auth = (p_char_props->is_defered_read ? 1 : 0); 00126 attr_md.wr_auth = (p_char_props->is_defered_write ? 1 : 0); 00127 attr_md.vlen = (p_char_props->is_var_len ? 1 : 0); 00128 attr_md.vloc = (p_char_props->is_value_user ? BLE_GATTS_VLOC_USER : BLE_GATTS_VLOC_STACK); 00129 00130 00131 memset(&char_md, 0, sizeof(ble_gatts_char_md_t)); 00132 if ((p_char_props->char_props.notify == 1)||(p_char_props->char_props.indicate == 1)) 00133 { 00134 00135 memset(&cccd_md, 0, sizeof(cccd_md)); 00136 set_security_req(p_char_props->cccd_write_access, &cccd_md.write_perm); 00137 BLE_GAP_CONN_SEC_MODE_SET_OPEN(&cccd_md.read_perm); 00138 00139 cccd_md.vloc = BLE_GATTS_VLOC_STACK; 00140 00141 char_md.p_cccd_md = &cccd_md; 00142 } 00143 char_md.char_props = p_char_props->char_props; 00144 00145 memset(&attr_char_value, 0, sizeof(ble_gatts_attr_t)); 00146 attr_char_value.p_uuid = &char_uuid; 00147 attr_char_value.p_attr_md = &attr_md; 00148 attr_char_value.max_len = p_char_props->max_len; 00149 if (p_char_props->p_init_value != NULL) 00150 { 00151 attr_char_value.init_len = p_char_props->init_len; 00152 attr_char_value.p_value = p_char_props->p_init_value; 00153 } 00154 if (p_char_props->p_user_descr != NULL) 00155 { 00156 memset(&user_descr_attr_md, 0, sizeof(ble_gatts_attr_md_t)); 00157 char_md.char_user_desc_max_size = p_char_props->p_user_descr->max_size; 00158 char_md.char_user_desc_size = p_char_props->p_user_descr->size; 00159 char_md.p_char_user_desc = p_char_props->p_user_descr->p_char_user_desc; 00160 00161 char_md.p_user_desc_md = &user_descr_attr_md; 00162 00163 set_security_req(p_char_props->p_user_descr->read_access, &user_descr_attr_md.read_perm); 00164 set_security_req(p_char_props->p_user_descr->write_access, &user_descr_attr_md.write_perm); 00165 00166 user_descr_attr_md.rd_auth = (p_char_props->p_user_descr->is_defered_read ? 1 : 0); 00167 user_descr_attr_md.wr_auth = (p_char_props->p_user_descr->is_defered_write ? 1 : 0); 00168 user_descr_attr_md.vlen = (p_char_props->p_user_descr->is_var_len ? 1 : 0); 00169 user_descr_attr_md.vloc = (p_char_props->p_user_descr->is_value_user ? BLE_GATTS_VLOC_USER : BLE_GATTS_VLOC_STACK); 00170 } 00171 if (p_char_props->p_presentation_format != NULL) 00172 { 00173 char_md.p_char_pf = p_char_props->p_presentation_format; 00174 } 00175 return sd_ble_gatts_characteristic_add(service_handle, 00176 &char_md, 00177 &attr_char_value, 00178 p_char_handle); 00179 } 00180 00181 00182 uint32_t descriptor_add(uint16_t char_handle, 00183 ble_add_descr_params_t * p_descr_props, 00184 uint16_t * p_descr_handle) 00185 { 00186 ble_gatts_attr_t descr_params; 00187 ble_uuid_t desc_uuid; 00188 ble_gatts_attr_md_t attr_md; 00189 00190 memset(&descr_params, 0, sizeof(descr_params)); 00191 if (p_descr_props->uuid_type == 0) 00192 { 00193 desc_uuid.type = BLE_UUID_TYPE_BLE; 00194 } 00195 else 00196 { 00197 desc_uuid.type = p_descr_props->uuid_type; 00198 } 00199 desc_uuid.uuid = p_descr_props->uuid; 00200 descr_params.p_uuid = &desc_uuid; 00201 00202 set_security_req(p_descr_props->read_access, &attr_md.read_perm); 00203 set_security_req(p_descr_props->write_access,&attr_md.write_perm); 00204 00205 attr_md.rd_auth = (p_descr_props->is_defered_read ? 1 : 0); 00206 attr_md.wr_auth = (p_descr_props->is_defered_write ? 1 : 0); 00207 attr_md.vlen = (p_descr_props->is_var_len ? 1 : 0); 00208 attr_md.vloc = (p_descr_props->is_value_user ? BLE_GATTS_VLOC_USER : BLE_GATTS_VLOC_STACK); 00209 descr_params.p_attr_md = &attr_md; 00210 00211 descr_params.init_len = p_descr_props->init_len; 00212 descr_params.init_offs = p_descr_props->init_offs; 00213 descr_params.max_len = p_descr_props->max_len; 00214 descr_params.p_value = p_descr_props->p_value; 00215 00216 return sd_ble_gatts_descriptor_add(char_handle, &descr_params, p_descr_handle); 00217 }
Generated on Tue Jul 12 2022 15:05:58 by
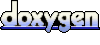