
RT1050 GUI demo using emWin library
Embed:
(wiki syntax)
Show/hide line numbers
MULTIPAGE_Private.h
00001 /********************************************************************* 00002 * SEGGER Microcontroller GmbH & Co. KG * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2016 SEGGER Microcontroller GmbH & Co. KG * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.38 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed to NXP Semiconductors USA, Inc. whose 00019 registered office is situated at 411 E. Plumeria Drive, San Jose, 00020 CA 95134, USA solely for the purposes of creating libraries for 00021 NXPs M0, M3/M4 and ARM7/9 processor-based devices, sublicensed and 00022 distributed under the terms and conditions of the NXP End User License 00023 Agreement. 00024 Full source code is available at: www.segger.com 00025 00026 We appreciate your understanding and fairness. 00027 ---------------------------------------------------------------------- 00028 Licensing information 00029 00030 Licensor: SEGGER Microcontroller Systems LLC 00031 Licensed to: NXP Semiconductors, 1109 McKay Dr, M/S 76, San Jose, CA 95131, USA 00032 Licensed SEGGER software: emWin 00033 License number: GUI-00186 00034 License model: emWin License Agreement, dated August 20th 2011 00035 Licensed product: - 00036 Licensed platform: NXP's ARM 7/9, Cortex-M0,M3,M4 00037 Licensed number of seats: - 00038 ---------------------------------------------------------------------- 00039 File : MULTIPAGE_Private.h 00040 Purpose : Private MULTIPAGE include 00041 --------------------END-OF-HEADER------------------------------------- 00042 */ 00043 00044 #ifndef MULTIPAGE_PRIVATE_H 00045 #define MULTIPAGE_PRIVATE_H 00046 00047 #include "GUI_Debug.h" 00048 #include "GUI_ARRAY.h" 00049 #include "MULTIPAGE.h" 00050 00051 #if GUI_WINSUPPORT 00052 00053 /********************************************************************* 00054 * 00055 * Defines 00056 * 00057 ********************************************************************** 00058 */ 00059 #define MULTIPAGE_STATE_ENABLED (1 << 0) 00060 #define MULTIPAGE_STATE_SCROLLMODE WIDGET_STATE_USER0 00061 00062 #define MULTIPAGE_NUMCOLORS 2 00063 00064 /********************************************************************* 00065 * 00066 * Object definition 00067 * 00068 ********************************************************************** 00069 */ 00070 // 00071 // MULTIPAGE_PAGE 00072 // 00073 typedef struct { 00074 WM_HWIN hWin; 00075 U8 Status; 00076 int ItemWidth; 00077 WM_HMEM hDrawObj[3]; 00078 char acText; 00079 } MULTIPAGE_PAGE; 00080 00081 // 00082 // MULTIPAGE_SKIN_PRIVATE 00083 // 00084 typedef struct { 00085 WIDGET_DRAW_ITEM_FUNC * pfDrawSkin; 00086 } MULTIPAGE_SKIN_PRIVATE; 00087 00088 // 00089 // MULTIPAGE_PROPS 00090 // 00091 typedef struct { 00092 const GUI_FONT * pFont; 00093 unsigned Align; 00094 GUI_COLOR aBkColor[MULTIPAGE_NUMCOLORS]; 00095 GUI_COLOR aTextColor[MULTIPAGE_NUMCOLORS]; 00096 MULTIPAGE_SKIN_PRIVATE SkinPrivate; 00097 int BorderSize0; 00098 int BorderSize1; 00099 unsigned TextAlign; 00100 unsigned Scrollbar; 00101 int (* pfGetTouchedPage)(MULTIPAGE_Handle hObj, int x, int y); 00102 int (* pfGetTabBarWidth)(MULTIPAGE_Handle hObj); 00103 } MULTIPAGE_PROPS; 00104 00105 // 00106 // MULTIPAGE_Obj 00107 // 00108 typedef struct MULTIPAGE_Obj MULTIPAGE_Obj; 00109 00110 struct MULTIPAGE_Obj { 00111 WIDGET Widget; 00112 void (* pfDrawTextItem)(MULTIPAGE_Obj * pObj, const char * pText, unsigned Index, const GUI_RECT * pRect, int x0, int xSize, int ColorIndex); 00113 WM_HWIN hClient; 00114 GUI_ARRAY hPageArray; 00115 unsigned Selection; 00116 int ScrollState; 00117 MULTIPAGE_PROPS Props; 00118 WIDGET_SKIN const * pWidgetSkin; 00119 MULTIPAGE_SKIN_PROPS SkinProps; 00120 int ItemHeight; 00121 int MaxHeight; 00122 }; 00123 00124 /********************************************************************* 00125 * 00126 * Macros for internal use 00127 * 00128 ********************************************************************** 00129 */ 00130 #if GUI_DEBUG_LEVEL >= GUI_DEBUG_LEVEL_CHECK_ALL 00131 #define MULTIPAGE_INIT_ID(p) (p->Widget.DebugId = MULTIPAGE_ID) 00132 #else 00133 #define MULTIPAGE_INIT_ID(p) 00134 #endif 00135 00136 #if GUI_DEBUG_LEVEL >= GUI_DEBUG_LEVEL_CHECK_ALL 00137 MULTIPAGE_Obj * MULTIPAGE_LockH(MULTIPAGE_Handle h); 00138 #define MULTIPAGE_LOCK_H(h) MULTIPAGE_LockH(h) 00139 #else 00140 #define MULTIPAGE_LOCK_H(h) (MULTIPAGE_Obj *)GUI_LOCK_H(h) 00141 #endif 00142 00143 /********************************************************************* 00144 * 00145 * Externals 00146 * 00147 ********************************************************************** 00148 */ 00149 extern GUI_COLOR MULTIPAGE__aEffectColor[2]; 00150 extern MULTIPAGE_PROPS MULTIPAGE__DefaultProps; 00151 00152 extern const WIDGET_SKIN MULTIPAGE__SkinClassic; 00153 extern WIDGET_SKIN MULTIPAGE__Skin; 00154 00155 extern WIDGET_SKIN const * MULTIPAGE__pSkinDefault; 00156 00157 /********************************************************************* 00158 * 00159 * Private functions 00160 * 00161 ********************************************************************** 00162 */ 00163 void MULTIPAGE__CalcBorderRect (MULTIPAGE_Obj * pObj, GUI_RECT * pRect); 00164 void MULTIPAGE__CalcClientRect (MULTIPAGE_Handle hObj, GUI_RECT * pRect); 00165 void MULTIPAGE__DeleteScrollbar(MULTIPAGE_Handle hObj); 00166 void MULTIPAGE__DrawTextItemH (MULTIPAGE_Obj * pObj, const char * pText, unsigned Index, const GUI_RECT * pRect, int x0, int w, int ColorIndex); 00167 int MULTIPAGE__GetPagePos (MULTIPAGE_Handle hObj, unsigned Index); 00168 int MULTIPAGE__GetPageWidth (MULTIPAGE_Handle hObj, unsigned Index); 00169 void MULTIPAGE__GetTabBarRect (MULTIPAGE_Handle hObj, GUI_RECT * pRect); 00170 int MULTIPAGE__SetDrawObj (MULTIPAGE_Handle hObj, GUI_DRAW_HANDLE hDrawObj, int Index, int State); 00171 void MULTIPAGE__UpdatePositions(MULTIPAGE_Handle hObj); 00172 00173 /********************************************************************* 00174 * 00175 * Private Skinning functions 00176 * 00177 ********************************************************************** 00178 */ 00179 int MULTIPAGE_SKIN__GetPagePos (MULTIPAGE_Handle hObj, unsigned Index); 00180 int MULTIPAGE_SKIN__GetTabBarWidth(MULTIPAGE_Handle hObj); 00181 int MULTIPAGE_SKIN__GetTouchedPage(MULTIPAGE_Handle hObj, int TouchX, int TouchY); 00182 00183 #endif // GUI_WINSUPPORT 00184 #endif // MULTIPAGE_PRIVATE_H 00185 00186 /*************************** End of file ****************************/
Generated on Tue Jul 12 2022 19:43:37 by
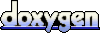