
RT1050 GUI demo using emWin library
Embed:
(wiki syntax)
Show/hide line numbers
GUI_JPEG_Private.h
00001 /********************************************************************* 00002 * SEGGER Microcontroller GmbH & Co. KG * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2016 SEGGER Microcontroller GmbH & Co. KG * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.38 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed to NXP Semiconductors USA, Inc. whose 00019 registered office is situated at 411 E. Plumeria Drive, San Jose, 00020 CA 95134, USA solely for the purposes of creating libraries for 00021 NXPs M0, M3/M4 and ARM7/9 processor-based devices, sublicensed and 00022 distributed under the terms and conditions of the NXP End User License 00023 Agreement. 00024 Full source code is available at: www.segger.com 00025 00026 We appreciate your understanding and fairness. 00027 ---------------------------------------------------------------------- 00028 Licensing information 00029 00030 Licensor: SEGGER Microcontroller Systems LLC 00031 Licensed to: NXP Semiconductors, 1109 McKay Dr, M/S 76, San Jose, CA 95131, USA 00032 Licensed SEGGER software: emWin 00033 License number: GUI-00186 00034 License model: emWin License Agreement, dated August 20th 2011 00035 Licensed product: - 00036 Licensed platform: NXP's ARM 7/9, Cortex-M0,M3,M4 00037 Licensed number of seats: - 00038 ---------------------------------------------------------------------- 00039 File : GUI_JPEG_Private.h 00040 Purpose : Private header 00041 ---------------------------------------------------------------------- 00042 00043 Explanation of terms: 00044 00045 Component - Color channel, e.g., Red or Luminance. 00046 Sample - Single component value (i.e., one number in the image data). 00047 Coefficient - Frequency coefficient (a DCT transform output number). 00048 Block - 8x8 group of samples or coefficients. 00049 MCU - (Minimum Coded Unit) is an interleaved set of blocks of size 00050 determined by the sampling factors, or a single block in a 00051 noninterleaved scan. 00052 00053 ---------------------------END-OF-HEADER------------------------------ 00054 */ 00055 00056 #ifndef GUI_JPEG_PRIVATE_H 00057 #define GUI_JPEG_PRIVATE_H 00058 00059 /********************************************************************* 00060 * 00061 * Defines 00062 * 00063 ********************************************************************** 00064 */ 00065 #define JPEG_LOCK_H(h) (GUI_JPEG_DCONTEXT *)GUI_LOCK_H(h) 00066 00067 /********************************************************************* 00068 * 00069 * Scan types 00070 */ 00071 #define GRAYSCALE 0 00072 #define YH1V1 1 00073 #define YH2V1 2 00074 #define YH1V2 3 00075 #define YH2V2 4 00076 00077 /********************************************************************* 00078 * 00079 * Maximum number of... 00080 */ 00081 #define MAX_COMPONENTS 4 00082 #define MAX_HUFFTABLES 8 00083 #define MAX_QUANTTABLES 4 00084 #define MAX_COMPSINSCAN 4 00085 #define MAX_BLOCKSPERMCU 10 00086 00087 /********************************************************************* 00088 * 00089 * Marker definitions 00090 */ 00091 #define M_SOF0 0xc0 // Start Of Frame 00092 #define M_SOF1 0xc1 00093 #define M_SOF2 0xc2 00094 #define M_SOF3 0xc3 00095 00096 #define M_SOF5 0xc5 00097 #define M_SOF6 0xc6 00098 #define M_SOF7 0xc7 00099 00100 #define M_JPG 0xc8 00101 #define M_SOF9 0xc9 00102 #define M_SOF10 0xca 00103 #define M_SOF11 0xcb 00104 00105 #define M_SOF13 0xcd 00106 #define M_SOF14 0xce 00107 #define M_SOF15 0xcf 00108 00109 #define M_DHT 0xc4 // Define Huffman Table 00110 00111 #define M_DAC 0xcc // Define Arithmetic Coding Table 00112 00113 #define M_RST0 0xd0 00114 #define M_RST1 0xd1 00115 #define M_RST2 0xd2 00116 #define M_RST3 0xd3 00117 #define M_RST4 0xd4 00118 #define M_RST5 0xd5 00119 #define M_RST6 0xd6 00120 #define M_RST7 0xd7 00121 00122 #define M_SOI 0xd8 // Start Of Image 00123 #define M_EOI 0xd9 00124 #define M_SOS 0xda // Start Of Scan 00125 #define M_DQT 0xdb // Define Quantisation Table 00126 #define M_DRI 0xdd // Define Restart Interval 00127 00128 #define M_APP0 0xe0 // Application Usage 00129 #define M_APP14 0xee // Adobe marker 00130 00131 #define M_TEM 0x01 00132 00133 #define ADOBE_TRANSFORM_RGB 0 00134 #define ADOBE_TRANSFORM_YCBCR 1 00135 00136 /********************************************************************* 00137 * 00138 * Types 00139 * 00140 ********************************************************************** 00141 */ 00142 // 00143 // Default parameter structure for reading data from memory 00144 // 00145 typedef struct { 00146 const U8 * pFileData; 00147 I32 FileSize; 00148 } GUI_JPEG_PARAM; 00149 00150 // 00151 // Huffman table definition 00152 // 00153 typedef struct { 00154 unsigned aLookUp[256]; 00155 U8 aCodeSize[256]; 00156 unsigned aTree[512]; 00157 } HUFF_TABLE; 00158 00159 // 00160 // Coefficient buffer used for progressive JPEGs 00161 // 00162 typedef struct { 00163 int NumBlocksX; 00164 int NumBlocksY; 00165 unsigned BlockSize; 00166 GUI_HMEM hData; 00167 } COEFF_BUFFER; 00168 00169 typedef struct GUI_JPEG_DCONTEXT GUI_JPEG_DCONTEXT; 00170 00171 struct GUI_JPEG_DCONTEXT { 00172 // 00173 // Function pointer for reading one byte 00174 // 00175 int (* pfGetU8)(GUI_JPEG_DCONTEXT * pContext, U8 * pByte); 00176 00177 GUI_GET_DATA_FUNC * pfGetData; // 'GetData' Function pointer 00178 void * pParam; // Pointer passed to 'GetData' function 00179 U32 Off; // Data pointer 00180 // 00181 // Image size 00182 // 00183 U16 xSize; 00184 U16 ySize; 00185 // 00186 // Input buffer 00187 // 00188 const U8 * pBuffer; 00189 unsigned NumBytesInBuffer; 00190 U8 StartOfFile; 00191 U8 aStuff[4]; // Stuff back buffer 00192 U8 NumBytesStuffed; // Number of stuffed bytes 00193 // 00194 // Bit buffer 00195 // 00196 U32 BitBuffer; 00197 int NumBitsLeft; 00198 // 00199 // Huffman tables 00200 // 00201 U8 aHuffNumTableAvail[MAX_HUFFTABLES]; 00202 U8 aaHuffNum[MAX_HUFFTABLES][17]; // Pointer to number of Huffman codes per bit size 00203 U8 aaHuffVal[MAX_HUFFTABLES][256]; // Pointer to Huffman codes 00204 HUFF_TABLE aHuffTable[MAX_HUFFTABLES]; 00205 HUFF_TABLE * apDC_Huff[MAX_BLOCKSPERMCU]; 00206 HUFF_TABLE * apAC_Huff[MAX_BLOCKSPERMCU]; 00207 // 00208 // Quantization tables 00209 // 00210 U16 aaQuantTbl[MAX_QUANTTABLES][64]; 00211 U16 * apQuantTbl[MAX_QUANTTABLES]; 00212 // 00213 // Component information 00214 // 00215 U8 NumCompsPerFrame; // Number of components per frame 00216 U8 aCompHSamp[MAX_COMPONENTS]; // Component's horizontal sampling factor 00217 U8 aCompVSamp[MAX_COMPONENTS]; // Component's vertical sampling factor 00218 U8 aCompQuant[MAX_COMPONENTS]; // Component's quantization table selector 00219 U8 aCompId [MAX_COMPONENTS]; // Component's ID 00220 U8 NumCompsPerScan; // Number of components per scan 00221 U8 aCompList[MAX_COMPSINSCAN]; // Components in this scan 00222 U8 aCompDC_Tab[MAX_COMPONENTS]; // Component's DC Huffman coding table selector 00223 U8 aCompAC_Tab[MAX_COMPONENTS]; // Component's AC Huffman coding table selector 00224 unsigned * apComponent[MAX_BLOCKSPERMCU]; // Points into the table aLastDC_Val[] 00225 unsigned aLastDC_Val[MAX_COMPONENTS]; // Table of last DC values 00226 // 00227 // Data used for progressive scans 00228 // 00229 U8 SpectralStart; // Spectral selection start 00230 U8 SpectralEnd; // Spectral selection end 00231 U8 SuccessiveLow; // Successive approximation low 00232 U8 SuccessiveHigh; // Successive approximation high 00233 COEFF_BUFFER aDC_Coeffs[MAX_COMPONENTS]; // DC coefficient buffer for progressive scan 00234 COEFF_BUFFER aAC_Coeffs[MAX_COMPONENTS]; // AC coefficient buffer for progressive scan 00235 int aBlockY_MCU[MAX_COMPONENTS]; // 00236 // 00237 // Common 00238 // 00239 U8 TransformationRequired; 00240 U8 IsProgressive; // Flag is set to 1 if JPEG is progressive 00241 U8 ScanType; // Gray, Yh1v1, Yh1v2, Yh2v1, Yh2v2 00242 int MaxMCUsPerRow; // Maximum number of MCUs per row 00243 int MaxMCUsPerCol; // Maximum number of MCUs per column 00244 int MaxBlocksPerMCU; // Maximum number of blocks per MCU 00245 int MaxBlocksPerRow; // Maximum number of blocks per row 00246 int MaxMCU_xSize; // MCU's max. X size in pixels 00247 int MaxMCU_ySize; // MCU's max. Y size in pixels 00248 int DestBytesPerPixel; // 4 (RGB) or 1 (Y) 00249 int DestBytesPerScanline; // Rounded up 00250 int RealDestBytesPerScanline; // Actual bytes 00251 int EOB_Run; // 'End Of Band' run 00252 int RestartInterval; 00253 int RestartsLeft; 00254 int NextRestartNum; 00255 int MCUsPerRow; 00256 int MCUsPerCol; 00257 int NumBlocksPerMCU; 00258 int aMCU_Org[MAX_BLOCKSPERMCU]; 00259 // 00260 // Block buffer 00261 // 00262 GUI_HMEM hBlocks; 00263 GUI_HMEM hBlockMaxZagSet; 00264 // 00265 // Sample buffer 00266 // 00267 GUI_HMEM hSampleBuf; 00268 U8 * pSampleBuf; 00269 // 00270 // Status 00271 // 00272 int TotalLinesLeft; // Total number of lines left in image 00273 int MCULinesLeft; // Total number of lines left in current MCU 00274 // 00275 // Output buffer(s) 00276 // 00277 GUI_HMEM hScanLine0; // Buffer 0 00278 GUI_HMEM hScanLine1; // Buffer 1, only used for V2 sampling factors 00279 U8 BufferIndex; 00280 // 00281 // Arrays used for converting YCbCr to RGB 00282 // 00283 int aCRR[256]; 00284 int aCBB[256]; 00285 I32 aCRG[256]; 00286 I32 aCBG[256]; 00287 // 00288 // Banding 00289 // 00290 U8 BandingRequired; // Flag if banding is required 00291 unsigned NumBands; // Number of required bands for drawing the complete frame 00292 int NumBlocksPerBand; // Number of vertical blocks per band 00293 int FirstBlockOfBand; 00294 int aFirstBlockOfBand[MAX_COMPONENTS]; 00295 }; 00296 00297 /********************************************************************* 00298 * 00299 * Private interface 00300 * 00301 ********************************************************************** 00302 */ 00303 int GUI_JPEG__AllocBandingCoeffBuffer (GUI_HMEM hContext); 00304 int GUI_JPEG__DecodeLine (GUI_JPEG_DCONTEXT * pContext); 00305 int GUI_JPEG__DecodeProgressiveBanding(GUI_JPEG_DCONTEXT * pContext); 00306 void GUI_JPEG__Free (GUI_JPEG_DCONTEXT * pContext); 00307 GUI_COLOR GUI_JPEG__GetColorGray (const U8 ** ppData, unsigned SkipCnt); 00308 GUI_COLOR GUI_JPEG__GetColorRGB (const U8 ** ppData, unsigned SkipCnt); 00309 int GUI_JPEG__GetData (void * p, const U8 ** ppData, unsigned NumBytesReq, U32 Off); 00310 int GUI_JPEG__InitDraw (GUI_HMEM hContext); 00311 int GUI_JPEG__ReadUntilSOF (GUI_HMEM hContext); 00312 void GUI_JPEG__SetNextBand (GUI_JPEG_DCONTEXT * pContext); 00313 int GUI_JPEG__SkipLine (GUI_JPEG_DCONTEXT * pContext); 00314 int GUI_JPEG__GetInfoEx (GUI_HMEM hContext, GUI_JPEG_INFO * pInfo); 00315 00316 #endif 00317 00318 /*************************** End of file ****************************/
Generated on Tue Jul 12 2022 19:43:36 by
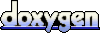