
Example Puck (BLE)
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /** 00002 * Copyright 2014 Nordic Semiconductor 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License 00015 */ 00016 00017 #define LOG_LEVEL_INFO 00018 00019 /** 00020 * Warning: remove LOG_LEVEL_* defines in production for major power savings. 00021 * 00022 * Defining a log level will set up a serial connection to use for logging. 00023 * This serial connection sets up a timer prohibiting the mbed chip 00024 * from entering low power states, drawing ~1.4mAh instead of ~20µAh with logging disabled. 00025 */ 00026 00027 #include "Puck.h" 00028 00029 Puck* puck = &Puck::getPuck(); 00030 00031 // Sample Gatt characteristic and service UUIDs 00032 const UUID SAMPLE_GATT_SERVICE = stringToUUID("bftj http post "); 00033 const UUID SAMPLE_GATT_CHARACTERISTIC = stringToUUID("bftj post body "); 00034 00035 int main(void) { 00036 // Add the Gatt characteristic 00037 int characteristicValueLength = 1; 00038 puck->addCharacteristic( 00039 SAMPLE_GATT_SERVICE, 00040 SAMPLE_GATT_CHARACTERISTIC, 00041 characteristicValueLength, 00042 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY); 00043 00044 // Initialize the puck 00045 puck->init(0xFEED); 00046 00047 // Set the initial value of the characteristic 00048 uint8_t new_value = 42; 00049 puck->updateCharacteristicValue(SAMPLE_GATT_CHARACTERISTIC, &new_value, characteristicValueLength); 00050 00051 // Let the puck do its thing 00052 while(puck->drive()); 00053 }
Generated on Wed Jul 13 2022 04:23:28 by
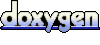