BLE-writeable Display Puck with an e-paper display. Built on the Puck IOT platform.
Dependencies: Puck lz mbed seeedstudio-epaper
main.cpp
00001 #include <mbed.h> 00002 #include "EPD.h" 00003 #include "lz.h" 00004 00005 // Remove the log level define in production. It will prevent your mbed from entering low power modes. 00006 #define LOG_LEVEL_ERROR 00007 #include "Puck.h" 00008 00009 int receiveIndex = 0; 00010 00011 Puck* puck = &Puck::getPuck(); 00012 00013 00014 EPD_Class EPD(p0, p2, p3, p8, p5, p6, p7); 00015 00016 00017 const UUID DISPLAY_SERVICE_UUID = stringToUUID("bftj display "); 00018 const UUID COMMAND_UUID = stringToUUID("bftj display com"); 00019 const UUID DATA_UUID = stringToUUID("bftj display dat"); 00020 00021 00022 /* 00023 00024 connections: 00025 00026 00027 LPC1768 <--> nRF51822 <--> Shield 00028 ---------||------------||-------- 00029 p12 <--> p2 <--> D2 (M_EPD_PANEL_ON) 00030 p13 <--> p3 <--> D3 (M_EPD_BORDER) 00031 p14 <--> p4 <--> D4 (M_/SD_CS) 00032 p25 <--> p5 <--> D5 (M_EPD_PWM) 00033 p16 <--> p6 <--> D6 (M_EPD_/RESET) 00034 p17 <--> p7 <--> D7 (M_EPD_BUSY) 00035 p18 <--> p8 <--> D8 (M_EPD_DISCHARGE) 00036 p19 <--> p26 <--> D9 (M_/WORD_STOCK_CS) 00037 p20 <--> p0 <--> D10 (M_/EPD_CS) 00038 p21 <--> p27 <--> A1 (M_OE123) 00039 p22 <--> p28 <--> A2 (M_CKV) 00040 p23 <--> p29 <--> A3 (M_STV_IN) 00041 00042 p5 <--> p20 <--> MOSI 00043 p6 <--> p22 <--> MISO 00044 p7 <--> p25 <--> SCK 00045 00046 VCC <--> VCC 00047 GND <--> GND 00048 00049 */ 00050 00051 #define COMMAND_NOOP 0 00052 #define COMMAND_CLEAR 1 00053 #define COMMAND_IMAGE_UPPER 2 00054 #define COMMAND_IMAGE_LOWER 3 00055 #define COMMAND_BEGIN_UPPER 4 00056 #define COMMAND_BEGIN_LOWER 5 00057 00058 #define IMAGE_SIZE 2904 00059 #define BUFFER_SIZE 2917 00060 #define Y_SIZE 88 00061 #define X_SIZE 264 00062 uint8_t buffer[BUFFER_SIZE]; 00063 00064 int currentCommand = COMMAND_NOOP; 00065 00066 00067 void reverseBufferSegment(int from, int to) { 00068 for(int i = from; i < (to + from + 1) / 2; i++) { 00069 int i2 = to + from - i - 1; 00070 int temp = buffer[i]; 00071 buffer[i] = buffer[i2]; 00072 buffer[i2] = temp; 00073 } 00074 LOG_DEBUG("Reversed buffer from index %i to index %i.\n", from, to); 00075 } 00076 00077 00078 void onCommandWritten(const uint8_t* value, uint8_t length) { 00079 currentCommand = value[0]; 00080 LOG_DEBUG("Received command: %i.\n", currentCommand); 00081 00082 switch(currentCommand) { 00083 00084 case COMMAND_BEGIN_UPPER: 00085 case COMMAND_BEGIN_LOWER: 00086 receiveIndex = BUFFER_SIZE; 00087 break; 00088 00089 case COMMAND_CLEAR: 00090 LOG_INFO("Clearing display.\n"); 00091 EPD.begin(EPD_2_7); 00092 EPD.start(); 00093 EPD.clear(); 00094 EPD.end(); 00095 break; 00096 00097 case COMMAND_IMAGE_UPPER: 00098 LOG_INFO("Writing image to top half of display.\n"); 00099 reverseBufferSegment(receiveIndex, BUFFER_SIZE); 00100 LOG_DEBUG("LZ_Uncompress(in: 0x%x, out 0x%x, insize: %i)\n", buffer + receiveIndex, buffer, BUFFER_SIZE - receiveIndex); 00101 LZ_Uncompress(buffer + receiveIndex, buffer, BUFFER_SIZE - receiveIndex); 00102 LOG_INFO("Uncompressed %i bytes of data.\n", BUFFER_SIZE - receiveIndex); 00103 EPD.begin(EPD_2_7); 00104 EPD.start(); 00105 EPD.image(buffer, 0, EPD.lines_per_display / 2); 00106 EPD.end(); 00107 break; 00108 00109 case COMMAND_IMAGE_LOWER: 00110 LOG_INFO("Writing image to bottom half of display.\n"); 00111 reverseBufferSegment(receiveIndex, BUFFER_SIZE); 00112 LZ_Uncompress(buffer + receiveIndex, buffer, BUFFER_SIZE - receiveIndex); 00113 LOG_INFO("Uncompressed %i bytes of data.\n", BUFFER_SIZE - receiveIndex); 00114 EPD.begin(EPD_2_7); 00115 EPD.start(); 00116 EPD.image(buffer, EPD.lines_per_display / 2, EPD.lines_per_display); 00117 EPD.end(); 00118 break; 00119 00120 } 00121 00122 currentCommand = COMMAND_NOOP; 00123 } 00124 00125 void onDataWritten(const uint8_t* value, uint8_t length) { 00126 LOG_VERBOSE("Data written, receiveIndex: %i\n", receiveIndex); 00127 for(int i = 0; i < length && receiveIndex > 0; i++) { 00128 buffer[--receiveIndex] = value[i]; 00129 } 00130 } 00131 00132 00133 int main() { 00134 LOG_INFO("Starting puck.\n"); 00135 00136 DigitalOut SD_CS(p4); 00137 DigitalOut WORD_STOCK_CS(p26); 00138 00139 SD_CS = 1; 00140 WORD_STOCK_CS = 1; 00141 00142 puck->addCharacteristic(DISPLAY_SERVICE_UUID, COMMAND_UUID, 1); 00143 puck->addCharacteristic(DISPLAY_SERVICE_UUID, DATA_UUID, 20); 00144 00145 00146 puck->onCharacteristicWrite(&COMMAND_UUID, onCommandWritten); 00147 puck->onCharacteristicWrite(&DATA_UUID, onDataWritten); 00148 00149 puck->init(0x5EED); 00150 00151 puck->countFreeMemory(); 00152 00153 currentCommand = COMMAND_NOOP; 00154 00155 while (puck->drive()); 00156 }
Generated on Tue Jul 12 2022 21:53:50 by
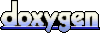